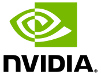 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CUDA_GRAYSCALE_CONVERT_H
24 #define __CUDA_GRAYSCALE_CONVERT_H
42 cudaError_t
cudaGray8ToGray32( uint8_t* input,
float* output,
size_t width,
size_t height );
54 cudaError_t
cudaGray32ToGray8(
float* input, uint8_t* output,
size_t width,
size_t height,
76 cudaError_t
cudaRGB8ToGray8( uchar3* input, uint8_t* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
86 cudaError_t
cudaRGBA8ToGray8( uchar4* input, uint8_t* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
101 cudaError_t
cudaRGB32ToGray8( float3* input, uint8_t* output,
size_t width,
size_t height,
102 bool swapRedBlue=
false,
const float2& pixelRange=
make_float2(0,255) );
117 cudaError_t
cudaRGBA32ToGray8( float4* input, uint8_t* output,
size_t width,
size_t height,
118 bool swapRedBlue=
false,
const float2& pixelRange=
make_float2(0,255) );
139 cudaError_t
cudaRGB8ToGray32( uchar3* input,
float* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
149 cudaError_t
cudaRGBA8ToGray32( uchar4* input,
float* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
159 cudaError_t
cudaRGB32ToGray32( float3* input,
float* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
169 cudaError_t
cudaRGBA32ToGray32( float4* input,
float* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
186 cudaError_t
cudaGray8ToRGB8( uint8_t* input, uchar3* output,
size_t width,
size_t height );
192 cudaError_t
cudaGray8ToRGBA8( uint8_t* input, uchar4* output,
size_t width,
size_t height );
198 cudaError_t
cudaGray8ToRGB32( uint8_t* input, float3* output,
size_t width,
size_t height );
204 cudaError_t
cudaGray8ToRGBA32( uint8_t* input, float4* output,
size_t width,
size_t height );
228 cudaError_t
cudaGray32ToRGB8(
float* input, uchar3* output,
size_t width,
size_t height,
241 cudaError_t
cudaGray32ToRGBA8(
float* input, uchar4* output,
size_t width,
size_t height,
248 cudaError_t
cudaGray32ToRGB32(
float* input, float3* output,
size_t width,
size_t height );
254 cudaError_t
cudaGray32ToRGBA32(
float* input, float4* output,
size_t width,
size_t height );
cudaError_t cudaRGB8ToGray32(uchar3 *input, float *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar3 RGB/BGR image into float grayscale.
cudaError_t cudaGray32ToGray8(float *input, uint8_t *output, size_t width, size_t height, const float2 &pixelRange=make_float2(0, 255))
Convert float grayscale image into uint8 grayscale.
cudaError_t cudaRGBA8ToGray32(uchar4 *input, float *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar4 RGBA/BGRA image into float grayscale.
cudaError_t cudaRGBA32ToGray32(float4 *input, float *output, size_t width, size_t height, bool swapRedBlue=false)
Convert float4 RGB/BGR image into float grayscale.
cudaError_t cudaGray32ToRGB8(float *input, uchar3 *output, size_t width, size_t height, const float2 &pixelRange=make_float2(0, 255))
Convert float grayscale image into uchar3 RGB/BGR.
cudaError_t cudaGray8ToRGBA32(uint8_t *input, float4 *output, size_t width, size_t height)
Convert uint8 grayscale image into float4 RGB/BGR.
cudaError_t cudaGray8ToRGB32(uint8_t *input, float3 *output, size_t width, size_t height)
Convert uint8 grayscale image into float3 RGB/BGR.
cudaError_t cudaGray8ToRGB8(uint8_t *input, uchar3 *output, size_t width, size_t height)
Convert uint8 grayscale image into uchar3 RGB/BGR.
cudaError_t cudaRGBA32ToGray8(float4 *input, uint8_t *output, size_t width, size_t height, bool swapRedBlue=false, const float2 &pixelRange=make_float2(0, 255))
Convert float4 RGBA/BGRA image into uint8 grayscale.
cudaError_t cudaGray32ToRGBA8(float *input, uchar4 *output, size_t width, size_t height, const float2 &pixelRange=make_float2(0, 255))
Convert float grayscale image into uchar4 RGB/BGR.
cudaError_t cudaGray8ToGray32(uint8_t *input, float *output, size_t width, size_t height)
Convert uint8 grayscale image into float grayscale.
cudaError_t cudaGray32ToRGB32(float *input, float3 *output, size_t width, size_t height)
Convert float grayscale image into float3 RGB/BGR.
cudaError_t cudaRGBA8ToGray8(uchar4 *input, uint8_t *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar4 RGBA/BGRA image into uint8 grayscale.
cudaError_t cudaRGB32ToGray8(float3 *input, uint8_t *output, size_t width, size_t height, bool swapRedBlue=false, const float2 &pixelRange=make_float2(0, 255))
Convert float3 RGB/BGR image into uint8 grayscale.
cudaError_t cudaGray32ToRGBA32(float *input, float4 *output, size_t width, size_t height)
Convert float grayscale image into float4 RGB/BGR.
cudaError_t cudaGray8ToRGBA8(uint8_t *input, uchar4 *output, size_t width, size_t height)
Convert uint8 grayscale image into uchar4 RGB/BGR.
__host__ __device__ float2 make_float2(float s)
Definition: cudaMath.h:81
cudaError_t cudaRGB32ToGray32(float3 *input, float *output, size_t width, size_t height, bool swapRedBlue=false)
Convert float3 RGB/BGR image into float grayscale.
cudaError_t cudaRGB8ToGray8(uchar3 *input, uint8_t *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar3 RGB/BGR image into uint8 grayscale.