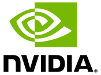 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __VIDEO_SOURCE_H_
24 #define __VIDEO_SOURCE_H_
36 #define VIDEO_SOURCE_USAGE_STRING "videoSource arguments: \n" \
37 " input resource URI of the input stream, for example:\n" \
38 " * /dev/video0 (V4L2 camera #0)\n" \
39 " * csi://0 (MIPI CSI camera #0)\n" \
40 " * rtp://@:1234 (RTP stream)\n" \
41 " * rtsp://user:pass@ip:1234 (RTSP stream)\n" \
42 " * webrtc://@:1234/my_stream (WebRTC stream)\n" \
43 " * file://my_image.jpg (image file)\n" \
44 " * file://my_video.mp4 (video file)\n" \
45 " * file://my_directory/ (directory of images)\n" \
46 " --input-width=WIDTH explicitly request a width of the stream (optional)\n" \
47 " --input-height=HEIGHT explicitly request a height of the stream (optional)\n" \
48 " --input-rate=RATE explicitly request a framerate of the stream (optional)\n" \
49 " --input-save=FILE path to video file for saving the input stream to disk\n" \
50 " --input-codec=CODEC RTP requires the codec to be set, one of these:\n" \
55 " --input-decoder=TYPE the decoder engine to use, one of these:\n" \
57 " * omx (aarch64/JetPack4 only)\n" \
58 " * v4l2 (aarch64/JetPack5 only)\n" \
59 " --input-flip=FLIP flip method to apply to input:\n" \
60 " * none (default)\n" \
61 " * counterclockwise\n" \
66 " * upper-right-diagonal\n" \
67 " * upper-left-diagonal\n" \
68 " --input-loop=LOOP for file-based inputs, the number of loops to run:\n" \
69 " * -1 = loop forever\n" \
70 " * 0 = don't loop (default)\n" \
71 " * >0 = set number of loops\n\n"
240 template<
typename T>
bool Capture( T** image, uint64_t timeout=
DEFAULT_TIMEOUT,
int* status=NULL ) {
return Capture((
void**)image, imageFormatFromType<T>(), timeout); }
283 virtual void Close();
341 virtual inline uint32_t
GetType()
const {
return 0; }
366 static const char*
TypeToStr( uint32_t type );
virtual bool Open()
Begin streaming the device.
virtual void Close()
Stop streaming the device.
bool IsType() const
Check if a this stream is of a particular type.
Definition: videoSource.h:356
@ EOS
end-of-stream (EOS)
Definition: videoSource.h:127
static const char * Usage()
Usage string for command line arguments to Create()
Definition: videoSource.h:186
uint32_t GetWidth() const
Return the width of the stream, in pixels.
Definition: videoSource.h:296
const URI & GetResource() const
Return the resource URI of the stream.
Definition: videoSource.h:326
bool Capture(T **image, uint64_t timeout=DEFAULT_TIMEOUT, int *status=NULL)
Capture the next image from the video stream.
Definition: videoSource.h:240
videoSource(const videoOptions &options)
@ TIMEOUT
a timeout occurred
Definition: videoSource.h:128
bool mStreaming
Definition: videoSource.h:377
bool IsType(uint32_t type) const
Check if this stream is of a particular type.
Definition: videoSource.h:347
bool IsStreaming() const
Check if the device is actively streaming or not.
Definition: videoSource.h:291
uint64_t mLastTimestamp
Definition: videoSource.h:380
bool Capture(T **image, int *status)
Capture the next image from the video stream, using the default timeout of 1000ms.
Definition: videoSource.h:211
@ ERROR
an error occurred
Definition: videoSource.h:126
imageFormat mRawFormat
Definition: videoSource.h:381
uint64_t GetFrameCount() const
Return the number of frames captured.
Definition: videoSource.h:311
URI resource
The resource URI of the device, IP stream, or file/directory.
Definition: videoOptions.h:49
uint32_t GetFrameRate() const
Return the framerate, in Hz or FPS.
Definition: videoSource.h:306
virtual uint32_t GetType() const
Return the interface type of the stream.
Definition: videoSource.h:341
imageFormat GetRawFormat() const
Get raw image format.
Definition: videoSource.h:321
Resource URI of a video device, IP stream, or file/directory.
Definition: URI.h:101
uint64_t GetLastTimestamp() const
Get timestamp of the last captured frame, in nanoseconds.
Definition: videoSource.h:316
videoOptions mOptions
Definition: videoSource.h:378
const char * TypeToStr() const
Convert this stream's class type to string.
Definition: videoSource.h:361
virtual ~videoSource()
Destroy interface and release all resources.
static const uint64_t DEFAULT_TIMEOUT
The default Capture timeout (1000ms)
Definition: videoSource.h:371
@ OK
frame capture successful
Definition: videoSource.h:129
Status
Stream status codes that are optionally returned from Capture()
Definition: videoSource.h:124
uint32_t GetHeight() const
Return the height of the stream, in pixels.
Definition: videoSource.h:301
#define VIDEO_SOURCE_USAGE_STRING
Standard command-line options able to be passed to videoSource::Create()
Definition: videoSource.h:36
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
uint32_t width
The width of the stream (in pixels).
Definition: videoOptions.h:64
const videoOptions & GetOptions() const
Return the videoOptions of the stream.
Definition: videoSource.h:331
uint64_t frameCount
The number of frames that have been captured or output on this interface.
Definition: videoOptions.h:83
float frameRate
The framerate of the stream (the default is 30Hz).
Definition: videoOptions.h:78
static videoSource * Create(const videoOptions &options)
Create videoSource interface from a videoOptions struct that's already been filled out.
uint32_t height
The height of the stream (in pixels).
Definition: videoOptions.h:71
The videoSource API is for capturing frames from video input devices such as MIPI CSI cameras,...
Definition: videoSource.h:118