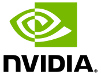 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __OBJECT_TRACKER_H__
24 #define __OBJECT_TRACKER_H__
34 #define OBJECT_TRACKER_USAGE_STRING "objectTracker arguments: \n" \
35 " --tracking flag to enable default tracker (IOU)\n" \
36 " --tracker=TRACKER enable tracking with 'IOU' or 'KLT'\n" \
37 " --tracker-min-frames=N the number of re-identified frames for a track to be considered valid (default: 3)\n" \
38 " --tracker-drop-frames=N number of consecutive lost frames before a track is dropped (default: 15)\n" \
39 " --tracker-overlap=N how much IOU overlap is required for a bounding box to be matched (default: 0.5)\n\n" \
45 #define LOG_TRACKER "[tracker] "
88 template<
typename T>
int Process( T* image, uint32_t width, uint32_t height,
detectNet::Detection* detections,
int numDetections ) {
return Process((
void*)image, width, height, imageFormatFromType<T>(), detections, numDetections); }
bool IsType(Type type) const
IsType.
Definition: objectTracker.h:113
#define OBJECT_TRACKER_USAGE_STRING
Standard command-line options able to be passed to detectNet::Create()
Definition: objectTracker.h:34
virtual ~objectTracker()
Destructor.
Object Detection result.
Definition: detectNet.h:122
static const char * TypeToStr(Type type)
Convert a Type enum to string.
virtual Type GetType() const =0
GetType.
@ IOU
Intersection-Over-Union (IOU) tracker.
Definition: objectTracker.h:61
Object tracker interface.
Definition: objectTracker.h:52
bool IsEnabled() const
IsEnabled.
Definition: objectTracker.h:98
Type
Tracker type enum.
Definition: objectTracker.h:58
@ NONE
Tracking disabled.
Definition: objectTracker.h:60
static const char * Usage()
Usage string for command line arguments to Create()
Definition: objectTracker.h:118
@ KLT
KLT tracker (only available with VPI)
Definition: objectTracker.h:62
virtual void SetEnabled(bool enabled)
SetEnabled.
Definition: objectTracker.h:103
int Process(T *image, uint32_t width, uint32_t height, detectNet::Detection *detections, int numDetections)
Process.
Definition: objectTracker.h:88
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
static objectTracker * Create(Type type)
Create a new object tracker.
bool mEnabled
Definition: objectTracker.h:133
static Type TypeFromStr(const char *str)
Parse a Type enum from a string.