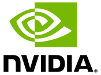 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __DETECT_NET_H__
24 #define __DETECT_NET_H__
34 #define DETECTNET_DEFAULT_INPUT "data"
40 #define DETECTNET_DEFAULT_COVERAGE "coverage"
46 #define DETECTNET_DEFAULT_BBOX "bboxes"
52 #define DETECTNET_DEFAULT_CONFIDENCE_THRESHOLD 0.5f
58 #define DETECTNET_DEFAULT_CLUSTERING_THRESHOLD 0.75f
65 #define DETECTNET_DEFAULT_THRESHOLD DETECTNET_DEFAULT_CONFIDENCE_THRESHOLD
71 #define DETECTNET_DEFAULT_ALPHA 120
77 #define DETECTNET_MODEL_TYPE "detection"
83 #define DETECTNET_USAGE_STRING "detectNet arguments: \n" \
84 " --network=NETWORK pre-trained model to load, one of the following:\n" \
85 " * ssd-mobilenet-v1\n" \
86 " * ssd-mobilenet-v2 (default)\n" \
87 " * ssd-inception-v2\n" \
89 " * peoplenet-pruned\n" \
91 " * trafficcamnet\n" \
93 " --model=MODEL path to custom model to load (caffemodel, uff, or onnx)\n" \
94 " --prototxt=PROTOTXT path to custom prototxt to load (for .caffemodel only)\n" \
95 " --labels=LABELS path to text file containing the labels for each class\n" \
96 " --input-blob=INPUT name of the input layer (default is '" DETECTNET_DEFAULT_INPUT "')\n" \
97 " --output-cvg=COVERAGE name of the coverage/confidence output layer (default is '" DETECTNET_DEFAULT_COVERAGE "')\n" \
98 " --output-bbox=BOXES name of the bounding output layer (default is '" DETECTNET_DEFAULT_BBOX "')\n" \
99 " --mean-pixel=PIXEL mean pixel value to subtract from input (default is 0.0)\n" \
100 " --confidence=CONF minimum confidence threshold for detection (default is 0.5)\n" \
101 " --clustering=CLUSTER minimum overlapping area threshold for clustering (default is 0.75)\n" \
102 " --alpha=ALPHA overlay alpha blending value, range 0-255 (default: 120)\n" \
103 " --overlay=OVERLAY detection overlay flags (e.g. --overlay=box,labels,conf)\n" \
104 " valid combinations are: 'box', 'lines', 'labels', 'conf', 'none'\n" \
105 " --profile enable layer profiling in TensorRT\n\n" \
141 inline float Width()
const {
return Right -
Left; }
144 inline float Height()
const {
return Bottom -
Top; }
147 inline float Area()
const {
return Width() * Height(); }
150 static inline float Width(
float x1,
float x2 ) {
return x2 - x1; }
153 static inline float Height(
float y1,
float y2 ) {
return y2 - y1; }
156 static inline float Area(
float x1,
float y1,
float x2,
float y2 ) {
return Width(x1,x2) * Height(y1,y2); }
159 inline void Center(
float* x,
float* y )
const {
if(x) *x =
Left + Width() * 0.5f;
if(y) *y =
Top + Height() * 0.5f; }
162 inline bool Contains(
float x,
float y )
const {
return x >=
Left && x <= Right && y >=
Top && y <=
Bottom; }
165 inline bool Intersects(
const Detection& det,
float areaThreshold=0.0f )
const {
return (IntersectionArea(det) /
fmaxf(Area(), det.Area()) > areaThreshold); }
168 inline bool Intersects(
float x1,
float y1,
float x2,
float y2,
float areaThreshold=0.0f )
const {
return (IntersectionArea(x1,y1,x2,y2) /
fmaxf(Area(), Area(x1,y1,x2,y2)) > areaThreshold); }
171 inline float IntersectionArea(
const Detection& det )
const {
return IntersectionArea(det.Left, det.Top, det.Right, det.Bottom); }
174 inline float IntersectionArea(
float x1,
float y1,
float x2,
float y2 )
const {
if(!Overlaps(x1,y1,x2,y2))
return 0.0f;
return (
fminf(
Right, x2) -
fmaxf(
Left, x1)) * (
fminf(
Bottom, y2) -
fmaxf(
Top, y1)); }
177 inline float IOU(
const Detection& det )
const {
return IOU(det.Left, det.Top, det.Right, det.Bottom); }
180 inline float IOU(
float x1,
float y1,
float x2,
float y2 )
const;
183 inline bool Overlaps(
const Detection& det )
