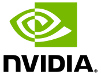 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __OBJECT_TRACKER_IOU_H__
24 #define __OBJECT_TRACKER_IOU_H__
34 #define OBJECT_TRACKER_DEFAULT_MIN_FRAMES 3
40 #define OBJECT_TRACKER_DEFAULT_DROP_FRAMES 15
45 #define OBJECT_TRACKER_DEFAULT_OVERLAP_THRESHOLD 0.5
127 objectTrackerIOU( uint32_t minFrames, uint32_t dropFrames,
float overlapThreshold );
#define OBJECT_TRACKER_DEFAULT_MIN_FRAMES
The number of re-identified frames before establishing a track.
Definition: objectTrackerIOU.h:34
uint32_t mIDCount
Definition: objectTrackerIOU.h:129
uint64_t mFrameCount
Definition: objectTrackerIOU.h:130
void SetOverlapThreshold(float threshold)
Set how much IOU overlap is required for a bounding box to be matched.
Definition: objectTrackerIOU.h:114
Object Detection result.
Definition: detectNet.h:122
void SetDropFrames(uint32_t frames)
Set the number of consecutive lost frames after which a track is removed.
Definition: objectTrackerIOU.h:104
Object tracker using Intersection-Over-Union (IOU)
Definition: objectTrackerIOU.h:59
virtual Type GetType() const
Definition: objectTrackerIOU.h:119
uint32_t GetMinFrames() const
The number of re-identified frames before before establishing a track.
Definition: objectTrackerIOU.h:89
uint32_t mMinFrames
Definition: objectTrackerIOU.h:132
objectTrackerIOU(uint32_t minFrames, uint32_t dropFrames, float overlapThreshold)
virtual ~objectTrackerIOU()
Destroy.
float GetOverlapThreshold() const
How much IOU overlap is required for a bounding box to be matched.
Definition: objectTrackerIOU.h:109
@ IOU
Intersection-Over-Union (IOU) tracker.
Definition: objectTracker.h:61
std::vector< detectNet::Detection > mTracks
Definition: objectTrackerIOU.h:137
Object tracker interface.
Definition: objectTracker.h:52
#define OBJECT_TRACKER_DEFAULT_OVERLAP_THRESHOLD
How much IOU overlap is required for a bounding box to be matched.
Definition: objectTrackerIOU.h:45
Type
Tracker type enum.
Definition: objectTracker.h:58
uint32_t GetDropFrames() const
The number of consecutive lost frames after which a track is removed.
Definition: objectTrackerIOU.h:99
float mOverlapThreshold
Definition: objectTrackerIOU.h:135
void SetMinFrames(uint32_t frames)
Set the number of re-identified frames before before establishing a track.
Definition: objectTrackerIOU.h:94
uint32_t mDropFrames
Definition: objectTrackerIOU.h:133
#define OBJECT_TRACKER_DEFAULT_DROP_FRAMES
The number of consecutive lost frames after which a track is removed.
Definition: objectTrackerIOU.h:40
static objectTrackerIOU * Create(uint32_t minFrames=OBJECT_TRACKER_DEFAULT_MIN_FRAMES, uint32_t dropFrames=OBJECT_TRACKER_DEFAULT_DROP_FRAMES, float overlapThreshold=OBJECT_TRACKER_DEFAULT_OVERLAP_THRESHOLD)
Create a new object tracker.
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
virtual int Process(void *image, uint32_t width, uint32_t height, imageFormat format, detectNet::Detection *detections, int numDetections)