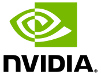 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __V4L2_CAPTURE_H__
24 #define __V4L2_CAPTURE_H__
26 #include <linux/videodev2.h>
68 void*
Capture(
size_t timeout=0 );
73 inline uint32_t
GetWidth()
const {
return mWidth; }
78 inline uint32_t
GetHeight()
const {
return mHeight; }
83 inline uint32_t
GetPitch()
const {
return mPitch; }
104 uint32_t mRequestWidth;
105 uint32_t mRequestHeight;
109 uint32_t mPixelDepth;
113 struct v4l2_buffer buf;
117 v4l2_mmap* mBuffersMMap;
118 size_t mBufferCountMMap;
120 std::vector<v4l2_fmtdesc> mFormats;
121 std::string mDevicePath;
Video4Linux2 (V4L2) camera capture streaming.
Definition: v4l2Camera.h:41
bool Close()
Stop streaming.
void * Capture(size_t timeout=0)
Return the next image.
uint32_t GetPitch() const
Return the size in bytes of one line of the image.
Definition: v4l2Camera.h:83
uint32_t GetHeight() const
Retrieve height, in pixels, of camera image.
Definition: v4l2Camera.h:78
static v4l2Camera * Create(const char *device_path)
Create V4L2 interface.
uint32_t GetWidth() const
Get width, in pixels, of camera image.
Definition: v4l2Camera.h:73
bool Open()
Start streaming.
uint32_t GetPixelDepth() const
Return the bit depth per pixel.
Definition: v4l2Camera.h:88