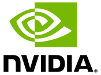 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GL_CAMERA_H__
24 #define __GL_CAMERA_H__
101 inline void SetFOV(
float fov ) { mFoV = fov; }
111 inline void SetNear(
float near ) { mNear = near; }
116 inline void SetFar(
float far ) { mFar = far; }
121 inline void SetEye(
float x,
float y,
float z ) { mEye[0] = x; mEye[1] = y; mEye[2] = z; }
126 inline void SetLookAt(
float x,
float y,
float z ) { mLookAt[0] = x; mLookAt[1] = y; mLookAt[2] = z; }
131 inline void SetRotation(
float yaw,
float pitch,
float roll ) { mRotation[0] = pitch; mRotation[1] = yaw; mRotation[2] = roll; }
136 inline void SetYaw(
float yaw ) { mRotation[1] = yaw; }
141 inline void SetPitch(
float pitch ) { mRotation[0] = pitch; }
146 inline void SetRoll(
float roll ) { mRotation[2] = roll; }
176 bool mouseInViewport()
const;
178 bool onEventLookAt( uint16_t msg,
int a,
int b );
179 bool onEventYawPitchRoll( uint16_t msg,
int a,
int b );
181 static bool onEvent( uint16_t msg,
int a,
int b,
void* user );
194 float mDefaultEye[3];
195 float mDefaultRotation[3];
196 float mDefaultLookAt[3];
198 float mPrevModelView[16];
199 float mPrevProjection[16];
201 float mMovementSpeed;
202 bool mMovementEnabled;
CameraMode
Enum specifying the camera mode.
Definition: glCamera.h:41
void SetRoll(float roll)
Set the roll angle, in radians.
Definition: glCamera.h:146
void SetNear(float near)
Set the distance to the near clipping plane.
Definition: glCamera.h:111
static glCamera * Create(CameraMode mode, int registerEvents=0)
Create OpenGL camera object with the specified CameraMode.
~glCamera()
Free the camera.
@ Ortho
Ortho (2D)
Definition: glCamera.h:45
void SetPitch(float pitch)
Set the pitch angle, in radians.
Definition: glCamera.h:141
void SetRotation(float yaw, float pitch, float roll)
Set the yaw/pitch/roll angles, in radians.
Definition: glCamera.h:131
void SetMovementSpeed(float speed)
Set the movement speed (in world units)
Definition: glCamera.h:151
void SetFar(float far)
Set the distance to the far clipping plane.
Definition: glCamera.h:116
void SetFOV(float fov)
Set the field of view (FOV), in degrees.
Definition: glCamera.h:101
void SetYaw(float yaw)
Set the yaw angle, in radians.
Definition: glCamera.h:136
void SetLookAt(float x, float y, float z)
Set the look-at point.
Definition: glCamera.h:126
void Reset()
Reset camera orientation to defaults.
@ YawPitchRoll
YawPitchRoll (first person.
Definition: glCamera.h:44
OpenGL perspective camera supporting Look-At, Yaw/Pitch/Roll, and Ortho modes.
Definition: glCamera.h:35
void Deactivate()
Restore previous GL_PROJECTION and GL_MODELVIEW matrices.
CameraMode GetCameraMode() const
Get the camera mode.
Definition: glCamera.h:91
void StoreDefaults()
Store the current configuration as defaults.
void SetMovementEnabled(bool enabled)
Enable or disable movement from user input.
Definition: glCamera.h:156
void Activate()
Activate GL_PROJECTION and GL_MODELVIEW matrices.
void RegisterEvents(uint32_t display=0)
Register to recieve input events (enable movement)
void SetEye(float x, float y, float z)
Set the eye position.
Definition: glCamera.h:121
void SetClippingPlane(float near, float far)
Set the near/far z-clipping plane.
Definition: glCamera.h:106
void SetCameraMode(CameraMode mode)
Set the camera mode.
Definition: glCamera.h:96
@ LookAt
LookAt (orbit)
Definition: glCamera.h:43