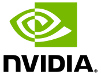 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __VIDEO_OPTIONS_H_
24 #define __VIDEO_OPTIONS_H_
273 void Print(
const char* prefix=NULL )
const;
278 bool Parse(
const char*
URI,
const int argc,
char** argv,
IoType ioType,
const char* extraFlag=NULL);
350 #define LOG_VIDEO "[video] "
static CodecType CodecTypeFromStr(const char *str)
Parse a Codec enum from a string.
static IoType IoTypeFromStr(const char *str)
Parse an IoType enum from a string.
@ FLIP_UPPER_RIGHT_DIAGONAL
Flip across upper right/lower left diagonal.
Definition: videoOptions.h:171
@ CODEC_MPEG4
MPEG4 (decode only)
Definition: videoOptions.h:207
@ CODEC_VP8
VP8.
Definition: videoOptions.h:204
@ DEVICE_DEFAULT
Unknown interface type.
Definition: videoOptions.h:134
DeviceType
Device interface types.
Definition: videoOptions.h:132
static const char * CodecToStr(Codec codec)
Convert a Codec enum to a string.
std::string sslKey
Path to a file containing a PEM-encoded private key.
Definition: videoOptions.h:268
static FlipMethod FlipMethodFromStr(const char *str)
Parse a FlipMethod enum from a string.
@ CODEC_NVENC
x86 only - NVENC hardware plugin (not currently implemented)
Definition: videoOptions.h:232
URI save
Optional path to save the compressed stream to a video file on disk, which is to be used in addition ...
Definition: videoOptions.h:57
int latency
Number of milliseconds of video to buffer for network RTSP or WebRTC streams.
Definition: videoOptions.h:127
static Codec CodecFromStr(const char *str)
Parse a Codec enum from a string.
@ DEVICE_FILE
Disk-based stream from a file or directory of files.
Definition: videoOptions.h:138
@ DEVICE_IP
IP-based network stream (e.g.
Definition: videoOptions.h:137
@ CODEC_H265
H.265.
Definition: videoOptions.h:203
@ FLIP_COUNTERCLOCKWISE
Rotate counter-clockwise 90 degrees.
Definition: videoOptions.h:167
bool Parse(const char *URI, const int argc, char **argv, IoType ioType, const char *extraFlag=NULL)
@ CODEC_H264
H.264.
Definition: videoOptions.h:202
@ INPUT
Input stream (e.g.
Definition: videoOptions.h:152
@ FLIP_NONE
Identity (no rotation)
Definition: videoOptions.h:166
URI resource
The resource URI of the device, IP stream, or file/directory.
Definition: videoOptions.h:49
Codec
Video codecs.
Definition: videoOptions.h:198
static const char * DeviceTypeToStr(DeviceType type)
Convert a DeviceType enum to a string.
@ CODEC_CPU
CPU-based implementation using libav (e.g.
Definition: videoOptions.h:229
@ FLIP_HORIZONTAL
Flip horizontally.
Definition: videoOptions.h:170
@ FLIP_ROTATE_180
Rotate 180 degrees.
Definition: videoOptions.h:168
static const char * IoTypeToStr(IoType type)
Convert an IoType enum to a string.
@ CODEC_UNKNOWN
Unknown/unsupported codec.
Definition: videoOptions.h:200
void Print(const char *prefix=NULL) const
Log the video settings, with an optional prefix label.
videoOptions()
Constructor using default options.
@ CODEC_RAW
Uncompressed (e.g.
Definition: videoOptions.h:201
Resource URI of a video device, IP stream, or file/directory.
Definition: URI.h:101
@ FLIP_VERTICAL
Flip vertically.
Definition: videoOptions.h:172
@ CODEC_NVDEC
x86 only - NVDEC hardware plugin (not currently implemented)
Definition: videoOptions.h:233
static const char * FlipMethodToStr(FlipMethod flip)
Convert a FlipMethod enum to a string.
uint32_t bitRate
The encoding bitrate for compressed streams (only applies to video codecs like H264/H265).
Definition: videoOptions.h:90
static DeviceType DeviceTypeFromStr(const char *str)
Parse a DeviceType enum from a string.
@ CODEC_V4L2
aarch64 & JetPack 5 only - V4L2 hardware plugins (e.g.
Definition: videoOptions.h:231
@ FLIP_CLOCKWISE
Rotate clockwise 90 degrees.
Definition: videoOptions.h:169
@ CODEC_OMX
aarch64 & JetPack 4 only - OMX hardware plugins (e.g.
Definition: videoOptions.h:230
@ FLIP_UPPER_LEFT_DIAGONAL
Flip across upper left/lower right diagonal.
Definition: videoOptions.h:173
@ CODEC_MJPEG
MJPEG.
Definition: videoOptions.h:208
std::string stunServer
URL of STUN server used for WebRTC.
Definition: videoOptions.h:250
int loop
Control the number of loops for videoSource disk-based inputs (for example, the number of times that ...
Definition: videoOptions.h:119
bool zeroCopy
If true, indicates the buffers are allocated in zeroCopy memory that is mapped to both the CPU and GP...
Definition: videoOptions.h:104
static const char * CodecTypeToStr(CodecType codecType)
Convert a CodecType enum to a string.
std::string sslCert
Path to a file containing a PEM-encoded SSL/TLS certificate.
Definition: videoOptions.h:259
@ OUTPUT
Output stream (e.g.
Definition: videoOptions.h:153
Codec codec
Indicates the codec used by the stream.
Definition: videoOptions.h:222
IoType ioType
Indicates if this stream is an input or an output.
Definition: videoOptions.h:159
@ CODEC_VP9
VP9.
Definition: videoOptions.h:205
@ CODEC_MPEG2
MPEG2 (decode only)
Definition: videoOptions.h:206
CodecType
Video codec engines.
Definition: videoOptions.h:227
IoType
Input/Output stream type.
Definition: videoOptions.h:150
@ DEVICE_DISPLAY
OpenGL output stream rendered to an attached display.
Definition: videoOptions.h:139
@ FLIP_DEFAULT
Default setting (none)
Definition: videoOptions.h:174
CodecType codecType
Indicates the underlying hardware/software engine used by the codec.
Definition: videoOptions.h:242
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
FlipMethod flipMethod
The flip method controls if and how an input frame is flipped/rotated in pre-processing from a MIPI C...
Definition: videoOptions.h:193
uint32_t numBuffers
The number of ring buffers used for threading.
Definition: videoOptions.h:97
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
FlipMethod
Settings of the flip method used by MIPI CSI cameras and compressed video inputs.
Definition: videoOptions.h:164
uint32_t width
The width of the stream (in pixels).
Definition: videoOptions.h:64
@ DEVICE_V4L2
V4L2 webcam (e.g.
Definition: videoOptions.h:135
@ DEVICE_CSI
MIPI CSI camera.
Definition: videoOptions.h:136
uint64_t frameCount
The number of frames that have been captured or output on this interface.
Definition: videoOptions.h:83
DeviceType deviceType
Indicates the type of device interface used by this stream.
Definition: videoOptions.h:145
float frameRate
The framerate of the stream (the default is 30Hz).
Definition: videoOptions.h:78
uint32_t height
The height of the stream (in pixels).
Definition: videoOptions.h:71