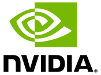 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __TIMESPEC_UTIL_H__
24 #define __TIMESPEC_UTIL_H__
37 inline void timestamp( timespec* timestampOut ) {
if(!timestampOut)
return; timestampOut->tv_sec=0; timestampOut->tv_nsec=0; clock_gettime(CLOCK_REALTIME, timestampOut); }
49 inline timespec
timeZero() { timespec t; t.tv_sec=0; t.tv_nsec=0;
return t; }
55 inline timespec
timeNew( time_t seconds,
long int nanoseconds ) { timespec t; t.tv_sec=seconds; t.tv_nsec=nanoseconds;
return t; }
61 inline timespec
timeNew(
long int nanoseconds ) {
const time_t sec=nanoseconds/1e+9;
return timeNew(sec, nanoseconds-sec*1e+9); }
67 inline timespec
timeAdd(
const timespec& a,
const timespec& b ) { timespec t; t.tv_sec=a.tv_sec+b.tv_sec; t.tv_nsec=a.tv_nsec+b.tv_nsec;
const time_t sec=t.tv_nsec/1e+9; t.tv_sec+=sec; t.tv_nsec-=sec*1e+9;
return t; }
73 inline void timeDiff(
const timespec& start,
const timespec& end, timespec* result )
75 if ((end.tv_nsec-start.tv_nsec)<0) {
76 result->tv_sec = end.tv_sec-start.tv_sec-1;
77 result->tv_nsec = 1000000000+end.tv_nsec-start.tv_nsec;
79 result->tv_sec = end.tv_sec-start.tv_sec;
80 result->tv_nsec = end.tv_nsec-start.tv_nsec;
88 inline timespec
timeDiff(
const timespec& start,
const timespec& end )
104 inline int timeCmp(
const timespec& a,
const timespec& b )
106 if( a.tv_sec < b.tv_sec )
108 else if( a.tv_sec > b.tv_sec )
112 if( a.tv_nsec < b.tv_nsec )
114 else if( a.tv_nsec > b.tv_nsec )
137 inline uint64_t
apptime_nano() { timespec t;
apptime(&t);
return (uint64_t)t.tv_sec * uint64_t(1000000000) + (uint64_t)t.tv_nsec; }
143 inline float apptime() { timespec t;
apptime(&t);
return t.tv_sec + t.tv_nsec * 0.000000001f; }
149 inline float timeFloat(
const timespec& a ) {
return a.tv_sec * 1000.0f + a.tv_nsec * 0.000001f; }
155 inline double timeDouble(
const timespec& a ) {
return a.tv_sec * 1000.0 + a.tv_nsec * 0.000001; }
179 inline void sleepTime(
const timespec& duration ) { nanosleep(&duration, NULL); }
timespec timeAdd(const timespec &a, const timespec &b)
Add two times together.
Definition: timespec.h:67
double timeDouble(const timespec &a)
Convert to 64-bit double (in milliseconds).
Definition: timespec.h:155
#define LogInfo(format, args...)
Log a printf-style info message (Log::INFO)
Definition: logging.h:168
float timeFloat(const timespec &a)
Convert to 32-bit float (in milliseconds).
Definition: timespec.h:149
void timestamp(timespec *timestampOut)
Retrieve a timestamp of the current system time.
Definition: timespec.h:37
void timePrint(const timespec ×tamp, const char *text=NULL)
Print the time to stdout.
Definition: timespec.h:173
const timespec __apptime_begin__
timespec timeZero()
Return a blank timespec that's been zero'd.
Definition: timespec.h:49
uint64_t apptime_nano()
Retrieve the elapsed time since the process started (in nanoseconds).
Definition: timespec.h:137
void sleepNs(uint64_t nanoseconds)
Put the current thread to sleep for a specified number of nanoseconds.
Definition: timespec.h:203
timespec timeNew(time_t seconds, long int nanoseconds)
Return an initialized timespec
Definition: timespec.h:55
void sleepMs(uint64_t milliseconds)
Put the current thread to sleep for a specified number of milliseconds.
Definition: timespec.h:191
void apptime(timespec *a)
Retrieve the elapsed time since the process started.
Definition: timespec.h:131
int timeCmp(const timespec &a, const timespec &b)
Compare two timestamps.
Definition: timespec.h:104
char * timeStr(const timespec ×tamp, char *strOut)
Produce a text representation of the timestamp.
Definition: timespec.h:167
void sleepTime(const timespec &duration)
Put the current thread to sleep for a specified time.
Definition: timespec.h:179
void sleepUs(uint64_t microseconds)
Put the current thread to sleep for a specified number of microseconds.
Definition: timespec.h:197
void timeDiff(const timespec &start, const timespec &end, timespec *result)
Find the difference between two timestamps.
Definition: timespec.h:73