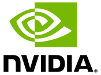 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
24 #ifndef TINYXML2_INCLUDED
25 #define TINYXML2_INCLUDED
27 #if defined(ANDROID_NDK) || defined(__BORLANDC__) || defined(__QNXNTO__)
56 #if defined( _DEBUG ) || defined (__DEBUG__)
57 # ifndef TINYXML2_DEBUG
58 # define TINYXML2_DEBUG
63 # pragma warning(push)
64 # pragma warning(disable: 4251)
68 # ifdef TINYXML2_EXPORT
69 # define TINYXML2_LIB __declspec(dllexport)
70 # elif defined(TINYXML2_IMPORT)
71 # define TINYXML2_LIB __declspec(dllimport)
76 # define TINYXML2_LIB __attribute__((visibility("default")))
82 #if defined(TINYXML2_DEBUG)
83 # if defined(_MSC_VER)
84 # // "(void)0," is for suppressing C4127 warning in "assert(false)", "assert(true)" and the like
85 # define TIXMLASSERT( x ) if ( !((void)0,(x))) { __debugbreak(); }
86 # elif defined (ANDROID_NDK)
87 # include <android/log.h>
88 # define TIXMLASSERT( x ) if ( !(x)) { __android_log_assert( "assert", "grinliz", "ASSERT in '%s' at %d.", __FILE__, __LINE__ ); }
91 # define TIXMLASSERT assert
94 # define TIXMLASSERT( x ) {}
101 static const int TIXML2_MAJOR_VERSION = 6;
102 static const int TIXML2_MINOR_VERSION = 2;
103 static const int TIXML2_PATCH_VERSION = 0;
105 #define TINYXML2_MAJOR_VERSION 6
106 #define TINYXML2_MINOR_VERSION 2
107 #define TINYXML2_PATCH_VERSION 0
114 static const int TINYXML2_MAX_ELEMENT_DEPTH = 100;
149 StrPair() : _flags( 0 ), _start( 0 ), _end( 0 ) {}
152 void Set(
char* start,
char* end,
int flags ) {
158 _flags = flags | NEEDS_FLUSH;
164 return _start == _end;
169 _start =
const_cast<char*
>(str);
172 void SetStr(
const char* str,
int flags=0 );
174 char*
ParseText(
char* in,
const char* endTag,
int strFlags,
int* curLineNumPtr );
181 void CollapseWhitespace();
193 void operator=(
StrPair& other );
202 template <
class T,
int INITIAL_SIZE>
208 _allocated( INITIAL_SIZE ),
214 if ( _mem != _pool ) {
225 EnsureCapacity( _size+1 );
233 EnsureCapacity( _size+count );
234 T* ret = &_mem[_size];
266 return _mem[ _size - 1];
282 _mem[i] = _mem[_size - 1];
300 void EnsureCapacity(
int cap ) {
302 if ( cap > _allocated ) {
304 int newAllocated = cap * 2;
305 T* newMem =
new T[newAllocated];
307 memcpy( newMem, _mem,
sizeof(T)*_size );
308 if ( _mem != _pool ) {
312 _allocated = newAllocated;
317 T _pool[INITIAL_SIZE];
334 virtual void*
Alloc() = 0;
335 virtual void Free(
void* ) = 0;
337 virtual void Clear() = 0;
344 template<
int ITEM_SIZE >
348 MemPoolT() : _blockPtrs(), _root(0), _currentAllocs(0), _nAllocs(0), _maxAllocs(0), _nUntracked(0) {}
355 while( !_blockPtrs.
Empty()) {
356 Block* lastBlock = _blockPtrs.
Pop();
370 return _currentAllocs;
376 Block* block =
new Block();
377 _blockPtrs.
Push( block );
379 Item* blockItems = block->items;
381 blockItems[i].next = &(blockItems[i + 1]);
386 Item*
const result = _root;
391 if ( _currentAllocs > _maxAllocs ) {
392 _maxAllocs = _currentAllocs;
399 virtual void Free(
void* mem ) {
404 Item* item =
static_cast<Item*
>( mem );
405 #ifdef TINYXML2_DEBUG
406 memset( item, 0xfe,
sizeof( *item ) );