const {
return !(det.Left >
Right || det.Right <
Left || det.Top >
Bottom || det.Bottom <
Top); }
186 inline bool Overlaps(
float x1,
float y1,
float x2,
float y2 )
const {
return !(x1 >
Right || x2 < Left || y1 >
Bottom || y2 <
Top); }
189 inline bool Expand(
float x1,
float y1,
float x2,
float y2 ) {
if(!Overlaps(x1, y1, x2, y2))
return false;
Left =
fminf(x1,
Left);
Top =
fminf(y1,
Top);
Right =
fmaxf(x2,
Right);
Bottom =
fmaxf(y2,
Bottom);
return true; }
245 static detectNet*
Create(
const char* prototxt_path,
const char* model_path,
float mean_pixel=0.0f,
267 static detectNet*
Create(
const char* prototxt_path,
const char* model_path,
float mean_pixel,
268 const char* class_labels,
const char* class_colors,
288 static detectNet*
Create(
const char* model_path,
const char* class_labels,
float threshold,
289 const char* input,
const Dims3& inputDims,
290 const char* output,
const char* numDetections,
324 template<
typename T>
int Detect( T* image, uint32_t width, uint32_t height,
Detection** detections, uint32_t overlay=
OVERLAY_DEFAULT ) {
return Detect((
void*)image, width, height, imageFormatFromType<T>(), detections, overlay); }
336 template<
typename T>
int Detect( T* image, uint32_t width, uint32_t height,
Detection* detections, uint32_t overlay=
OVERLAY_DEFAULT ) {
return Detect((
void*)image, width, height, imageFormatFromType<T>(), detections, overlay); }
371 int Detect(
float* input, uint32_t width, uint32_t height, Detection** detections, uint32_t overlay=
OVERLAY_DEFAULT );
384 int Detect(
float* input, uint32_t width, uint32_t height, Detection* detections, uint32_t overlay=
OVERLAY_DEFAULT );
393 template<
typename T>
bool Overlay( T* input, T* output, uint32_t width, uint32_t height,
Detection* detections, uint32_t numDetections, uint32_t flags=
OVERLAY_DEFAULT ) {
return Overlay(input, output, width, height, imageFormatFromType<T>(), detections, numDetections, flags); }
402 bool Overlay(
void* input,
void* output, uint32_t width, uint32_t height,
imageFormat format, Detection* detections, uint32_t numDetections, uint32_t flags=
OVERLAY_DEFAULT );
465 inline const char*
GetClassDesc( uint32_t index )
const {
if(index >=
mClassDesc.size()) { printf(
"invalid class %u\n", index);
return "Invalid"; }
return mClassDesc[index].c_str(); }
522 bool init(
const char* prototxt_path,
const char* model_path,
const char* class_labels,
const char* class_colors,
523 float threshold,
const char* input,
const char* coverage,
const char* bboxes, uint32_t maxBatchSize,
527 int postProcess(
void* input, uint32_t width, uint32_t height,
imageFormat format, Detection* detections );
565 const float overlap_x0 =
fmaxf(
Left, x1);
566 const float overlap_y0 =
fmaxf(
Top, y1);
571 if( (overlap_x1 - overlap_x0 <= 0) || (overlap_y1 - overlap_y0 <= 0) )
575 const float size_1 = Area();
576 const float size_2 = Area(x1, y1, x2, y2);
578 const float size_intersection = (overlap_x1 - overlap_x0) * (overlap_y1 - overlap_y0);
579 const float size_union = size_1 + size_2 - size_intersection;
581 return size_intersection / size_union;
const char * GetClassPath() const
Retrieve the path to the file containing the class descriptions.
Definition: detectNet.h:475
float Confidence
Confidence value of the detected object.
Definition: detectNet.h:126
@ OVERLAY_NONE
No overlay.
Definition: detectNet.h:206
float Left
Left bounding box coordinate (in pixels)
Definition: detectNet.h:135
void SetConfidenceThreshold(float threshold)
Set the minimum threshold for detection.
Definition: detectNet.h:424
@ OVERLAY_DEFAULT
The default choice of overlay.
