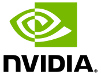 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __SEGMENTATION_NET_H__
24 #define __SEGMENTATION_NET_H__
34 #define SEGNET_DEFAULT_INPUT "input_0"
40 #define SEGNET_DEFAULT_OUTPUT "output_0"
46 #define SEGNET_DEFAULT_ALPHA 150
52 #define SEGNET_MODEL_TYPE "segmentation"
58 #define SEGNET_USAGE_STRING "segNet arguments: \n" \
59 " --network=NETWORK pre-trained model to load, one of the following:\n" \
60 " * fcn-resnet18-cityscapes-512x256\n" \
61 " * fcn-resnet18-cityscapes-1024x512\n" \
62 " * fcn-resnet18-cityscapes-2048x1024\n" \
63 " * fcn-resnet18-deepscene-576x320\n" \
64 " * fcn-resnet18-deepscene-864x480\n" \
65 " * fcn-resnet18-mhp-512x320\n" \
66 " * fcn-resnet18-mhp-640x360\n" \
67 " * fcn-resnet18-voc-320x320 (default)\n" \
68 " * fcn-resnet18-voc-512x320\n" \
69 " * fcn-resnet18-sun-512x400\n" \
70 " * fcn-resnet18-sun-640x512\n" \
71 " --model=MODEL path to custom model to load (caffemodel, uff, or onnx)\n" \
72 " --prototxt=PROTOTXT path to custom prototxt to load (for .caffemodel only)\n" \
73 " --labels=LABELS path to text file containing the labels for each class\n" \
74 " --colors=COLORS path to text file containing the colors for each class\n" \
75 " --input-blob=INPUT name of the input layer (default: '" SEGNET_DEFAULT_INPUT "')\n" \
76 " --output-blob=OUTPUT name of the output layer (default: '" SEGNET_DEFAULT_OUTPUT "')\n" \
77 " --alpha=ALPHA overlay alpha blending value, range 0-255 (default: 150)\n" \
78 " --visualize=VISUAL visualization flags (e.g. --visualize=overlay,mask)\n" \
79 " valid combinations are: 'overlay', 'mask'\n" \
80 " --profile enable layer profiling in TensorRT\n\n"
138 static segNet*
Create(
const char* prototxt_path,
const char* model_path,
139 const char* class_labels,
const char* class_colors=NULL,
175 template<
typename T>
bool Process( T* input, uint32_t width, uint32_t height,
const char* ignore_class=
"void" ) {
return Process((
void*)input, width, height, imageFormatFromType<T>(), ignore_class); }
185 bool Process(
void* input, uint32_t width, uint32_t height,
imageFormat format,
const char* ignore_class=
"void" );
196 bool Process(
float* input, uint32_t width, uint32_t height,
const char* ignore_class=
"void" );
201 template<
typename T>
bool Mask( T* output, uint32_t width, uint32_t height,
FilterMode filter=
FILTER_LINEAR ) {
return Mask((
void*)output, width, height, imageFormatFromType<T>(), filter); }
218 bool Mask( uint8_t* output, uint32_t width, uint32_t height );
229 template<
typename T>
bool Overlay( T* output, uint32_t width, uint32_t height,
FilterMode filter=
FILTER_LINEAR ) {
return Overlay((
void*)output, width, height, imageFormatFromType<T>(), filter); }
288 void SetClassColor( uint32_t classIndex,
float r,
float g,
float b,
float a=255.0f );
321 bool classify(
const char* ignore_class );
323 bool overlayPoint(
void* input, uint32_t in_width, uint32_t in_height,
imageFormat in_format,
void* output, uint32_t out_width, uint32_t out_height,
imageFormat out_format,
bool mask_only );
324 bool overlayLinear(
void* input, uint32_t in_width, uint32_t in_height,
imageFormat in_format,
void* output, uint32_t out_width, uint32_t out_height,
imageFormat out_format,
bool mask_only );
@ FILTER_LINEAR
Bilinear filtering.
Definition: segNet.h:96
bool Overlay(T *output, uint32_t width, uint32_t height, FilterMode filter=FILTER_LINEAR)
Produce the segmentation overlay alpha blended on top of the original image.
Definition: segNet.h:229
virtual ~segNet()
Destroy.
bool saveClassLegend(const char *filename)
uchar3 color
The RGB color of the point.
Definition: cudaPointCloud.h:11
uint32_t GetGridHeight() const
Retrieve the number of rows in the classification grid.