412 printf(
"Mempool %s watermark=%d [%dk] current=%d size=%d nAlloc=%d blocks=%d\n",
413 name, _maxAllocs, _maxAllocs * ITEM_SIZE / 1024, _currentAllocs,
414 ITEM_SIZE, _nAllocs, _blockPtrs.
Size() );
444 char itemData[ITEM_SIZE];
449 DynArray< Block*, 10 > _blockPtrs;
557 while( IsWhiteSpace(*p) ) {
558 if (curLineNumPtr && *p ==
'\n') {
567 return const_cast<char*
>( SkipWhiteSpace(
const_cast<const char*
>(p), curLineNumPtr ) );
573 return !IsUTF8Continuation(p) && isspace(
static_cast<unsigned char>(p) );
581 if ( isalpha( ch ) ) {
584 return ch ==
':' || ch ==
'_';
588 return IsNameStartChar( ch )
594 inline static bool StringEqual(
const char* p,
const char* q,
int nChar=INT_MAX ) {
601 return strncmp( p, q, nChar ) == 0;
605 return ( p & 0x80 ) != 0;
608 static const char* ReadBOM(
const char* p,
bool* hasBOM );
611 static const char* GetCharacterRef(
const char* p,
char* value,
int*
length );
612 static void ConvertUTF32ToUTF8(
unsigned long input,
char* output,
int*
length );
615 static void ToStr(
int v,
char* buffer,
int bufferSize );
616 static void ToStr(
unsigned v,
char* buffer,
int bufferSize );
617 static void ToStr(
bool v,
char* buffer,
int bufferSize );
618 static void ToStr(
float v,
char* buffer,
int bufferSize );
619 static void ToStr(
double v,
char* buffer,
int bufferSize );
620 static void ToStr(int64_t v,
char* buffer,
int bufferSize);
623 static bool ToInt(
const char* str,
int* value );
624 static bool ToUnsigned(
const char* str,
unsigned* value );
625 static bool ToBool(
const char* str,
bool* value );
626 static bool ToFloat(
const char* str,
float* value );
627 static bool ToDouble(
const char* str,
double* value );
628 static bool ToInt64(
const char* str, int64_t* value);
635 static void SetBoolSerialization(
const char* writeTrue,
const char* writeFalse);
638 static const char* writeBoolTrue;
639 static const char* writeBoolFalse;
738 const char* Value()
const;
743 void SetValue(
const char* val,
bool staticMem=
false );
774 const XMLElement* FirstChildElement(
const char* name = 0 )
const;
777 return const_cast<XMLElement*
>(
const_cast<const XMLNode*
>(
this)->FirstChildElement( name ));
792 const XMLElement* LastChildElement(
const char* name = 0 )
const;
795 return const_cast<XMLElement*
>(
const_cast<const XMLNode*
>(
this)->LastChildElement(name) );
808 const XMLElement* PreviousSiblingElement(
const char* name = 0 )
const ;
811 return const_cast<XMLElement*
>(
const_cast<const XMLNode*
>(
this)->PreviousSiblingElement( name ) );
824 const XMLElement* NextSiblingElement(
const char* name = 0 )
const;
827 return const_cast<XMLElement*
>(
const_cast<const XMLNode*
>(
this)->NextSiblingElement( name ) );
840 return InsertEndChild( addThis );
863 void DeleteChildren();
868 void DeleteChild(
XMLNode* node );
902 virtual bool ShallowEqual(
const XMLNode* compare )
const = 0;
926 virtual bool Accept(
XMLVisitor* visitor )
const = 0;
946 virtual char* ParseDeep(
char* p,
StrPair* parentEndTag,
int* curLineNumPtr);
964 static void DeleteNode(
XMLNode* node );
965 void InsertChildPreamble(
XMLNode* insertThis )
const;
966 const XMLElement* ToElementWithName(
const char* name )
const;
989 virtual bool Accept(
XMLVisitor* visitor )
const;
1008 virtual bool ShallowEqual(
const XMLNode* compare )
const;
1014 char* ParseDeep(
char* p,
StrPair* parentEndTag,
int* curLineNumPtr );
1036 virtual bool Accept(
XMLVisitor* visitor )
const;
1039 virtual bool ShallowEqual(
const XMLNode* compare )
const;
1045 char* ParseDeep(
char* p,
StrPair* parentEndTag,
int* curLineNumPtr);
1075 virtual bool Accept(
XMLVisitor* visitor )
const;
1078 virtual bool ShallowEqual(
const XMLNode* compare )
const;
1084 char* ParseDeep(
char* p,
StrPair* parentEndTag,
int* curLineNumPtr );
1110 virtual bool Accept(
XMLVisitor* visitor )
const;
1113 virtual bool ShallowEqual(
const XMLNode* compare )
const;
1119 char* ParseDeep(
char* p,
StrPair* parentEndTag,
int* curLineNumPtr );
1139 const char* Name()
const;
1142 const char* Value()
const;
1164 QueryInt64Value(&i);
1171 QueryUnsignedValue( &i );
1177 QueryBoolValue( &b );
1183 QueryDoubleValue( &d );
1189 QueryFloatValue( &f );
1197 XMLError QueryIntValue(
int* value )
const;
1199 XMLError QueryUnsignedValue(
unsigned int* value )
const;
1201 XMLError QueryInt64Value(int64_t* value)
const;
1203 XMLError QueryBoolValue(
bool* value )
const;
1205 XMLError QueryDoubleValue(
double* value )
const;
1207 XMLError QueryFloatValue(
float* value )
const;
1210 void SetAttribute(
const char* value );
1212 void SetAttribute(
int value );
1214 void SetAttribute(
unsigned value );
1216 void SetAttribute(int64_t value);
1218 void SetAttribute(
