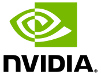 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __POSE_NET_H__
24 #define __POSE_NET_H__
37 #define POSENET_DEFAULT_INPUT "input"
43 #define POSENET_DEFAULT_CMAP "cmap"
49 #define POSENET_DEFAULT_PAF "paf"
55 #define POSENET_DEFAULT_THRESHOLD 0.15f
62 #define POSENET_DEFAULT_KEYPOINT_SCALE 0.0052f
69 #define POSENET_DEFAULT_LINK_SCALE 0.0013f
75 #define POSENET_MODEL_TYPE "pose"
81 #define POSENET_USAGE_STRING "poseNet arguments: \n" \
82 " --network=NETWORK pre-trained model to load, one of the following:\n" \
83 " * resnet18-body (default)\n" \
84 " * resnet18-hand\n" \
85 " * densenet121-body\n" \
86 " --model=MODEL path to custom model to load (caffemodel, uff, or onnx)\n" \
87 " --prototxt=PROTOTXT path to custom prototxt to load (for .caffemodel only)\n" \
88 " --labels=LABELS path to text file containing the labels for each class\n" \
89 " --input-blob=INPUT name of the input layer (default is '" POSENET_DEFAULT_INPUT "')\n" \
90 " --output-cvg=COVERAGE name of the coverge output layer (default is '" POSENET_DEFAULT_CMAP "')\n" \
91 " --output-bbox=BOXES name of the bounding output layer (default is '" POSENET_DEFAULT_PAF "')\n" \
92 " --mean-pixel=PIXEL mean pixel value to subtract from input (default is 0.0)\n" \
93 " --threshold=THRESHOLD minimum threshold for detection (default is 0.5)\n" \
94 " --overlay=OVERLAY detection overlay flags (e.g. --overlay=links,keypoints)\n" \
95 " valid combinations are: 'box', 'links', 'keypoints', 'none'\n" \
96 " --keypoint-scale=X radius scale for keypoints, relative to image (default: 0.0052)\n" \
97 " --link-scale=X line width scale for links, relative to image (default: 0.0013)\n" \
98 " --profile enable layer profiling in TensorRT\n\n"
132 std::vector<std::array<uint32_t, 2>>
Links;
138 inline int FindLink(uint32_t a, uint32_t b)
const;
182 static poseNet*
Create(
const char* model_path,
const char* topology,
const char* colors,
220 template<
typename T>
bool Process( T* image, uint32_t width, uint32_t height, std::vector<ObjectPose>& poses, uint32_t overlay=
OVERLAY_DEFAULT ) {
return Process((
void*)image, width, height, imageFormatFromType<T>(), poses, overlay); }
241 template<
typename T>
bool Process( T* image, uint32_t width, uint32_t height, uint32_t overlay=
OVERLAY_DEFAULT ) {
return Process((
void*)image, width, height, imageFormatFromType<T>(), overlay); }
256 template<
typename T>
bool Overlay( T* input, T* output, uint32_t width, uint32_t height,
const std::vector<ObjectPose>& poses, uint32_t overlay=
OVERLAY_DEFAULT ) {
return Overlay((
void*)input, (
void*)output, width, height, imageFormatFromType<T>(), overlay); }
261 bool Overlay(
void* input,
void* output, uint32_t width, uint32_t height,
imageFormat format,
const std::vector<ObjectPose>& poses, uint32_t overlay=
OVERLAY_DEFAULT );
353 bool init(
const char* model_path,
const char* topology,
const char* colors,
float threshold,
354 const char* input,
const char* cmap,
const char* paf, uint32_t maxBatchSize,
357 bool postProcess(std::vector<ObjectPose>& poses, uint32_t width, uint32_t height);
393 for( uint32_t n=0; n < numKeypoints; n++ )
405 const uint32_t numKeypoints =
Keypoints.size();
407 for( uint32_t n=0; n < numKeypoints; n++ )
419 const uint32_t numLinks = Links.size();
421 for( uint32_t n=0; n < numLinks; n++ )
423 if( a == Keypoints[Links[n][0]].ID && b == Keypoints[Links[n][1]].ID )
435 for( uint32_t n=0; n < numKeypoints; n++ )
void SetLinkScale(float scale)
Set the scale used to calculate the width of link lines.
