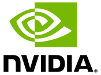 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CUDA_POINT_CLOUD_H__
24 #define __CUDA_POINT_CLOUD_H__
83 bool Reserve( uint32_t maxPoints );
98 bool Extract(
float* depth, float4* rgba, uint32_t width, uint32_t height );
103 bool Extract(
float* depth, uint32_t depth_width, uint32_t depth_height,
104 float4* rgba, uint32_t color_width, uint32_t color_height );
149 bool Save(
const char* filename );
164 void SetCalibration(
const float2& focalLength,
const float2& principalPoint );
bool mHasNewPoints
Definition: cudaPointCloud.h:188
Point vertex.
Definition: cudaPointCloud.h:45
size_t GetMaxSize() const
Retrieve the maximum size in bytes of the point cloud.
Definition: cudaPointCloud.h:124
uchar3 color
The RGB color of the point.
Definition: cudaPointCloud.h:56
Vertex * mPointsGPU
Definition: cudaPointCloud.h:173
uint32_t GetNumPoints() const
Retrieve the number of points being used.
Definition: cudaPointCloud.h:109
uint32_t mNumPoints
Definition: cudaPointCloud.h:178
uint32_t mMaxPoints
Definition: cudaPointCloud.h:179
CUDA-accelerated point cloud processing.
Definition: cudaPointCloud.h:39
uint32_t GetMaxPoints() const
Retrieve the max number of points in memory.
Definition: cudaPointCloud.h:114
bool allocDepthResize(size_t size)
uint8_t classID
The class ID of the point.
Definition: cudaPointCloud.h:62
bool SetCalibration(const char *filename)
Set the intrinsic camera calibration.
bool Save(const char *filename)
Save point cloud to PCD file.
static cudaPointCloud * Create()
Create.
bool Render()
Render the point cloud with OpenGL.
Vertex * mPointsCPU
Definition: cudaPointCloud.h:172
void Clear()
Clear the points, but keep the memory allocated.
~cudaPointCloud()
Destructor.
float * mDepthResize
Definition: cudaPointCloud.h:184
bool mHasRGB
Definition: cudaPointCloud.h:187
Vertex * GetData() const
Retrieve memory pointer to point cloud data.
Definition: cudaPointCloud.h:129
Vertex * GetData(size_t index) const
Retrieve memory pointer to a specific point.
Definition: cudaPointCloud.h:134
OpenGL perspective camera supporting Look-At, Yaw/Pitch/Roll, and Ortho modes.
Definition: glCamera.h:35
void Free()
Free the memory being used to store the point cloud.
float2 mPrincipalPoint
Definition: cudaPointCloud.h:182
float2 mFocalLength
Definition: cudaPointCloud.h:181
glCamera * mCameraGL
Definition: cudaPointCloud.h:176
glBuffer * mBufferGL
Definition: cudaPointCloud.h:175
OpenGL buffer with CUDA interoperability.
Definition: glBuffer.h:114
float3 pos
The XYZ position of the point.
Definition: cudaPointCloud.h:50
size_t mDepthSize
Definition: cudaPointCloud.h:185
struct cudaPointCloud::Vertex __attribute__((packed))
bool Reserve(uint32_t maxPoints)
Allocate and reserve memory for the max number of points.
bool HasRGB() const
Does the point cloud have RGB data?
Definition: cudaPointCloud.h:139
bool Extract(float *depth, float4 *rgba, uint32_t width, uint32_t height)
Extract point cloud from depth map and optional RGBA image.
size_t GetSize() const
Retrieve the size in bytes currently being used.
Definition: cudaPointCloud.h:119
bool mHasCalibration
Definition: cudaPointCloud.h:189