bool value );
1220 void SetAttribute(
double value );
1222 void SetAttribute(
float value );
1225 enum { BUF_SIZE = 200 };
1227 XMLAttribute() : _name(), _value(),_parseLineNum( 0 ), _next( 0 ), _memPool( 0 ) {}
1228 virtual ~XMLAttribute() {}
1230 XMLAttribute(
const XMLAttribute& );
1231 void operator=(
const XMLAttribute& );
1232 void SetName(
const char* name );
1234 char* ParseDeep(
char* p,
bool processEntities,
int* curLineNumPtr );
1236 mutable StrPair _name;
1237 mutable StrPair _value;
1239 XMLAttribute* _next;
1257 void SetName(
const char* str,
bool staticMem=
false ) {
1258 SetValue( str, staticMem );
1267 virtual bool Accept(
XMLVisitor* visitor )
const;
1292 const char* Attribute(
const char* name,
const char* value=0 )
const;
1300 int IntAttribute(
const char* name,
int defaultValue = 0)
const;
1302 unsigned UnsignedAttribute(
const char* name,
unsigned defaultValue = 0)
const;
1304 int64_t Int64Attribute(
const char* name, int64_t defaultValue = 0)
const;
1306 bool BoolAttribute(
const char* name,
bool defaultValue =
false)
const;
1308 double DoubleAttribute(
const char* name,
double defaultValue = 0)
const;
1310 float FloatAttribute(
const char* name,
float defaultValue = 0)
const;
1382 *value = a->
Value();
1406 return QueryIntAttribute( name, value );
1410 return QueryUnsignedAttribute( name, value );
1414 return QueryInt64Attribute(name, value);
1418 return QueryBoolAttribute( name, value );
1422 return QueryDoubleAttribute( name, value );
1426 return QueryFloatAttribute( name, value );
1470 void DeleteAttribute(
const char* name );
1474 return _rootAttribute;
1477 const XMLAttribute* FindAttribute(
const char* name )
const;
1507 const char* GetText()
const;
1543 void SetText(
const char* inText );
1545 void SetText(
int value );
1547 void SetText(
unsigned value );
1549 void SetText(int64_t value);
1551 void SetText(
bool value );
1553 void SetText(
double value );
1555 void SetText(
float value );
1583 XMLError QueryIntText(
int* ival )
const;
1585 XMLError QueryUnsignedText(
unsigned* uval )
const;
1587 XMLError QueryInt64Text(int64_t* uval)
const;
1589 XMLError QueryBoolText(
bool* bval )
const;
1591 XMLError QueryDoubleText(
double* dval )
const;
1593 XMLError QueryFloatText(
float* fval )
const;
1595 int IntText(
int defaultValue = 0)
const;
1598 unsigned UnsignedText(
unsigned defaultValue = 0)
const;
1600 int64_t Int64Text(int64_t defaultValue = 0)
const;
1602 bool BoolText(
bool defaultValue =
false)
const;
1604 double DoubleText(
double defaultValue = 0)
const;
1606 float FloatText(
float defaultValue = 0)
const;
1615 return _closingType;
1618 virtual bool ShallowEqual(
const XMLNode* compare )
const;
1621 char* ParseDeep(
char* p,
StrPair* parentEndTag,
int* curLineNumPtr );
1632 XMLAttribute* FindOrCreateAttribute(
const char* name );
1634 char* ParseAttributes(
char* p,
int* curLineNumPtr );
1635 static void DeleteAttribute( XMLAttribute* attribute );
1636 XMLAttribute* CreateAttribute();
1638 enum { BUF_SIZE = 200 };
1639 ElementClosingType _closingType;
1643 XMLAttribute* _rootAttribute;
1692 XMLError Parse(
const char* xml,
size_t nBytes=(
size_t)(-1) );
1699 XMLError LoadFile(
const char* filename );
1719 XMLError SaveFile(
const char* filename,
bool compact =
false );
1728 XMLError SaveFile( FILE* fp,
bool compact =
false );
1731 return _processEntities;
1734 return _whitespaceMode;
1753 return FirstChildElement();
1756 return FirstChildElement();
1774 virtual bool Accept(
XMLVisitor* visitor )
const;
1787 XMLComment* NewComment(
const char* comment );
1793 XMLText* NewText(
const char* text );
1817 void DeleteNode(
XMLNode* node );
1831 const char* ErrorName()
const;
1832 static const char* ErrorIDToName(
XMLError errorID);
1837 const char* ErrorStr()
const;
1840 void PrintError()
const;
1845 return _errorLineNum;
1861 char* Identify(
char* p,
XMLNode** node );
1878 bool _processEntities;
1884 int _parseCurLineNum;
1903 void SetError(
XMLError error,
int lineNum,
const char* format, ... );
1908 class DepthTracker {
1911 this->_document = document;
1912 document->PushDepth();
1915 _document->PopDepth();
1918 XMLDocument * _document;
1923 template<
class NodeType,
int PoolElementSize>
1924 NodeType* CreateUnlinkedNode( MemPoolT<PoolElementSize>& pool );
1927 template<
class NodeType,
int PoolElementSize>
1928 inline NodeType* XMLDocument::CreateUnlinkedNode( MemPoolT<PoolElementSize>& pool )
1930 TIXMLASSERT(
sizeof( NodeType ) == PoolElementSize );
1931 TIXMLASSERT(
sizeof( NodeType ) == pool.ItemSize() );
1932 NodeType* returnNode =
new (pool.Alloc()) NodeType(
this );
1934 returnNode->_memPool = &pool;
1936 _unlinked.
