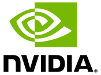 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GL_VIEWPORT_H__
24 #define __GL_VIEWPORT_H__
61 static glDisplay*
Create(
const char* title=NULL,
int width=-1,
int height=-1,
62 float r=0.05f,
float g=0.05f,
float b=0.05f,
float a=1.0f );
113 static const uint32_t
Type = (1 << 3);
153 void Render(
float* image, uint32_t width, uint32_t height,
float x=0.0f,
float y=30.0f,
bool normalize=
true );
160 template<
typename T>
bool Render( T* image, uint32_t width, uint32_t height ) {
return Render((
void**)image, width, height, imageFormatFromType<T>()); }
167 virtual bool Render(
void* image, uint32_t width, uint32_t height,
imageFormat format );
193 void RenderOnce(
float* image, uint32_t width, uint32_t height,
float x=5.0f,
float y=30.0f,
bool normalize=
true );
207 void RenderLine(
float x1,
float y1,
float x2,
float y2,
float r,
float g,
float b,
float a=1.0f,
float thickness=2.0f );
213 void RenderOutline(
float x,
float y,
float width,
float height,
float r,
float g,
float b,
float a=1.0f,
float thickness=2.0f );
219 void RenderRect(
float x,
float y,
float width,
float height,
float r,
float g,
float b,
float a=1.0f );
225 void RenderRect(
float r,
float g,
float b,
float a=1.0f );
245 void SetSize( uint32_t width, uint32_t height );
299 void SetViewport(
int left,
int top,
int right,
int bottom );
317 virtual void SetStatus(
const char* str );
526 bool GetDragRect(
int* x,
int* y,
int* width,
int* height );
554 void RemoveWidget( uint32_t index,
bool deleteWidget=
true );
584 std::vector<glWidget*>
FindWidgets(
int x,
int y );
599 static bool onEvent( uint16_t msg,
int a,
int b,
void* user );
int mMousePos[2]
Definition: glDisplay.h:632
void dispatchEvent(uint16_t msg, int a, int b)
GLXContext mContextGL
Definition: glDisplay.h:613
Definition: glDisplay.h:601
int GetWidgetIndex(const glWidget *widget) const
Retrieve the index of a widget (or -1 if not found)
void RenderImage(void *image, uint32_t width, uint32_t height, imageFormat format, float x=0.0f, float y=30.0f, bool normalize=true)
Render a CUDA image (uchar3, uchar4, float3, float4) using OpenGL interop.
void RenderOutline(float x, float y, float width, float height, float r, float g, float b, float a=1.0f, float thickness=2.0f)
Render the outline of a rect in screen coordinates with the specified color.
DragMode GetDragMode() const
Get the dragging behavior mode.
Definition: glDisplay.h:511
uint32_t glGetNumDisplays()
Return the number of created glDisplay windows.
static const char * DEFAULT_TITLE
Default title bar name.
Definition: glDisplay.h:329
void ResetCursor()
Reset the mouse cursor back to it's default.
void ProcessEvents()
Process UI event messages.
void Render(glTexture *texture, float x=5.0f, float y=30.0f)
Render an OpenGL texture.
XVisualInfo * mVisualX
Definition: glDisplay.h:611
void RenderOnce(void *image, uint32_t width, uint32_t height, imageFormat format, float x=5.0f, float y=30.0f, bool normalize=true)
Begin the frame, render one CUDA image using OpenGL interop, and end the frame.
void SetCursor(uint32_t cursor)
Set the active mouse cursor.
@ DragDisabled
Disable issuing all MOUSE_DRAG events.
Definition: glDisplay.h:503
void SetDefaultCursor(uint32_t cursor, bool activate=true)
Set the default mouse cursor that gets used by ResetCursor()
virtual void SetStatus(const char *str)
Set the window title string.
OpenGL display window and image/video renderer with CUDA interoperability.
