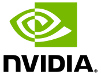 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GSTREAMER_DECODER_H__
24 #define __GSTREAMER_DECODER_H__
105 static const uint32_t
Type = (1 << 1);
142 static void onEOS(_GstAppSink* sink,
void* user_data);
144 static GstFlowReturn
onPreroll(_GstAppSink* sink,
void* user_data);
145 static GstFlowReturn
onBuffer(_GstAppSink* sink,
void* user_data);
_GstAppSink * mAppSink
Definition: gstDecoder.h:152
virtual bool Capture(void **image, imageFormat format, uint64_t timeout=DEFAULT_TIMEOUT, int *status=NULL)
Capture the next decoded frame.
Event object for signalling other threads.
Definition: Event.h:33
Hardware-accelerated video decoder for Jetson using GStreamer.
Definition: gstDecoder.h:52
static GstFlowReturn onBuffer(_GstAppSink *sink, void *user_data)
bool mEOS
Definition: gstDecoder.h:158
virtual uint32_t GetType() const
Return the interface type (gstDecoder::Type)
Definition: gstDecoder.h:100
bool mCustomSize
Definition: gstDecoder.h:156
WebRTC signalling server for establishing and negotiating connections with peers for bi-directional m...
Definition: WebRTCServer.h:116
virtual void Close()
Close the stream.
gstBufferManager recieves GStreamer buffers from appsink elements and unpacks/maps them into CUDA add...
Definition: gstBufferManager.h:61
virtual bool Open()
Open the stream.
static gstDecoder * Create(const videoOptions &options)
Create a decoder from the provided video options.
bool IsEOS() const
Return true if End Of Stream (EOS) has been reached.
Definition: gstDecoder.h:95
gstDecoder(const videoOptions &options)
static bool IsSupportedExtension(const char *ext)
Return true if the extension is in the list of SupportedExtensions.
std::string mLaunchStr
Definition: gstDecoder.h:155
bool isLooping() const
Definition: gstDecoder.h:139
Codec
Video codecs.
Definition: videoOptions.h:198
static const char * SupportedExtensions[]
String array of supported video file extensions, terminated with a NULL sentinel value.
Definition: gstDecoder.h:118
Resource URI of a video device, IP stream, or file/directory.
Definition: URI.h:101
bool mCustomRate
Definition: gstDecoder.h:157
static void onEOS(_GstAppSink *sink, void *user_data)
static GstFlowReturn onPreroll(_GstAppSink *sink, void *user_data)
int loop
Control the number of loops for videoSource disk-based inputs (for example, the number of times that ...
Definition: videoOptions.h:119
videoOptions mOptions
Definition: videoSource.h:378
static void onWebsocketMessage(WebRTCPeer *peer, const char *message, size_t message_size, void *user_data)
GstElement * mPipeline
Definition: gstDecoder.h:151
size_t mLoopCount
Definition: gstDecoder.h:159
gstBufferManager * mBufferManager
Definition: gstDecoder.h:161
bool mWebRTCConnected
Definition: gstDecoder.h:164
static const uint64_t DEFAULT_TIMEOUT
The default Capture timeout (1000ms)
Definition: videoSource.h:371
Event mWaitEvent
Definition: gstDecoder.h:154
GstBus * mBus
Definition: gstDecoder.h:150
WebRTCServer * mWebRTCServer
Definition: gstDecoder.h:163
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
Remote peer that has connected.
Definition: WebRTCServer.h:85
static const uint32_t Type
Unique type identifier of gstDecoder class.
Definition: gstDecoder.h:105
The videoSource API is for capturing frames from video input devices such as MIPI CSI cameras,...
Definition: videoSource.h:118