Definition: detectNet.h:212
int postProcessDetectNet(Detection *detections, uint32_t width, uint32_t height)
float GetOverlayAlpha() const
Retrieve the overlay alpha blending value for classes that don't have it explicitly set (between 0-25...
Definition: detectNet.h:505
#define DETECTNET_DEFAULT_COVERAGE
Name of default output blob of the coverage map for DetectNet caffe model.
Definition: detectNet.h:40
int TrackStatus
-1 for dropped, 0 for initializing, 1 for active/valid
Definition: detectNet.h:130
float IOU(float x1, float y1, float x2, float y2) const
Return true if the bounding boxes overlap.
Definition: detectNet.h:563
float GetThreshold() const
Retrieve the minimum threshold for detection.
Definition: detectNet.h:408
float GetConfidenceThreshold() const
Retrieve the minimum threshold for detection.
Definition: detectNet.h:419
bool preProcess(void *input, uint32_t width, uint32_t height, imageFormat format)
uchar3 color
The RGB color of the point.
Definition: cudaPointCloud.h:11
void SetClusteringThreshold(float threshold)
Set the overlapping area % threshold for clustering.
Definition: detectNet.h:434
std::string mClassPath
Definition: detectNet.h:551
Detection()
Definition: detectNet.h:198
float mClusteringThreshold
Definition: detectNet.h:540
@ OVERLAY_CONFIDENCE
Overlay the detection confidence values.
Definition: detectNet.h:209
int Detect(T *image, uint32_t width, uint32_t height, Detection *detections, uint32_t overlay=OVERLAY_DEFAULT)
Detect object locations in an image, into an array of the results allocated by the user.
Definition: detectNet.h:336
#define DETECTNET_DEFAULT_INPUT
Name of default input blob for DetectNet caffe model.
Definition: detectNet.h:34
Object recognition and localization networks with TensorRT support.
Definition: detectNet.h:116
objectTracker * mTracker
Definition: detectNet.h:537
void sortDetections(Detection *detections, int numDetections)
Object Detection result.
Definition: detectNet.h:122
float mMeanPixel
Definition: detectNet.h:542
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
uint32_t mNumClasses
Definition: detectNet.h:552
float4 * mClassColors
Definition: detectNet.h:546
const char * GetClassLabel(uint32_t index) const
Retrieve the description of a particular class.
Definition: detectNet.h:460
nvinfer1::Dims3 Dims3
Definition: tensorNet.h:58
static uint32_t OverlayFlagsFromStr(const char *flags)
Parse a string sequence into OverlayFlags enum.
void SetClassColor(uint32_t classIndex, float r, float g, float b, float a=255.0f)
Set the visualization color of a particular class of object.
Definition: detectNet.h:490
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
uint32_t ClassID
Class index of the detected object.
Definition: detectNet.h:125
void SetLineWidth(float width)
Set the line width used during overlay when OVERLAY_LINES is used.
Definition: detectNet.h:500
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
void SetClassColor(uint32_t classIndex, const float4 &color)
Set the visualization color of a particular class of object.
Definition: detectNet.h:485
int postProcess(void *input, uint32_t width, uint32_t height, imageFormat format, Detection *detections)
int clusterDetections(Detection *detections, int n)
OverlayFlags
Overlay flags (can be OR'd together).
Definition: detectNet.h:204
float fminf(float a, float b)
Definition: cudaMath.h:51
bool loadClassColors(const char *filename)
void SetOverlayAlpha(float alpha)
Set overlay alpha blending value for all classes (between 0-255).
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
std::vector< std::string > mClassSynset
Definition: detectNet.h:549
float mOverlayAlpha
Definition: detectNet.h:544
virtual ~detectNet()
Destory.
uint32_t mDetectionSet
Definition: detectNet.h:555
Object tracker interface.
Definition: objectTracker.h:52
objectTracker * GetTracker() const
Get the object tracker being used.
Definition: detectNet.h:439
int postProcessSSD_UFF(Detection *detections, uint32_t width, uint32_t height)
uint32_t GetMaxDetections() const
Retrieve the maximum number of simultaneous detections the network supports.
Definition: detectNet.h:450
@ OVERLAY_LABEL
Overlay the class description labels.
Definition: detectNet.h:208
int TrackLost
The number of consecutive frames tracking has been lost for.