Definition: poseNet.h:333
int * mObjects
Definition: poseNet.h:374
bool Overlay(T *input, T *output, uint32_t width, uint32_t height, const std::vector< ObjectPose > &poses, uint32_t overlay=OVERLAY_DEFAULT)
Overlay the results on the image.
Definition: poseNet.h:256
bool init(const char *model_path, const char *topology, const char *colors, float threshold, const char *input, const char *cmap, const char *paf, uint32_t maxBatchSize, precisionType precision, deviceType device, bool allowGPUFallback)
float Bottom
Bounding box bottom, as determined by the bottom-most keypoint in the pose.
Definition: poseNet.h:119
const char * GetKeypointName(uint32_t index) const
Get the name of a keypoint in the topology by it's ID.
Definition: poseNet.h:286
std::string category
Definition: poseNet.h:344
static const int PAF_INTEGRAL_SAMPLES
Definition: poseNet.h:338
@ OVERLAY_KEYPOINTS
Overlay the keypoints (joints) as circles.
Definition: poseNet.h:149
uchar3 color
The RGB color of the point.
Definition: cudaPointCloud.h:11
void * mAssignmentWorkspace
Definition: poseNet.h:380
int * mPeakCounts
Definition: poseNet.h:372
#define POSENET_USAGE_STRING
Standard command-line options able to be passed to poseNet::Create()
Definition: poseNet.h:81
virtual ~poseNet()
Destory.
float * mScoreGraph
Definition: poseNet.h:378
static uint32_t OverlayFlagsFromStr(const char *flags)
Parse a string sequence into OverlayFlags enum.
bool loadKeypointColors(const char *filename)
float * mRefinedPeaks
Definition: poseNet.h:377
float GetKeypointScale() const
Get the scale used to calculate the radius of keypoints relative to input image dimensions.
Definition: poseNet.h:316
float Top
Bounding box top, as determined by the top-most keypoint in the pose.
Definition: poseNet.h:118
float mThreshold
Definition: poseNet.h:364
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
bool postProcess(std::vector< ObjectPose > &poses, uint32_t width, uint32_t height)
void SetKeypointScale(float scale)
Set the scale used to calculate the radius of keypoint circles.
Definition: poseNet.h:322
#define POSENET_DEFAULT_PAF
Name of default output blob of the Part Affinity Field (PAF) for pose estimation ONNX model.
Definition: poseNet.h:49
std::vector< std::string > keypoints
Definition: poseNet.h:345
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
int FindKeypoint(uint32_t id) const
Find a link index by two keypoint ID's, or return -1 if not found.
Definition: poseNet.h:403
uint32_t GetNumKeypoints() const
Get the number of keypoints in the topology.
Definition: poseNet.h:281
#define POSENET_DEFAULT_INPUT
Name of default input blob for pose estimation ONNX model.
Definition: poseNet.h:37
float mLinkScale
Definition: poseNet.h:365
OverlayFlags
Overlay flags (can be OR'd together).
Definition: poseNet.h:144
float GetLinkScale() const
Get the scale used to calculate the width of link lines relative to input image dimensions.
Definition: poseNet.h:327
int * mConnections
Definition: poseNet.h:373
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
static const int CMAP_WINDOW_SIZE
Definition: poseNet.h:337
@ OVERLAY_DEFAULT
Definition: poseNet.h:150
void * mConnectionWorkspace
Definition: poseNet.h:381
Topology mTopology
Definition: poseNet.h:362
static const char * Usage()
Usage string for command line arguments to Create()
Definition: poseNet.h:204
int numLinks
Definition: poseNet.h:347
A keypoint or joint in the topology.