Push(returnNode);
2015 return XMLHandle( _node ? _node->FirstChild() : 0 );
2019 return XMLHandle( _node ? _node->FirstChildElement( name ) : 0 );
2023 return XMLHandle( _node ? _node->LastChild() : 0 );
2027 return XMLHandle( _node ? _node->LastChildElement( name ) : 0 );
2031 return XMLHandle( _node ? _node->PreviousSibling() : 0 );
2035 return XMLHandle( _node ? _node->PreviousSiblingElement( name ) : 0 );
2039 return XMLHandle( _node ? _node->NextSibling() : 0 );
2043 return XMLHandle( _node ? _node->NextSiblingElement( name ) : 0 );
2052 return ( _node ? _node->ToElement() : 0 );
2056 return ( _node ? _node->ToText() : 0 );
2060 return ( _node ? _node->ToUnknown() : 0 );
2064 return ( _node ? _node->ToDeclaration() : 0 );
2095 return XMLConstHandle( _node ? _node->FirstChildElement( name ) : 0 );
2101 return XMLConstHandle( _node ? _node->LastChildElement( name ) : 0 );
2107 return XMLConstHandle( _node ? _node->PreviousSiblingElement( name ) : 0 );
2113 return XMLConstHandle( _node ? _node->NextSiblingElement( name ) : 0 );
2121 return ( _node ? _node->ToElement() : 0 );
2124 return ( _node ? _node->ToText() : 0 );
2127 return ( _node ? _node->ToUnknown() : 0 );
2130 return ( _node ? _node->ToDeclaration() : 0 );
2189 XMLPrinter( FILE* file=0,
bool compact =
false,
int depth = 0 );
2193 void PushHeader(
bool writeBOM,
bool writeDeclaration );
2197 void OpenElement(
const char* name,
bool compactMode=
false );
2199 void PushAttribute(
const char* name,
const char* value );
2200 void PushAttribute(
const char* name,
int value );
2201 void PushAttribute(
const char* name,
unsigned value );
2202 void PushAttribute(
const char* name, int64_t value);
2203 void PushAttribute(
const char* name,
bool value );
2204 void PushAttribute(
const char* name,
double value );
2206 virtual void CloseElement(
bool compactMode=
false );
2209 void PushText(
const char* text,
bool cdata=
false );
2211 void PushText(
int value );
2213 void PushText(
unsigned value );
2215 void PushText(int64_t value);
2217 void PushText(
bool value );
2219 void PushText(
float value );
2221 void PushText(
double value );
2224 void PushComment(
const char* comment );
2226 void PushDeclaration(
const char* value );
2227 void PushUnknown(
const char* value );
2235 virtual bool VisitExit(
const XMLElement& element );
2237 virtual bool Visit(
const XMLText& text );
2238 virtual bool Visit(
const XMLComment& comment );
2240 virtual bool Visit(
const XMLUnknown& unknown );
2247 return _buffer.Mem();
2255 return _buffer.Size();
2264 _firstElement =
true;
2273 virtual void PrintSpace(
int depth );
2274 void Print(
const char* format, ... );
2275 void Write(
const char* data,
size_t size );
2276 inline void Write(
const char* data ) {
Write( data, strlen( data ) ); }
2277 void Putc(
char ch );
2279 void SealElementIfJustOpened();
2284 void PrintString(
const char*,
bool restrictedEntitySet );
2290 bool _processEntities;
2297 bool _entityFlag[ENTITY_RANGE];
2298 bool _restrictedEntityFlag[ENTITY_RANGE];
2310 #if defined(_MSC_VER)
2311 # pragma warning(pop)
2314 #endif // TINYXML2_INCLUDED
int QueryAttribute(const char *name, int *value) const
Given an attribute name, QueryAttribute() returns XML_SUCCESS, XML_WRONG_ATTRIBUTE_TYPE if the conver...
Definition: xml.h:1405
int QueryAttribute(const char *name, int64_t *value) const
Definition: xml.h:1413
virtual void SetTracked()=0
virtual const XMLDocument * ToDocument() const
Definition: xml.h:1677
@ TEXT_ELEMENT_LEAVE_ENTITIES
Definition: xml.h:142
static char * SkipWhiteSpace(char *p, int *curLineNumPtr)
Definition: xml.h:566
bool HasBOM() const
Returns true if this document has a leading Byte Order Mark of UTF8.
Definition: xml.h:1740
void ClearError()
Definition: xml.h:1819
unsigned UnsignedValue() const
Query as an unsigned integer. See IntValue()
Definition: xml.h:1169
const XMLText * ToText() const
Definition: xml.h:2123
virtual const XMLText * ToText() const
Definition: xml.h:713
static bool IsUTF8Continuation(char p)
Definition: xml.h:604
@ ATTRIBUTE_NAME
Definition: xml.h:143
bool Error() const
Return true if there was an error parsing the document.