Definition: glDisplay.h:47
int mDefaultCursor
Definition: glDisplay.h:616
uint32_t mNormalizedHeight
Definition: glDisplay.h:640
bool mKeyStates[1024]
Definition: glDisplay.h:636
std::vector< glTexture * > mTextures
Definition: glDisplay.h:643
glTexture * allocTexture(uint32_t width, uint32_t height, imageFormat format)
glDisplay(const videoOptions &options)
int mMouseDragOrigin[2]
Definition: glDisplay.h:634
void * user
Definition: glDisplay.h:604
void SetBackgroundColor(float r, float g, float b, float a=1.0f)
Set the window's background color.
bool mResizedToFeed
Definition: glDisplay.h:619
Window mWindowX
Definition: glDisplay.h:612
bool mMouseButtons[16]
Definition: glDisplay.h:635
void SetFullscreen(bool fullscreen)
Set the window to fullscreen mode or not.
uint32_t GetNumWidgets() const
Retrieve the number of widgets.
Definition: glDisplay.h:564
virtual uint32_t GetType() const
Return the interface type (glDisplay::Type)
Definition: glDisplay.h:108
bool mRendering
Definition: glDisplay.h:618
void SetDragMode(DragMode mode)
Set the dragging behavior mode.
Definition: glDisplay.h:516
bool mStreaming
Definition: videoOutput.h:321
void AddEventHandler(glEventHandler callback, void *user=NULL)
Register an event message handler that will be called by ProcessEvents()
uint32_t mID
Definition: glDisplay.h:623
void ResetDefaultCursor(bool activate=true)
Reset the default cursor back to it's original, the arrow.
#define KEY_OFFSET
Definition: glEvents.h:45
void SetTitle(const char *str)
Set the window title string.
static const int screenIdx
Definition: glDisplay.h:607
glWidget * GetWidget(const uint32_t index) const
Retrieve a widget.
Definition: glDisplay.h:569
void GetBackgroundColor(float *r, float *g, float *b, float *a=NULL)
Retrieve the window's background color.
void SetMaximized(bool maximized)
Maximize or un-maximize the window.
bool IsClosed() const
Returns true if the window has been closed.
Definition: glDisplay.h:88
int mActiveCursor
Definition: glDisplay.h:615
bool IsOpen() const
Returns true if the window is open.
Definition: glDisplay.h:83
std::vector< glWidget * > FindWidgets(int x, int y)
Find all widgets by coordinate, or NULL if no widget overlaps with that coordinate.
bool GetMouseButton(uint32_t button) const
Get the mouse button state.
Definition: glDisplay.h:402
const int * GetMousePosition() const
Get the mouse position.
Definition: glDisplay.h:380
bool IsDragging(DragMode mode=DragDefault) const
Is the mouse currently dragging in the specified mode?
Definition: glDisplay.h:521
uint32_t mNormalizedWidth
Definition: glDisplay.h:639
virtual bool Open()
Open the window.
static bool onEvent(uint16_t msg, int a, int b, void *user)
uint32_t GetID() const
Get the ID of this display instance into glGetDisplay()
Definition: glDisplay.h:103
static const uint32_t Type
Unique type identifier of glDisplay class.
Definition: glDisplay.h:113
bool IsRendering() const
Returns true if between BeginRender() and EndRender()
Definition: glDisplay.h:93
Atom mWindowClosedMsg
Definition: glDisplay.h:620
void SetViewport(int left, int top, int right, int bottom)
Set the active viewport being rendered to.
int mMouseDrag[2]
Definition: glDisplay.h:633
glEventHandler callback
Definition: glDisplay.h:603
void GetMousePosition(int *x, int *y) const
Get the mouse position.
Definition: glDisplay.h:385
void ResetViewport()
Reset to the full viewport (and change back GL_PROJECTION)
float * mNormalizedCUDA
Definition: glDisplay.h:638
bool(* glEventHandler)(uint16_t event, int a, int b, void *user)
Event message handler callback for recieving UI messages from a window.