Definition: poseNet.h:124
static poseNet * Create(const char *network="resnet18-body", float threshold=POSENET_DEFAULT_THRESHOLD, uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load a pre-trained model.
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
bool Process(T *image, uint32_t width, uint32_t height, uint32_t overlay=OVERLAY_DEFAULT)
Perform pose estimation on the given image, and overlay the results.
Definition: poseNet.h:241
void SetKeypointColor(uint32_t index, const float4 &color)
Set the overlay color for a keypoint.
Definition: poseNet.h:301
int * mPeaks
Definition: poseNet.h:371
float GetThreshold() const
Retrieve the minimum confidence threshold.
Definition: poseNet.h:266
@ OVERLAY_NONE
No overlay.
Definition: poseNet.h:146
float4 GetKeypointColor(uint32_t index) const
Get the overlay color of a keypoint.
Definition: poseNet.h:296
float Right
Bounding box right, as determined by the right-most keypoint in the pose.
Definition: poseNet.h:117
void SetKeypointAlpha(uint32_t index, float alpha)
Set the alpha channel for a keypoint color (between 0-255).
Definition: poseNet.h:306
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
int FindLink(uint32_t a, uint32_t b) const
Definition: poseNet.h:417
static const int MAX_OBJECTS
Definition: poseNet.h:340
const char * GetCategory() const
Get the category of objects that are detected (e.g.
Definition: poseNet.h:276
float Left
Bounding box left, as determined by the left-most keypoint in the pose.
Definition: poseNet.h:116
#define POSENET_DEFAULT_CMAP
Name of default output blob of the confidence map for pose estimation ONNX model.
Definition: poseNet.h:43
bool loadTopology(const char *json_path, Topology *topology)
void SetThreshold(float threshold)
Set the minimum confidence threshold.
Definition: poseNet.h:271
float x
The x coordinate of the keypoint.
Definition: poseNet.h:127
bool Process(T *image, uint32_t width, uint32_t height, std::vector< ObjectPose > &poses, uint32_t overlay=OVERLAY_DEFAULT)
Perform pose estimation on the given image, returning object poses, and overlay the results.
Definition: poseNet.h:220
std::vector< std::array< uint32_t, 2 > > Links
List of links in the object.
Definition: poseNet.h:132
#define POSENET_DEFAULT_THRESHOLD
Default value of the minimum confidence threshold.
Definition: poseNet.h:55
uint32_t ID
Object ID in the image frame, starting with 0.
Definition: poseNet.h:114
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
int mNumObjects
Definition: poseNet.h:375
@ OVERLAY_LINKS
Overlay the skeleton links (bones) as lines
Definition: poseNet.h:148
uint32_t ID
Type ID of the keypoint - the name can be retrieved with poseNet::GetKeypointName()
Definition: poseNet.h:126
int FindKeypointID(const char *name) const
Find the ID of a keypoint by name, or return -1 if not found.
Definition: poseNet.h:386
static const int MAX_LINKS
Definition: poseNet.h:339
float mKeypointScale
Definition: poseNet.h:366
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
float4 * mKeypointColors
Definition: poseNet.h:368
float y
The y coordinate of the keypoint.
Definition: poseNet.h:128
Definition: poseNet.h:342
int links[MAX_LINKS *4]
Definition: poseNet.h:346
@ OVERLAY_BOX
Overlay object bounding boxes.
Definition: poseNet.h:147
The pose of an object, composed of links between keypoints.
Definition: poseNet.h:112
Pose estimation models with TensorRT support.
Definition: poseNet.h:105
__device__ cudaVectorTypeInfo< T >::Base alpha(T vec, typename cudaVectorTypeInfo< T >::Base default_alpha=255)
Definition: cudaVector.h:98
std::vector< Keypoint > Keypoints
List of keypoints in the object, which contain the keypoint ID and x/y coordinates.
Definition: poseNet.h:131