Definition: xml.h:1824
virtual bool Visit(const XMLComment &)
Visit a comment node.
Definition: xml.h:511
@ XML_NO_TEXT_NODE
Definition: xml.h:541
bool Empty() const
Definition: xml.h:163
int ErrorLineNum() const
Return the line where the error occured, or zero if unknown.
Definition: xml.h:1843
@ ITEMS_PER_BLOCK
Definition: xml.h:436
T * Mem()
Definition: xml.h:291
bool CData() const
Returns true if this is a CDATA text element.
Definition: xml.h:1003
XMLNode * _lastChild
Definition: xml.h:954
virtual XMLDeclaration * ToDeclaration()
Safely cast to a Declaration, or null.
Definition: xml.h:702
virtual XMLElement * ToElement()
Safely cast to an Element, or null.
Definition: xml.h:1261
~DynArray()
Definition: xml.h:213
@ COLLAPSE_WHITESPACE
Definition: xml.h:1649
DynArray< const char *, 10 > _stack
Definition: xml.h:2281
XMLNode * NextSibling()
Definition: xml.h:819
#define TINYXML2_LIB
Definition: xml.h:78
const XMLNode * ToNode() const
Definition: xml.h:2117
void SetAttribute(const char *value)
Set the attribute to a string value.
XMLHandle(const XMLHandle &ref)
Copy constructor.
Definition: xml.h:2005
virtual bool VisitExit(const XMLDocument &)
Visit a document.
Definition: xml.h:489
DynArray()
Definition: xml.h:206
void Trace(const char *name)
Definition: xml.h:411
XMLHandle PreviousSiblingElement(const char *name=0)
Get the previous sibling element of this handle.
Definition: xml.h:2034
@ XML_ERROR_PARSING_UNKNOWN
Definition: xml.h:536
virtual void Free(void *mem)
Definition: xml.h:399
XMLError
Definition: xml.h:521
XMLError QueryBoolAttribute(const char *name, bool *value) const
See QueryIntAttribute()
Definition: xml.h:1352
virtual ~XMLVisitor()
Definition: xml.h:482
XMLNode * _parent
Definition: xml.h:949
bool NoChildren() const
Returns true if this node has no children.
Definition: xml.h:758
~MemPoolT()
Definition: xml.h:349
XMLError QueryStringAttribute(const char *name, const char **value) const
See QueryIntAttribute()
Definition: xml.h:1377
void SetName(const char *str, bool staticMem=false)
Set the name of the element.
Definition: xml.h:1257
@ XML_ERROR_FILE_NOT_FOUND
Definition: xml.h:525
virtual XMLNode * ShallowClone(XMLDocument *) const
Make a copy of this node, but not its children.
Definition: xml.h:1866
virtual const XMLElement * ToElement() const
Definition: xml.h:710
Printing functionality.
Definition: xml.h:2180
@ XML_ERROR_FILE_COULD_NOT_BE_OPENED
Definition: xml.h:526
@ XML_WRONG_ATTRIBUTE_TYPE
Definition: xml.h:524
void SetAttribute(const char *name, const char *value)
Sets the named attribute to value.
Definition: xml.h:1430
const T * Mem() const
Definition: xml.h:286
virtual int ItemSize() const =0
A XMLHandle is a class that wraps a node pointer with null checks; this is an incredibly useful thing...
Definition: xml.h:1995
@ NEEDS_WHITESPACE_COLLAPSING
Definition: xml.h:139
const T & PeekTop() const
Definition: xml.h:264
virtual bool Visit(const XMLUnknown &)
Visit an unknown node.
Definition: xml.h:515
const char * Value() const
The value of the attribute.
double DoubleValue() const
Query as a double. See IntValue()
Definition: xml.h:1181
int QueryAttribute(const char *name, unsigned int *value) const
Definition: xml.h:1409
@ XML_ERROR_PARSING_DECLARATION
Definition: xml.h:535
@ XML_ERROR_FILE_READ_ERROR
Definition: xml.h:527
XMLNode * _prev
Definition: xml.h:956
@ TEXT_ELEMENT
Definition: xml.h:141
virtual const XMLUnknown * ToUnknown() const
Definition: xml.h:1106
@ XML_ERROR_PARSING_ELEMENT
Definition: xml.h:529
XMLError ErrorID() const
Return the errorID.
Definition: xml.h:1828
#define TIXMLASSERT(x)
Definition: xml.h:94
XMLHandle(XMLNode &node)
Create a handle from a node.
Definition: xml.h:2002
virtual bool VisitEnter(const XMLElement &, const XMLAttribute *)
Visit an element.
Definition: xml.h:494
void PopArr(int count)
Definition: xml.h:245
Implements the interface to the "Visitor pattern" (see the Accept() method.) If you call the Accept()...