Definition: glEvents.h:291
bool mInitialShow
Definition: glDisplay.h:617
Screen * mScreenX
Definition: glDisplay.h:610
int mViewport[4]
Definition: glDisplay.h:630
Cursor mCursors[256]
Definition: glDisplay.h:614
glDisplay * glGetDisplay(uint32_t display=0)
Retrieve a display window object.
void BeginRender(bool processEvents=true)
Clear window and begin rendering a frame.
@ DragCreate
Create a new widget from a dragging rectangle, and issue WIDGET_CREATED events.
Definition: glDisplay.h:505
glWidget * AddWidget(glWidget *widget)
Add a widget to the window that recieves events and is rendered.
~glDisplay()
Destroy window.
void RemoveEventHandler(glEventHandler callback, void *user=NULL)
Remove an event message handler from being called by ProcessEvents() RemoveEventHandler() will search...
OpenGL texture with CUDA interoperability.
Definition: glTexture.h:51
uint32_t mScreenWidth
Definition: glDisplay.h:624
uint32_t mScreenHeight
Definition: glDisplay.h:625
std::vector< glWidget * > mWidgets
Definition: glDisplay.h:642
void RemoveAllWidgets(bool deleteWidgets=true)
Remove all widgets from the window (and optionally delete them)
DragMode
Drag behavior enum.
Definition: glDisplay.h:500
float GetFPS() const
Get the average frame time (in milliseconds).
Definition: glDisplay.h:98
bool Render(T *image, uint32_t width, uint32_t height)
Render a CUDA image (uchar3, uchar4, float3, float4) using OpenGL interop.
Definition: glDisplay.h:160
void RenderRect(float x, float y, float width, float height, float r, float g, float b, float a=1.0f)
Render a filled rect in screen coordinates with the specified color.
float mBgColor[4]
Definition: glDisplay.h:629
DragMode mDragMode
Definition: glDisplay.h:621
The videoOutput API is for rendering and transmitting frames to video input devices such as display w...
Definition: videoOutput.h:104
static glDisplay * Create(const char *title=NULL, int width=-1, int height=-1, float r=0.05f, float g=0.05f, float b=0.05f, float a=1.0f)
Create a new OpenGL display window with the specified options.
bool IsFullscreen()
Determine if the window is fullscreen or not.
void RenderLine(float x1, float y1, float x2, float y2, float r, float g, float b, float a=1.0f, float thickness=2.0f)
Render a line in screen coordinates with the specified color.
@ DragDefault
Issue MOUSE_DRAG events, but nothing else.
Definition: glDisplay.h:502
bool GetDragCoords(int *x1, int *y1, int *x2, int *y2)
Get the current dragging coordinates, or return false if not dragging.
bool IsMaximized()
Determine if the window is maximized or not.
@ DragSelect
Select widgets from a dragging rectangle, and issue WIDGET_SELECTED events.
Definition: glDisplay.h:504
std::vector< eventHandler > mEventHandlers
Definition: glDisplay.h:644
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
void RemoveWidget(glWidget *widget, bool deleteWidget=true)
Remove a widget from the window (and optionally delete it)
bool GetDragRect(int *x, int *y, int *width, int *height)
Get the current dragging rectangle, or return false if not dragging.
__host__ __device__ float2 normalize(float2 v)
Definition: cudaMath.h:1580
bool GetKey(uint32_t key) const
Get the state of a key (lowercase, without modifiers applied)
Definition: glDisplay.h:425
Display * mDisplayX
Definition: glDisplay.h:609
void SetSize(uint32_t width, uint32_t height)
Set the window's size.
glWidget * FindWidget(int x, int y)
Find first widget by coordinate, or NULL if no widget overlaps with that coordinate.
timespec mLastTime
Definition: glDisplay.h:627
void EndRender()
Finish rendering and refresh / flip the backbuffer.
float mAvgTime
Definition: glDisplay.h:628