Definition: detectNet.h:132
const char * GetClassDesc(uint32_t index) const
Retrieve the description of a particular class.
Definition: detectNet.h:465
static detectNet * Create(const char *network="ssd-mobilenet-v2", float threshold=DETECTNET_DEFAULT_CONFIDENCE_THRESHOLD, uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load a pre-trained model.
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
bool init(const char *prototxt_path, const char *model_path, const char *class_labels, const char *class_colors, float threshold, const char *input, const char *coverage, const char *bboxes, uint32_t maxBatchSize, precisionType precision, deviceType device, bool allowGPUFallback)
static const uint32_t mNumDetectionSets
Definition: detectNet.h:558
@ OVERLAY_BOX
Overlay the object bounding boxes (filled)
Definition: detectNet.h:207
void SetThreshold(float threshold)
Set the minimum threshold for detection.
Definition: detectNet.h:414
float mConfidenceThreshold
Definition: detectNet.h:539
int TrackID
Unique tracking ID (or -1 if untracked)
Definition: detectNet.h:129
__host__ __device__ float4 make_float4(float s)
Definition: cudaMath.h:248
float Right
Right bounding box coordinate (in pixels)
Definition: detectNet.h:136
Detection * mDetectionSets
Definition: detectNet.h:554
bool Overlay(T *input, T *output, uint32_t width, uint32_t height, Detection *detections, uint32_t numDetections, uint32_t flags=OVERLAY_DEFAULT)
Draw the detected bounding boxes overlayed on an RGBA image.
Definition: detectNet.h:393
bool loadClassInfo(const char *filename)
uint32_t mMaxDetections
Definition: detectNet.h:556
uint32_t GetNumClasses() const
Retrieve the number of object classes supported in the detector.
Definition: detectNet.h:455
float Bottom
Bottom bounding box coordinate (in pixels)
Definition: detectNet.h:138
#define DETECTNET_DEFAULT_CONFIDENCE_THRESHOLD
Default value of the minimum detection threshold.
Definition: detectNet.h:52
float4 GetClassColor(uint32_t classIndex) const
Retrieve the RGBA visualization color a particular class.
Definition: detectNet.h:480
std::vector< std::string > mClassDesc
Definition: detectNet.h:548
@ OVERLAY_TRACKING
Overlay tracking information (like track ID)
Definition: detectNet.h:210
float GetLineWidth() const
Retrieve the line width used during overlay when OVERLAY_LINES is used.
Definition: detectNet.h:495
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
detectNet(float meanPixel=0.0f)
const char * GetClassSynset(uint32_t index) const
Retrieve the class synset category of a particular class.
Definition: detectNet.h:470
int TrackFrames
The number of frames the object has been re-identified for.
Definition: detectNet.h:131
int Detect(T *image, uint32_t width, uint32_t height, Detection **detections, uint32_t overlay=OVERLAY_DEFAULT)
Detect object locations from an image, returning an array containing the detection results.
Definition: detectNet.h:324
void SetTracker(objectTracker *tracker)
Set the object tracker to be used.
Definition: detectNet.h:444
float Top
Top bounding box cooridnate (in pixels)
Definition: detectNet.h:137
float mLineWidth
Definition: detectNet.h:543
float fmaxf(float a, float b)
Definition: cudaMath.h:56
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
static const char * Usage()
Usage string for command line arguments to Create()
Definition: detectNet.h:308
#define DETECTNET_DEFAULT_BBOX
Name of default output blob of the grid of bounding boxes for DetectNet caffe model.
Definition: detectNet.h:46
@ OVERLAY_LINES
Overlay the bounding box lines (unfilled)
Definition: detectNet.h:211
int postProcessDetectNet_v2(Detection *detections, uint32_t width, uint32_t height)
#define DETECTNET_USAGE_STRING
Standard command-line options able to be passed to detectNet::Create()
Definition: detectNet.h:83
float GetClusteringThreshold() const
Retrieve the overlapping area % threshold for clustering.
Definition: detectNet.h:429
__device__ cudaVectorTypeInfo< T >::Base alpha(T vec, typename cudaVectorTypeInfo< T >::Base default_alpha=255)
Definition: cudaVector.h:98
int postProcessSSD_ONNX(Detection *detections, uint32_t width, uint32_t height)