Definition: xml.h:479
XMLElement * FirstChildElement(const char *name=0)
Definition: xml.h:776
XMLError QueryInt64Attribute(const char *name, int64_t *value) const
See QueryIntAttribute()
Definition: xml.h:1343
const XMLDeclaration * ToDeclaration() const
Definition: xml.h:2129
@ XML_ERROR_PARSING_TEXT
Definition: xml.h:532
char * ParseText(char *in, const char *endTag, int strFlags, int *curLineNumPtr)
Whitespace WhitespaceMode() const
Definition: xml.h:1733
virtual bool VisitExit(const XMLDocument &)
Visit a document.
Definition: xml.h:2230
void SetAttribute(const char *name, double value)
Sets the named attribute to value.
Definition: xml.h:1457
char * ParseName(char *in)
virtual XMLUnknown * ToUnknown()
Safely cast to an Unknown, or null.
Definition: xml.h:1103
virtual const XMLComment * ToComment() const
Definition: xml.h:716
int QueryAttribute(const char *name, bool *value) const
Definition: xml.h:1417
virtual bool VisitExit(const XMLElement &)
Visit an element.
Definition: xml.h:498
const XMLNode * NextSibling() const
Get the next (right) sibling node of this node.
Definition: xml.h:815
const XMLElement * ToElement() const
Definition: xml.h:2120
virtual ~XMLText()
Definition: xml.h:1012
@ UNUSED_XML_ERROR_ELEMENT_MISMATCH
Definition: xml.h:528
int QueryAttribute(const char *name, double *value) const
Definition: xml.h:1421
const XMLDocument * GetDocument() const
Get the XMLDocument that owns this XMLNode.
Definition: xml.h:675
@ XML_ERROR_PARSING_COMMENT
Definition: xml.h:534
XMLHandle & operator=(const XMLHandle &ref)
Assignment.
Definition: xml.h:2008
void Set(char *start, char *end, int flags)
Definition: xml.h:152
XMLConstHandle(const XMLNode *node)
Definition: xml.h:2079
An attribute is a name-value pair.
Definition: xml.h:1134
XMLElement * ToElement()
Safe cast to XMLElement. This can return null.
Definition: xml.h:2051
void SetAttribute(const char *name, bool value)
Sets the named attribute to value.
Definition: xml.h:1452
const XMLConstHandle NextSibling() const
Definition: xml.h:2109
bool ProcessEntities() const
Definition: xml.h:1730
const XMLElement * RootElement() const
Definition: xml.h:1755
const char * CStr() const
If in print to memory mode, return a pointer to the XML file in memory.
Definition: xml.h:2246
XML text.
Definition: xml.h:985
Whitespace
Definition: xml.h:1647
const XMLConstHandle LastChildElement(const char *name=0) const
Definition: xml.h:2100
virtual XMLUnknown * ToUnknown()
Safely cast to an Unknown, or null.
Definition: xml.h:706
const XMLAttribute * FirstAttribute() const
Return the first attribute in the list.
Definition: xml.h:1473
XMLError QueryUnsignedValue(unsigned int *value) const
See QueryIntValue.
XMLError QueryInt64Value(int64_t *value) const
See QueryIntValue.
static bool IsNameStartChar(unsigned char ch)
Definition: xml.h:576
@ XML_SUCCESS
Definition: xml.h:522
int CStrSize() const
If in print to memory mode, return the size of the XML file in memory.
Definition: xml.h:2254
XMLError QueryDoubleValue(double *value) const
See QueryIntValue.
@ COMMENT
Definition: xml.h:146
XMLNode * FirstChild()
Definition: xml.h:767
XMLError QueryBoolValue(bool *value) const
See QueryIntValue.
const XMLNode * FirstChild() const
Get the first child node, or null if none exists.
Definition: xml.h:763
T Pop()
Definition: xml.h:239
int GetLineNum() const
Gets the line number the node is in, if the document was parsed from a file.
Definition: xml.h:746
int Size() const
Definition: xml.h:269
XMLNode * _firstChild
Definition: xml.h:953
@ NEEDS_ENTITY_PROCESSING
Definition: xml.h:137
void SetInternedStr(const char *str)
Definition: xml.h:167
XMLNode * LinkEndChild(XMLNode *addThis)
Definition: xml.h:839
XMLText(XMLDocument *doc)
Definition: xml.h:1011
In correct XML the declaration is the first entry in the file.
Definition: xml.h:1064
bool Empty() const
Definition: xml.h:250
const XMLConstHandle FirstChild() const
Definition: xml.h:2091
Any tag that TinyXML-2 doesn't recognize is saved as an unknown.
Definition: xml.h:1099
void SetAttribute(const char *name, unsigned value)
Sets the named attribute to value.
Definition: xml.h:1440
@ UNUSED_XML_ERROR_IDENTIFYING_TAG
Definition: xml.h:531
void SetCData(bool isCData)
Declare whether this should be CDATA or standard text.
Definition: xml.h:999
const XMLUnknown * ToUnknown() const
Definition: xml.h:2126
XMLText * ToText()
Safe cast to XMLText. This can return null.
Definition: xml.h:2055
ElementClosingType
Definition: xml.h:1609
The element is a container class.
Definition: xml.h:1248
int CurrentAllocs() const
Definition: xml.h:369
XMLHandle LastChild()
Get the last child of this handle.