Definition: segNet.h:316
static uint32_t VisualizationFlagsFromStr(const char *str, uint32_t default_value=VISUALIZE_OVERLAY)
Parse a string of one of more VisualizationMode values.
void * mLastInputImg
last input image to be processed, stored for overlay
Definition: segNet.h:337
const char * GetClassLabel(uint32_t id) const
Retrieve the description of a particular class.
Definition: segNet.h:268
int FindClassID(const char *label_name)
Find the ID of a particular class (by label name).
imageFormat mLastInputFormat
pixel format of last input image
Definition: segNet.h:340
const char * GetClassPath() const
Retrieve the path to the file containing the class label descriptions.
Definition: segNet.h:304
bool overlayPoint(void *input, uint32_t in_width, uint32_t in_height, imageFormat in_format, void *output, uint32_t out_width, uint32_t out_height, imageFormat out_format, bool mask_only)
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
#define DIMS_C(x)
Definition: tensorNet.h:60
static FilterMode FilterModeFromStr(const char *str, FilterMode default_value=FILTER_LINEAR)
Parse a string from one of the FilterMode values.
#define SEGNET_DEFAULT_INPUT
Name of default input blob for segmentation model.
Definition: segNet.h:34
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
FilterMode
Enumeration of mask/overlay filtering modes.
Definition: segNet.h:93
float4 GetClassColor(uint32_t id) const
Retrieve the RGBA visualization color a particular class.
Definition: segNet.h:278
void SetClassColor(uint32_t classIndex, const float4 &color)
Set the visualization color of a particular class of object.
#define DIMS_H(x)
Definition: tensorNet.h:61
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
bool * mColorsAlphaSet
true if class color had been explicitly set from file or user
Definition: segNet.h:333
bool loadClassLabels(const char *filename)
static segNet * Create(const char *network="fcn-resnet18-voc", uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load a pre-trained model.
bool overlayLinear(void *input, uint32_t in_width, uint32_t in_height, imageFormat in_format, void *output, uint32_t out_width, uint32_t out_height, imageFormat out_format, bool mask_only)
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
bool Process(T *input, uint32_t width, uint32_t height, const char *ignore_class="void")
Perform the initial inferencing processing portion of the segmentation.
Definition: segNet.h:175
Image segmentation with FCN-Alexnet or custom models, using TensorRT.
Definition: segNet.h:87
@ FILTER_POINT
Nearest point sampling.
Definition: segNet.h:95
@ VISUALIZE_MASK
View just the colorized segmentation class mask.
Definition: segNet.h:105
#define SEGNET_USAGE_STRING
Standard command-line options able to be passed to segNet::Create()
Definition: segNet.h:58
uint8_t * mClassMap
runtime buffer for the argmax-classified class index of each tile
Definition: segNet.h:335
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
bool Mask(T *output, uint32_t width, uint32_t height, FilterMode filter=FILTER_LINEAR)
Produce a colorized segmentation mask.
Definition: segNet.h:201
void SetOverlayAlpha(float alpha, bool explicit_exempt=true)
Set overlay alpha blending value for all classes (between 0-255), (optionally except for those that h...
float GetOverlayAlpha() const
Retrieve the overlay alpha blending value for classes that don't have it explicitly set.
#define DIMS_W(x)
Definition: tensorNet.h:62
#define SEGNET_DEFAULT_OUTPUT
Name of default output blob for segmentation model.
Definition: segNet.h:40
VisualizationFlags
Visualization flags.
Definition: segNet.h:102
static const char * Usage()
Usage string for command line arguments to Create()
Definition: segNet.h:160
std::vector< std::string > mClassLabels
Definition: segNet.h:330
const char * GetClassDesc(uint32_t id) const
Retrieve the description of a particular class.
Definition: segNet.h:273
uint32_t GetGridWidth() const
Retrieve the number of columns in the classification grid.
Definition: segNet.h:310
float4 * mClassColors
array of overlay colors in shared CPU/GPU memory
Definition: segNet.h:334
@ VISUALIZE_OVERLAY
Overlay the segmentation class colors with alpha blending.
Definition: segNet.h:104
uint32_t mLastInputWidth
width in pixels of last input image to be processed
Definition: segNet.h:338
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
bool classify(const char *ignore_class)
uint32_t mLastInputHeight
height in pixels of last input image to be processed
Definition: segNet.h:339
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
bool loadClassColors(const char *filename)
std::string mClassPath
Definition: segNet.h:331
uint32_t GetNumClasses() const
Retrieve the number of object classes supported in the detector.
Definition: segNet.h:263
std::vector< layerInfo > mOutputs
Definition: tensorNet.h:819
__device__ cudaVectorTypeInfo< T >::Base alpha(T vec, typename cudaVectorTypeInfo< T >::Base default_alpha=255)
Definition: cudaVector.h:98