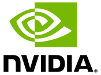 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __COMMAND_LINE_H_
24 #define __COMMAND_LINE_H_
60 bool GetFlag(
const char* argName,
bool allowOtherDelimiters=
true )
const;
73 float GetFloat(
const char* argName,
float defaultValue=0.0f,
bool allowOtherDelimiters=
true )
const;
86 int GetInt(
const char* argName,
int defaultValue=0,
bool allowOtherDelimiters=
true )
const;
99 uint32_t
GetUnsignedInt(
const char* argName, uint32_t defaultValue=0,
bool allowOtherDelimiters=
true )
const;
113 const char*
GetString(
const char* argName,
const char* defaultValue=NULL,
bool allowOtherDelimiters=
true )
const;
125 const char*
GetPosition(
unsigned int position,
const char* defaultValue=NULL )
const;
136 void AddArg(
const char* arg );
141 void AddArgs(
const char** args );
146 void AddFlag(
const char* flag );
169 #define ARG_POSITION(x) x
void AddArgs(const char **args)
Add arguments to the command line.
const char * GetPosition(unsigned int position, const char *defaultValue=NULL) const
Get positional string argument.
bool GetFlag(const char *argName, bool allowOtherDelimiters=true) const
Checks to see whether the specified flag was included on the command line.
void AddFlag(const char *flag)
Add a flag to the command line.
uint32_t GetUnsignedInt(const char *argName, uint32_t defaultValue=0, bool allowOtherDelimiters=true) const
Get unsigned integer argument.
unsigned int GetPositionArgs() const
Get the number of positional arguments in the command line.
int GetInt(const char *argName, int defaultValue=0, bool allowOtherDelimiters=true) const
Get integer argument.
float GetFloat(const char *argName, float defaultValue=0.0f, bool allowOtherDelimiters=true) const
Get float argument.
void AddArg(const char *arg)
Add an argument to the command line.
commandLine(const int argc, char **argv, const char *extraFlag=NULL)
Constructor, takes the command line from main()
char ** argv
The argument strings that the object was created with from main()
Definition: commandLine.h:161
int argc
The argument count that the object was created with from main()
Definition: commandLine.h:156
const char * GetString(const char *argName, const char *defaultValue=NULL, bool allowOtherDelimiters=true) const
Get string argument.
void Print() const
Print out the command line for reference.
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35