Definition: xml.h:2022
ElementClosingType ClosingType() const
Definition: xml.h:1614
virtual const XMLDocument * ToDocument() const
Definition: xml.h:719
void TransferTo(StrPair *other)
virtual XMLDocument * ToDocument()
Safely cast to a Document, or null.
Definition: xml.h:1673
const XMLNode * Parent() const
Get the parent of this node on the DOM.
Definition: xml.h:749
void ClearBuffer()
If in print to memory mode, reset the buffer to the beginning.
Definition: xml.h:2261
StrPair _value
Definition: xml.h:950
@ XML_ERROR_PARSING_CDATA
Definition: xml.h:533
virtual bool CompactMode(const XMLElement &)
Definition: xml.h:2268
XMLElement * RootElement()
Return the root element of DOM.
Definition: xml.h:1752
XMLError QueryFloatValue(float *value) const
See QueryIntValue.
void SetUserData(void *userData)
Set user data into the XMLNode.
Definition: xml.h:933
XMLError QueryDoubleAttribute(const char *name, double *value) const
See QueryIntAttribute()
Definition: xml.h:1360
void SetAttribute(const char *name, float value)
Sets the named attribute to value.
Definition: xml.h:1462
void Clear()
Definition: xml.h:219
virtual XMLElement * ToElement()
Safely cast to an Element, or null.
Definition: xml.h:686
virtual ~MemPool()
Definition: xml.h:331
T * PushArr(int count)
Definition: xml.h:230
@ CLOSED
Definition: xml.h:1611
@ ATTRIBUTE_VALUE_LEAVE_ENTITIES
Definition: xml.h:145
void SetStr(const char *str, int flags=0)
void * GetUserData() const
Get user data set into the XMLNode.
Definition: xml.h:940
XMLHandle(XMLNode *node)
Create a handle from any node (at any depth of the tree.) This can be a null pointer.
Definition: xml.h:1999
virtual void * Alloc()
Definition: xml.h:373
virtual int ItemSize() const
Definition: xml.h:366
int _parseLineNum
Definition: xml.h:951
virtual const XMLElement * ToElement() const
Definition: xml.h:1264
const XMLConstHandle LastChild() const
Definition: xml.h:2097
XMLError QueryUnsignedAttribute(const char *name, unsigned int *value) const
See QueryIntAttribute()
Definition: xml.h:1334
MemPool()
Definition: xml.h:330
@ XML_ERROR_PARSING_ATTRIBUTE
Definition: xml.h:530
T & operator[](int i)
Definition: xml.h:254
void SetTracked()
Definition: xml.h:417
virtual ~XMLPrinter()
Definition: xml.h:2190
MemPoolT()
Definition: xml.h:348
const XMLNode * LastChild() const
Get the last child node, or null if none exists.
Definition: xml.h:781
XMLDeclaration * ToDeclaration()
Safe cast to XMLDeclaration. This can return null.
Definition: xml.h:2063
XMLHandle FirstChildElement(const char *name=0)
Get the first child element of this handle.
Definition: xml.h:2018
const T & operator[](int i) const
Definition: xml.h:259
const XMLConstHandle NextSiblingElement(const char *name=0) const
Definition: xml.h:2112
@ PRESERVE_WHITESPACE
Definition: xml.h:1648
XMLNode * _next
Definition: xml.h:957
StrPair()
Definition: xml.h:149
__host__ __device__ float length(float2 v)
Definition: cudaMath.h:1563
void * _userData
Definition: xml.h:959
XMLHandle PreviousSibling()
Get the previous sibling of this handle.
Definition: xml.h:2030
bool BoolValue() const
Query as a boolean. See IntValue()
Definition: xml.h:1175
XMLNode is a base class for every object that is in the XML Document Object Model (DOM),...
Definition: xml.h:668
virtual bool Visit(const XMLText &)
Visit a text node.
Definition: xml.h:507
virtual void Free(void *)=0
XMLConstHandle & operator=(const XMLConstHandle &ref)
Definition: xml.h:2086
XMLElement * PreviousSiblingElement(const char *name=0)
Definition: xml.h:810
virtual const XMLDeclaration * ToDeclaration() const
Definition: xml.h:1071
XMLElement * NextSiblingElement(const char *name=0)
Definition: xml.h:826
XMLNode * ToNode()
Safe cast to XMLNode. This can return null.
Definition: xml.h:2047
void SetAttribute(const char *name, int value)
Sets the named attribute to value.
Definition: xml.h:1435
int QueryAttribute(const char *name, float *value) const
Definition: xml.h:1425
void Push(T t)
Definition: xml.h:223
static bool IsWhiteSpace(char p)
Definition: xml.h:572
const XMLConstHandle PreviousSiblingElement(const char *name=0) const
Definition: xml.h:2106
virtual XMLDeclaration * ToDeclaration()
Safely cast to a Declaration, or null.
Definition: xml.h:1068
XMLElement * LastChildElement(const char *name=0)
Definition: xml.h:794
void Write(const char *data)
Definition: xml.h:2276
virtual XMLText * ToText()
Safely cast to Text, or null.
