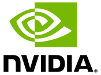 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __OPENGL_UTILITY_H
24 #define __OPENGL_UTILITY_H
38 #define LOG_GL "[OpenGL] "
44 #define GL(x) { x; glCheckError( #x, __FILE__, __LINE__ ); }
50 #define GL_VERIFY(x) { x; if(glCheckError( #x, __FILE__, __LINE__ )) return false; }
56 #define GL_VERIFYN(x) { x; if(glCheckError( #x, __FILE__, __LINE__ )) return NULL; }
62 #define GL_CHECK(msg) { glCheckError(msg, __FILE__, __LINE__); }
68 inline bool glCheckError(
const char* msg,
const char* file,
int line)
70 GLenum err = glGetError();
72 if( err == GL_NO_ERROR )
79 case GL_INVALID_ENUM: e =
"invalid enum";
break;
80 case GL_INVALID_VALUE: e =
"invalid value";
break;
81 case GL_INVALID_OPERATION: e =
"invalid operation";
break;
82 case GL_STACK_OVERFLOW: e =
"stack overflow";
break;
83 case GL_STACK_UNDERFLOW: e =
"stack underflow";
break;
84 case GL_OUT_OF_MEMORY: e =
"out of memory";
break;
85 #ifdef GL_TABLE_TOO_LARGE_EXT
86 case GL_TABLE_TOO_LARGE_EXT: e =
"table too large";
break;
88 #ifdef GL_TEXTURE_TOO_LARGE_EXT
89 case GL_TEXTURE_TOO_LARGE_EXT: e =
"texture too large";
break;
91 default: e =
"unknown error";
108 GLenum err = glGetError();
110 if( err == GL_NO_ERROR )
113 const char* e = NULL;
117 case GL_INVALID_ENUM: e =
"invalid enum";
break;
118 case GL_INVALID_VALUE: e =
"invalid value";
break;
119 case GL_INVALID_OPERATION: e =
"invalid operation";
break;
120 case GL_STACK_OVERFLOW: e =
"stack overflow";
break;
121 case GL_STACK_UNDERFLOW: e =
"stack underflow";
break;
122 case GL_OUT_OF_MEMORY: e =
"out of memory";
break;
123 #ifdef GL_TABLE_TOO_LARGE_EXT
124 case GL_TABLE_TOO_LARGE_EXT: e =
"table too large";
break;
126 #ifdef GL_TEXTURE_TOO_LARGE_EXT
127 case GL_TEXTURE_TOO_LARGE_EXT: e =
"texture too large";
break;
129 default: e =
"unknown error";
143 GLint total_mem_kb = 0;
144 GLint cur_avail_mem_kb = 0;
146 const GLenum GL_GPU_MEM_INFO_TOTAL_AVAILABLE_MEM_NVX = 0x9048;
147 const GLenum GL_GPU_MEM_INFO_CURRENT_AVAILABLE_MEM_NVX = 0x9049;
149 glGetIntegerv(GL_GPU_MEM_INFO_TOTAL_AVAILABLE_MEM_NVX, &total_mem_kb);
150 glGetIntegerv(GL_GPU_MEM_INFO_CURRENT_AVAILABLE_MEM_NVX,&cur_avail_mem_kb);
152 LogInfo(
LOG_GL "GPU memory free %i / %i kb\n", cur_avail_mem_kb, total_mem_kb);
161 inline void glDrawLine(
float x1,
float y1,
float x2,
float y2,
float r,
float g,
float b,
float a=1.0f,
float thickness=2.0f )
163 glLineWidth(thickness);
166 glColor4f(r, g, b, a);
180 inline void glDrawOutline(
float x,
float y,
float width,
float height,
float r,
float g,
float b,
float a=1.0f,
float thickness=2.0f )
182 const float right = x + width;
183 const float bottom = y + height;
185 glLineWidth(thickness);
186 glBegin(GL_LINE_LOOP);
188 glColor4f(r, g, b, a);
191 glVertex2f(right, y);
192 glVertex2f(right, bottom);
193 glVertex2f(x, bottom);
204 inline void glDrawRect(
float x,
float y,
float width,
float height,
float r,
float g,
float b,
float a=1.0f )
206 const float right = x + width;
207 const float bottom = y + height;
211 glColor4f(r, g, b, a);
214 glVertex2f(right, y);
215 glVertex2f(right, bottom);
216 glVertex2f(x, bottom);
#define LOG_GL
OpenGL logging prefix.
Definition: glUtility.h:38
#define LogInfo(format, args...)
Log a printf-style info message (Log::INFO)
Definition: logging.h:168
void glDrawRect(float x, float y, float width, float height, float r, float g, float b, float a=1.0f)
Render a filled rect in screen coordinates with the specified color.
Definition: glUtility.h:204
void glDrawLine(float x1, float y1, float x2, float y2, float r, float g, float b, float a=1.0f, float thickness=2.0f)
Render a line in screen coordinates with the specified color.
Definition: glUtility.h:161
void glDrawOutline(float x, float y, float width, float height, float r, float g, float b, float a=1.0f, float thickness=2.0f)
Render the outline of a rect in screen coordinates with the specified color.
Definition: glUtility.h:180
unsigned int uint
Definition: cudaMath.h:36
void glPrintFreeMem()
Print the amount of free GPU memory.
Definition: glUtility.h:141
#define LogError(format, args...)
Log a printf-style error message (Log::ERROR)
Definition: logging.h:150
bool glCheckError(const char *msg, const char *file, int line)
OpenGL error-checking messsage function.
Definition: glUtility.h:68