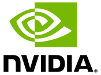 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __WEBRTC_SERVER_H__
24 #define __WEBRTC_SERVER_H__
26 #include <libsoup/soup.h>
42 #define WEBRTC_DEFAULT_PORT 41567
49 #define WEBRTC_DEFAULT_STUN_SERVER "stun.l.google.com:19302"
55 #define LOG_WEBRTC "[webrtc] "
125 const char* ssl_cert=NULL,
const char* ssl_key=NULL,
126 bool threaded=
true );
153 void AddRoute(
const char* path,
HttpListener callback,
void* user_data=NULL, uint32_t flags=0 );
200 WebRTCServer( uint16_t port,
const char* stun_server,
const char* ssl_cert_file,
const char* ssl_key_file,
bool threaded );
205 static void*
runThread(
void* user_data );
207 static void onHttpRequest( SoupServer* soup_server, SoupMessage* message,
const char* path, GHashTable* query, SoupClientContext* client_context,
void* user_data );
208 static void onHttpDefault( SoupServer* soup_server, SoupMessage* message,
const char* path, GHashTable* query, SoupClientContext* client_context,
void* user_data );
210 static void onWebsocketOpened( SoupServer* server, SoupWebsocketConnection* connection,
const char *path, SoupClientContext* client_context,
void* user_data );
211 static void onWebsocketMessage( SoupWebsocketConnection* connection, SoupWebsocketDataType data_type, GBytes* message,
void* user_data );
212 static void onWebsocketClosed( SoupWebsocketConnection* connection,
void* user_data );
const char * GetSTUNServer() const
Get the STUN server being used.
Definition: WebRTCServer.h:177
std::string printRouteInfo(WebsocketRoute *route) const
WebRTCServer * server
Definition: WebRTCServer.h:89
std::string mStunServer
Definition: WebRTCServer.h:244
std::string mSSLKeyFile
Definition: WebRTCServer.h:247
uint32_t mPeerCount
Definition: WebRTCServer.h:253
std::string path
Definition: WebRTCServer.h:216
void AddRoute(const char *path, HttpListener callback, void *user_data=NULL, uint32_t flags=0)
Register a function to handle incoming http/www requests at the specified path.
void * user_data
Definition: WebRTCServer.h:97
void(* WebsocketListener)(WebRTCPeer *peer, const char *message, size_t message_size, void *user_data)
Function pointer to a callback for handling websocket requests.
Definition: WebRTCServer.h:138
std::string ip_address
Definition: WebRTCServer.h:95
uint32_t mRefCount
Definition: WebRTCServer.h:252
WebRTC signalling server for establishing and negotiating connections with peers for bi-directional m...
Definition: WebRTCServer.h:116
SoupClientContext * client_context
Definition: WebRTCServer.h:88
std::string path
Definition: WebRTCServer.h:94
static void onHttpRequest(SoupServer *soup_server, SoupMessage *message, const char *path, GHashTable *query, SoupClientContext *client_context, void *user_data)
static void onHttpDefault(SoupServer *soup_server, SoupMessage *message, const char *path, GHashTable *query, SoupClientContext *client_context, void *user_data)
uint32_t ID
Definition: WebRTCServer.h:91
uint32_t flags
Definition: WebRTCServer.h:219
@ WEBRTC_PUBLIC
Definition: WebRTCServer.h:66
uint32_t flags
Definition: WebRTCServer.h:92
@ WEBRTC_PRIVATE
Definition: WebRTCServer.h:65
@ WEBRTC_PEER_CLOSED
Definition: WebRTCServer.h:77
WebsocketRoute * findWebsocketRoute(const char *path) const
WebRTCFlags
Flags for route decorators or peer state.
Definition: WebRTCServer.h:62
@ WEBRTC_PEER_STREAMING
Definition: WebRTCServer.h:76
uint16_t mPort
Definition: WebRTCServer.h:251
void Release()
Release a reference to the server instance.
@ WEBRTC_MULTI_CLIENT
Definition: WebRTCServer.h:71
Definition: WebRTCServer.h:214
@ WEBRTC_PEER_CONNECTING
Definition: WebRTCServer.h:74
@ WEBRTC_RECEIVE
Definition: WebRTCServer.h:70
std::vector< WebRTCPeer * > peers
Definition: WebRTCServer.h:228
@ WEBRTC_SEND
Definition: WebRTCServer.h:69
SoupServerCallback HttpListener
Function pointer to a callback for handling HTTP requests.
Definition: WebRTCServer.h:144
SoupWebsocketConnection * connection
Definition: WebRTCServer.h:87
bool mHasHTTPS
Definition: WebRTCServer.h:249
bool IsThreaded() const
Return true if the server is running in it's own thread.
Definition: WebRTCServer.h:189
Definition: WebRTCServer.h:222
std::string path
Definition: WebRTCServer.h:224
@ WEBRTC_AUDIO
Definition: WebRTCServer.h:67
SoupServer * mSoupServer
Definition: WebRTCServer.h:243
bool HasHTTPS() const
Return true if the server is using HTTPS.
Definition: WebRTCServer.h:183
HttpRoute * findHttpRoute(const char *path) const
HttpListener callback
Definition: WebRTCServer.h:217
static WebRTCServer * Create(uint16_t port=WEBRTC_DEFAULT_PORT, const char *stun_server=WEBRTC_DEFAULT_STUN_SERVER, const char *ssl_cert=NULL, const char *ssl_key=NULL, bool threaded=true)
Create a WebRTC server on this port.
Thread * mThread
Definition: WebRTCServer.h:255
std::vector< HttpRoute * > mHttpRoutes
Definition: WebRTCServer.h:240
void freeRoute(HttpRoute *route)
bool ProcessRequests(bool blocking=false)
Process incoming requests on the server.
std::string mSSLCertFile
Definition: WebRTCServer.h:246
@ WEBRTC_VIDEO
Definition: WebRTCServer.h:68
@ WEBRTC_PEER_CONNECTED
Definition: WebRTCServer.h:75
static void * runThread(void *user_data)
#define WEBRTC_DEFAULT_PORT
Default HTTP/websocket port used by the WebRTC server.
Definition: WebRTCServer.h:42
uint32_t flags
Definition: WebRTCServer.h:227
static void onWebsocketClosed(SoupWebsocketConnection *connection, void *user_data)
static void onWebsocketMessage(SoupWebsocketConnection *connection, SoupWebsocketDataType data_type, GBytes *message, void *user_data)
Thread class for launching an asynchronous operating-system dependent thread.
Definition: Thread.h:44
WebsocketListener callback
Definition: WebRTCServer.h:225
std::vector< WebsocketRoute * > mWebsocketRoutes
Definition: WebRTCServer.h:241
Remote peer that has connected.
Definition: WebRTCServer.h:85
WebRTCServer(uint16_t port, const char *stun_server, const char *ssl_cert_file, const char *ssl_key_file, bool threaded)
#define WEBRTC_DEFAULT_STUN_SERVER
Default STUN server used for WebRTC.
Definition: WebRTCServer.h:49
void * user_data
Definition: WebRTCServer.h:218
void * user_data
Definition: WebRTCServer.h:226
static void onWebsocketOpened(SoupServer *server, SoupWebsocketConnection *connection, const char *path, SoupClientContext *client_context, void *user_data)