Definition: xml.h:690
void SetBOM(bool useBOM)
Sets whether to write the BOM when writing the file.
Definition: xml.h:1745
XMLConstHandle(const XMLConstHandle &ref)
Definition: xml.h:2083
XMLError QueryIntAttribute(const char *name, int *value) const
Given an attribute name, QueryIntAttribute() returns XML_SUCCESS, XML_WRONG_ATTRIBUTE_TYPE if the con...
Definition: xml.h:1325
static bool StringEqual(const char *p, const char *q, int nChar=INT_MAX)
Definition: xml.h:594
void SwapRemove(int i)
Definition: xml.h:279
@ XML_CAN_NOT_CONVERT_TEXT
Definition: xml.h:540
XMLUnknown * ToUnknown()
Safe cast to XMLUnknown. This can return null.
Definition: xml.h:2059
A Document binds together all the functionality.
Definition: xml.h:1658
int GetLineNum() const
Gets the line number the attribute is in, if the document was parsed from a file.
Definition: xml.h:1145
XMLNode * Parent()
Definition: xml.h:753
@ XML_ELEMENT_DEPTH_EXCEEDED
Definition: xml.h:542
virtual XMLText * ToText()
Safely cast to Text, or null.
Definition: xml.h:991
virtual XMLComment * ToComment()
Safely cast to a Comment, or null.
Definition: xml.h:694
virtual bool VisitEnter(const XMLDocument &)
Visit a document.
Definition: xml.h:485
float FloatValue() const
Query as a float. See IntValue()
Definition: xml.h:1187
int64_t Int64Value() const
Definition: xml.h:1162
XMLDocument * _document
Definition: xml.h:948
int IntValue() const
IntValue interprets the attribute as an integer, and returns the value.
Definition: xml.h:1156
int Untracked() const
Definition: xml.h:421
@ ATTRIBUTE_VALUE
Definition: xml.h:144
@ NEEDS_NEWLINE_NORMALIZATION
Definition: xml.h:138
virtual const XMLText * ToText() const
Definition: xml.h:994
virtual XMLDocument * ToDocument()
Safely cast to a Document, or null.
Definition: xml.h:698
XMLHandle NextSiblingElement(const char *name=0)
Get the next sibling element of this handle.
Definition: xml.h:2042
static const char * SkipWhiteSpace(const char *p, int *curLineNumPtr)
Definition: xml.h:554
void SetAttribute(const char *name, int64_t value)
Sets the named attribute to value.
Definition: xml.h:1446
@ XML_ERROR_MISMATCHED_ELEMENT
Definition: xml.h:538
static bool IsNameChar(unsigned char ch)
Definition: xml.h:587
const XMLConstHandle FirstChildElement(const char *name=0) const
Definition: xml.h:2094
@ XML_ERROR_COUNT
Definition: xml.h:544
const XMLNode * PreviousSibling() const
Get the previous (left) sibling node of this node.
Definition: xml.h:799
virtual bool ShallowEqual(const XMLNode *) const
Test if 2 nodes are the same, but don't test children.
Definition: xml.h:1869
@ XML_NO_ATTRIBUTE
Definition: xml.h:523
void Clear()
Definition: xml.h:353
virtual const XMLUnknown * ToUnknown() const
Definition: xml.h:725
virtual bool Visit(const XMLDeclaration &)
Visit a declaration.
Definition: xml.h:503
virtual const XMLDeclaration * ToDeclaration() const
Definition: xml.h:722
XMLDocument * GetDocument()
Get the XMLDocument that owns this XMLNode.
Definition: xml.h:680
XMLError QueryFloatAttribute(const char *name, float *value) const
See QueryIntAttribute()
Definition: xml.h:1368
@ XML_ERROR_PARSING
Definition: xml.h:539
XMLNode * PreviousSibling()
Definition: xml.h:803
XMLConstHandle(const XMLNode &node)
Definition: xml.h:2081
@ XML_ERROR_EMPTY_DOCUMENT
Definition: xml.h:537
XMLHandle NextSibling()
Get the next sibling of this handle.
Definition: xml.h:2038
A variant of the XMLHandle class for working with const XMLNodes and Documents.
Definition: xml.h:2076
int Capacity() const
Definition: xml.h:274
XMLHandle FirstChild()
Get the first child of this handle.
Definition: xml.h:2014
XMLHandle LastChildElement(const char *name=0)
Get the last child element of this handle.
Definition: xml.h:2026
XMLError QueryIntValue(int *value) const
QueryIntValue interprets the attribute as an integer, and returns the value in the provided parameter...
const XMLAttribute * Next() const
The next attribute in the list.
Definition: xml.h:1148
@ OPEN
Definition: xml.h:1610
bool _elementJustOpened
Definition: xml.h:2280
XMLNode * LastChild()
Definition: xml.h:785
const XMLConstHandle PreviousSibling() const
Definition: xml.h:2103
const char * Name() const
Get the name of an element (which is the Value() of the node.)
Definition: xml.h:1253