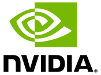 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CUDA_RGB_CONVERT_H
24 #define __CUDA_RGB_CONVERT_H
44 cudaError_t
cudaRGB8ToBGR8( uchar3* input, uchar3* output,
size_t width,
size_t height );
51 cudaError_t
cudaRGB32ToBGR32( float3* input, float3* output,
size_t width,
size_t height );
58 cudaError_t
cudaRGBA8ToBGRA8( uchar4* input, uchar4* output,
size_t width,
size_t height );
65 cudaError_t
cudaRGBA32ToBGRA32( float4* input, float4* output,
size_t width,
size_t height );
85 cudaError_t
cudaRGB8ToRGBA8( uchar3* input, uchar4* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
94 cudaError_t
cudaRGBA8ToRGB8( uchar4* input, uchar3* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
114 cudaError_t
cudaRGB32ToRGBA32( float3* input, float4* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
123 cudaError_t
cudaRGBA32ToRGB32( float4* input, float3* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
143 cudaError_t
cudaRGB8ToRGB32( uchar3* input, float3* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
152 cudaError_t
cudaRGB8ToRGBA32( uchar3* input, float4* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
161 cudaError_t
cudaRGBA8ToRGB32( uchar4* input, float3* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
170 cudaError_t
cudaRGBA8ToRGBA32( uchar4* input, float4* output,
size_t width,
size_t height,
bool swapRedBlue=
false );
195 cudaError_t
cudaRGB32ToRGB8( float3* input, uchar3* output,
size_t width,
size_t height,
196 bool swapRedBlue=
false,
const float2& pixelRange=
make_float2(0,255) );
210 cudaError_t
cudaRGB32ToRGBA8( float3* input, uchar4* output,
size_t width,
size_t height,
211 bool swapRedBlue=
false,
const float2& pixelRange=
make_float2(0,255) );
225 cudaError_t
cudaRGBA32ToRGB8( float4* input, uchar3* output,
size_t width,
size_t height,
226 bool swapRedBlue=
false,
const float2& pixelRange=
make_float2(0,255) );
240 cudaError_t
cudaRGBA32ToRGBA8( float4* input, uchar4* output,
size_t width,
size_t height,
241 bool swapRedBlue=
false,
const float2& pixelRange=
make_float2(0,255) );
cudaError_t cudaRGBA8ToRGBA32(uchar4 *input, float4 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar4 RGBA/BGRA image to float4 RGBA/BGRA image.
cudaError_t cudaRGBA8ToRGB32(uchar4 *input, float3 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar4 RGBA/BGRA image to float3 RGB/BGR image.
cudaError_t cudaRGBA32ToRGB32(float4 *input, float3 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert float4 RGBA/BGRA image into float3 RGB/BGR image.
cudaError_t cudaRGBA32ToRGB8(float4 *input, uchar3 *output, size_t width, size_t height, bool swapRedBlue=false, const float2 &pixelRange=make_float2(0, 255))
Convert float4 RGBA/BGRA image into uchar3 image.
cudaError_t cudaRGBA8ToRGB8(uchar4 *input, uchar3 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar4 RGBA/BGRA image to uchar3 RGB/BGR image.
cudaError_t cudaRGBA32ToRGBA8(float4 *input, uchar4 *output, size_t width, size_t height, bool swapRedBlue=false, const float2 &pixelRange=make_float2(0, 255))
Convert float4 RGBA/BGRA image into uchar4 RGBA/BGRA image.
cudaError_t cudaRGB32ToBGR32(float3 *input, float3 *output, size_t width, size_t height)
Convert float3 RGB image to float3 BGR (or convert BGR to RGB).
cudaError_t cudaRGB8ToRGBA8(uchar3 *input, uchar4 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar3 RGB/BGR image to uchar4 RGBA/BGRA image.
cudaError_t cudaRGB8ToRGB32(uchar3 *input, float3 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar3 RGB/BGR image to float3 RGB/BGR image.
cudaError_t cudaRGBA32ToBGRA32(float4 *input, float4 *output, size_t width, size_t height)
Convert float4 RGBA image to float4 BGRA (or convert BGRA to RGBA).
cudaError_t cudaRGB8ToRGBA32(uchar3 *input, float4 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert uchar3 RGB/BGR image to float4 RGBA/BGRA image.
cudaError_t cudaRGB32ToRGBA32(float3 *input, float4 *output, size_t width, size_t height, bool swapRedBlue=false)
Convert float3 RGB/BGR image into float4 RGBA/BGRA image.
cudaError_t cudaRGB32ToRGBA8(float3 *input, uchar4 *output, size_t width, size_t height, bool swapRedBlue=false, const float2 &pixelRange=make_float2(0, 255))
Convert float3 RGB/BGR image into uchar4 RGBA/BGRA image.
__host__ __device__ float2 make_float2(float s)
Definition: cudaMath.h:81
cudaError_t cudaRGB8ToBGR8(uchar3 *input, uchar3 *output, size_t width, size_t height)
Convert uchar3 RGB image to uchar3 BGR (or convert BGR to RGB).
cudaError_t cudaRGB32ToRGB8(float3 *input, uchar3 *output, size_t width, size_t height, bool swapRedBlue=false, const float2 &pixelRange=make_float2(0, 255))
Convert float3 RGB/BGR image into uchar3 RGB/BGR image.
cudaError_t cudaRGBA8ToBGRA8(uchar4 *input, uchar4 *output, size_t width, size_t height)
Convert uchar4 RGBA image to uchar4 BGRA (or convert BGRA to RGBA).