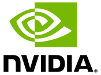 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __MULTITHREAD_H_
24 #define __MULTITHREAD_H_
35 typedef void* (*ThreadEntryFunction)(
void* user_param );
78 void Stop(
bool wait=
false );
105 static int SetPriority(
int priority, pthread_t* thread=NULL );
121 static void Yield(
unsigned int ms );
138 static bool SetAffinity(
unsigned int cpu, pthread_t* thread=NULL );
int GetPriorityLevel()
Get this thread's priority level.
static int GetCPU()
Look up which CPU core the thread is running on.
static void Yield(unsigned int ms)
Whatever thread you are calling from, yield the processor for the specified number of milliseconds.
bool SetPriorityLevel(int priority)
Set this thread's priority level.
bool LockAffinity(unsigned int cpu)
Lock this thread to a CPU core.
bool Start()
Start the thread.
static int GetMinPriority()
Get the minimum priority level avaiable.
pthread_t * GetThreadID()
Get thread identififer.
Definition: Thread.h:126
static int GetPriority(pthread_t *thread=NULL)
Get the priority level of the thread.
static bool SetAffinity(unsigned int cpu, pthread_t *thread=NULL)
Lock the specified thread's affinity to a CPU core.
static void * DefaultEntry(void *param)
pthread_t mThreadID
Definition: Thread.h:149
virtual ~Thread()
Destructor.
bool mThreadStarted
Definition: Thread.h:150
void Stop(bool wait=false)
Signal for the thread to stop running.
static void InitRealtime()
Prime the system for realtime use.
static int GetMaxPriority()
Get the maximum priority level available.
Thread()
Default constructor.
Thread class for launching an asynchronous operating-system dependent thread.
Definition: Thread.h:44
static int SetPriority(int priority, pthread_t *thread=NULL)
Set the priority level of the thread.
virtual void Run()
User-implemented thread main() function.
void *(* ThreadEntryFunction)(void *user_param)
Function pointer typedef representing a thread's main entry point.
Definition: Thread.h:35