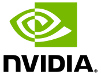 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __OBJECT_TRACKER_KLT_H__
24 #define __OBJECT_TRACKER_KLT_H__
28 #include <vpi/Array.h>
29 #include <vpi/Image.h>
30 #include <vpi/Status.h>
31 #include <vpi/Stream.h>
32 #include <vpi/algo/KLTFeatureTracker.h>
48 static objectTrackerKLT*
Create();
53 static objectTrackerKLT*
Create(
int argc,
char** argv );
68 inline virtual Type
GetType()
const {
return KLT; }
78 bool init( uint32_t width, uint32_t height,
imageFormat format );
90 VPIArray mOutputBoxes;
91 VPIArray mOutputPreds;
94 VPIKLTFeatureTrackerParams mParams;
96 std::vector<VPIKLTTrackedBoundingBox> mBoxes;
97 std::vector<VPIHomographyTransform2D> mPreds;
Object Detection result.
Definition: detectNet.h:122
virtual Type GetType() const =0
GetType.
Object tracker interface.
Definition: objectTracker.h:52
@ KLT
KLT tracker (only available with VPI)
Definition: objectTracker.h:62
int Process(T *image, uint32_t width, uint32_t height, detectNet::Detection *detections, int numDetections)
Process.
Definition: objectTracker.h:88
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
static objectTracker * Create(Type type)
Create a new object tracker.