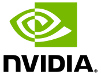 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __LOGGING_UTILS_H_
24 #define __LOGGING_UTILS_H_
36 #define LOG_USAGE_STRING "logging arguments: \n" \
37 " --log-file=FILE output destination file (default is stdout)\n" \
38 " --log-level=LEVEL message output threshold, one of the following:\n" \
44 " * verbose (default)\n" \
46 " --verbose enable verbose logging (same as --log-level=verbose)\n" \
47 " --debug enable debug logging (same as --log-level=debug)\n\n"
99 static void SetFile( FILE* file );
105 static void SetFile(
const char* filename );
115 static void ParseCmdLine(
const int argc,
char** argv );
144 #define GenericLogMessage(level, format, args...) if( level <= Log::GetLevel() ) fprintf(Log::GetFile(), format, ## args)
150 #define LogError(format, args...) GenericLogMessage(Log::ERROR, LOG_COLOR_RED LOG_LEVEL_PREFIX_ERROR format LOG_COLOR_RESET, ## args)
156 #define LogWarning(format, args...) GenericLogMessage(Log::WARNING, LOG_COLOR_YELLOW LOG_LEVEL_PREFIX_WARNING format LOG_COLOR_RESET, ## args)
162 #define LogSuccess(format, args...) GenericLogMessage(Log::SUCCESS, LOG_COLOR_GREEN LOG_LEVEL_PREFIX_SUCCESS format LOG_COLOR_RESET, ## args)
168 #define LogInfo(format, args...) GenericLogMessage(Log::INFO, LOG_LEVEL_PREFIX_INFO format, ## args)
174 #define LogVerbose(format, args...) GenericLogMessage(Log::VERBOSE, LOG_LEVEL_PREFIX_VERBOSE format, ## args)
180 #define LogDebug(format, args...) GenericLogMessage(Log::DEBUG, LOG_LEVEL_PREFIX_DEBUG format, ## args)
191 #ifdef LOG_DISABLE_COLORS
192 #define LOG_COLOR_RESET ""
193 #define LOG_COLOR_RED ""
194 #define LOG_COLOR_GREEN ""
195 #define LOG_COLOR_YELLOW ""
196 #define LOG_COLOR_BLUE ""
197 #define LOG_COLOR_MAGENTA ""
198 #define LOG_COLOR_CYAN ""
199 #define LOG_COLOR_LIGHT_GRAY ""
200 #define LOG_COLOR_DARK_GRAY ""
203 #define LOG_COLOR_RESET "\033[0m"
204 #define LOG_COLOR_RED "\033[0;31m"
205 #define LOG_COLOR_GREEN "\033[0;32m"
206 #define LOG_COLOR_YELLOW "\033[0;33m"
207 #define LOG_COLOR_BLUE "\033[0;34m"
208 #define LOG_COLOR_MAGENTA "\033[0;35m"
209 #define LOG_COLOR_CYAN "\033[0;36m"
210 #define LOG_COLOR_LIGHT_GRAY "\033[0;37m"
211 #define LOG_COLOR_DARK_GRAY "\033[0;90m"
214 #ifdef LOG_ENABLE_LEVEL_PREFIX
215 #define LOG_LEVEL_PREFIX_ERROR "[E]"
216 #define LOG_LEVEL_PREFIX_WARNING "[W]"
217 #define LOG_LEVEL_PREFIX_SUCCESS "[S]"
218 #define LOG_LEVEL_PREFIX_INFO "[I]"
219 #define LOG_LEVEL_PREFIX_VERBOSE "[V]"
220 #define LOG_LEVEL_PREFIX_DEBUG "[D]"
222 #define LOG_LEVEL_PREFIX_ERROR ""
223 #define LOG_LEVEL_PREFIX_WARNING ""
224 #define LOG_LEVEL_PREFIX_SUCCESS ""
225 #define LOG_LEVEL_PREFIX_INFO ""
226 #define LOG_LEVEL_PREFIX_VERBOSE ""
227 #define LOG_LEVEL_PREFIX_DEBUG ""
static const char * Usage()
Usage string for command line arguments to Create()
Definition: logging.h:110
Level
Defines the logging level of a message, and the threshold used by the logger to either drop or output...
Definition: logging.h:61
static const char * LevelToStr(Level level)
Convert a logging level to string.
@ DEFAULT
The default level is VERBOSE
Definition: logging.h:70
static void SetFile(FILE *file)
Set the logging output.
static void SetLevel(Level level)
Set the current logging level.
Definition: logging.h:81
@ WARNING
Warning conditions where the application may be able to proceed in some capacity.
Definition: logging.h:65
@ VERBOSE
Verbose details about program execution.
Definition: logging.h:68
@ SILENT
No messages are output.
Definition: logging.h:63
static Level mLevel
Definition: logging.h:133
static const char * GetFilename()
Get the filename of the log output.
Definition: logging.h:92
static Level LevelFromStr(const char *str)
Parse a logging level from a string.
static Level GetLevel()
Get the current logging level.
Definition: logging.h:76
@ SUCCESS
Successful events (e.g.
Definition: logging.h:66
static std::string mFilename
Definition: logging.h:135
Message logging with a variable level of output and destinations.
Definition: logging.h:54
static FILE * mFile
Definition: logging.h:134
#define LOG_USAGE_STRING
Standard command-line options able to be passed to videoOutput::Create()
Definition: logging.h:36
@ INFO
Informational messages that are more important than VERBOSE messages.
Definition: logging.h:67
static void ParseCmdLine(const int argc, char **argv)
Parse command line options (see Usage() above)
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
@ ERROR
Major errors that may impact application execution.
Definition: logging.h:64
static FILE * GetFile()
Get the current log output.
Definition: logging.h:86
@ DEBUG
Low-level debugging (disabled by default)
Definition: logging.h:69