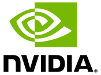 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __VIDEO_OUTPUT_H_
24 #define __VIDEO_OUTPUT_H_
38 #define VIDEO_OUTPUT_USAGE_STRING "videoOutput arguments: \n" \
39 " output resource URI of the output stream, for example:\n" \
40 " * file://my_image.jpg (image file)\n" \
41 " * file://my_video.mp4 (video file)\n" \
42 " * file://my_directory/ (directory of images)\n" \
43 " * rtp://<remote-ip>:1234 (RTP stream)\n" \
44 " * rtsp://@:8554/my_stream (RTSP stream)\n" \
45 " * webrtc://@:1234/my_stream (WebRTC stream)\n" \
46 " * display://0 (OpenGL window)\n" \
47 " --output-codec=CODEC desired codec for compressed output streams:\n" \
48 " * h264 (default), h265\n" \
52 " --output-encoder=TYPE the encoder engine to use, one of these:\n" \
54 " * omx (aarch64/JetPack4 only)\n" \
55 " * v4l2 (aarch64/JetPack5 only)\n" \
56 " --output-save=FILE path to a video file for saving the compressed stream\n" \
57 " to disk, in addition to the primary output above\n" \
58 " --bitrate=BITRATE desired target VBR bitrate for compressed streams,\n" \
59 " in bits per second. The default is 4000000 (4 Mbps)\n" \
60 " --headless don't create a default OpenGL GUI window\n\n"
186 template<
typename T>
bool Render( T* image, uint32_t width, uint32_t height ) {
return Render((
void**)image, width, height, imageFormatFromType<T>()); }
200 virtual bool Render(
void* image, uint32_t width, uint32_t height,
imageFormat format );
221 virtual void Close();
281 virtual void SetStatus(
const char* str );
291 virtual inline uint32_t
GetType()
const {
return 0; }
316 static const char*
TypeToStr( uint32_t type );
virtual void SetStatus(const char *str)
Set a status string (i.e.
#define VIDEO_OUTPUT_USAGE_STRING
Standard command-line options able to be passed to videoOutput::Create()
Definition: videoOutput.h:38
void AddOutput(videoOutput *output)
Add an output sub-stream.
Definition: videoOutput.h:265
videoOutput * GetOutput(uint32_t index) const
Return a sub-stream.
Definition: videoOutput.h:275
uint64_t GetFrameCount() const
Return the number of frames output.
Definition: videoOutput.h:249
virtual bool Open()
Begin streaming the device.
bool IsType() const
Check if a this stream is of a particular type.
Definition: videoOutput.h:306
virtual ~videoOutput()
Destroy interface and release all resources.
uint32_t GetHeight() const
Return the height of the stream, in pixels.
Definition: videoOutput.h:239
bool Render(T *image, uint32_t width, uint32_t height)
Render and output the next frame to the stream.
Definition: videoOutput.h:186
bool mStreaming
Definition: videoOutput.h:321
const URI & GetResource() const
Return the resource URI of the stream.
Definition: videoOutput.h:254
videoOptions mOptions
Definition: videoOutput.h:322
virtual void Close()
Stop streaming the device.
URI resource
The resource URI of the device, IP stream, or file/directory.
Definition: videoOptions.h:49
videoOutput(const videoOptions &options)
uint32_t GetNumOutputs() const
Return the number of sub-streams.
Definition: videoOutput.h:270
bool IsType(uint32_t type) const
Check if this stream is of a particular type.
Definition: videoOutput.h:297
Resource URI of a video device, IP stream, or file/directory.
Definition: URI.h:101
static videoOutput * Create(const videoOptions &options)
Create videoOutput interface from a videoOptions struct that's already been filled out.
static videoOutput * CreateNullOutput()
Create videoOutput interface that acts as a NULL output and does nothing with incoming frames.
const char * TypeToStr() const
Convert this stream's class type to string.
Definition: videoOutput.h:311
uint32_t GetWidth() const
Return the width of the stream, in pixels.
Definition: videoOutput.h:234
static const char * Usage()
Usage string for command line arguments to Create()
Definition: videoOutput.h:167
The videoOutput API is for rendering and transmitting frames to video input devices such as display w...
Definition: videoOutput.h:104
bool IsStreaming() const
Check if the device is actively streaming or not.
Definition: videoOutput.h:229
float GetFrameRate() const
Return the framerate, in Hz or FPS.
Definition: videoOutput.h:244
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
uint32_t width
The width of the stream (in pixels).
Definition: videoOptions.h:64
std::vector< videoOutput * > mOutputs
Definition: videoOutput.h:324
const videoOptions & GetOptions() const
Return the videoOptions of the stream.
Definition: videoOutput.h:259
uint64_t frameCount
The number of frames that have been captured or output on this interface.
Definition: videoOptions.h:83
float frameRate
The framerate of the stream (the default is 30Hz).
Definition: videoOptions.h:78
uint32_t height
The height of the stream (in pixels).
Definition: videoOptions.h:71
virtual uint32_t GetType() const
Return the interface type of the stream.
Definition: videoOutput.h:291