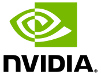 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __IMAGE_WRITER_H_
24 #define __IMAGE_WRITER_H_
72 template<
typename T>
bool Render( T* image, uint32_t width, uint32_t height ) {
return Render((
void**)image, width, height, imageFormatFromType<T>()); }
78 virtual bool Render(
void* image, uint32_t width, uint32_t height,
imageFormat format );
88 static const uint32_t
Type = (1 << 5);
static const uint32_t Type
Unique type identifier of imageWriter class.
Definition: imageWriter.h:88
uint32_t mFileCount
Definition: imageWriter.h:113
char mFileOut[1024]
Definition: imageWriter.h:114
virtual ~imageWriter()
Destructor.
static const char * SupportedExtensions[]
String array of supported image file extensions, terminated with a NULL sentinel value.
Definition: imageWriter.h:101
virtual uint32_t GetType() const
Return the interface type (imageWriter::Type)
Definition: imageWriter.h:83
imageWriter(const videoOptions &options)
static imageWriter * Create(const char *path, const videoOptions &options=videoOptions())
Create an imageWriter instance from a path and optional videoOptions.
Save an image or set of images to disk.
Definition: imageWriter.h:50
static bool IsSupportedExtension(const char *ext)
Return true if the extension is in the list of SupportedExtensions.
The videoOutput API is for rendering and transmitting frames to video input devices such as display w...
Definition: videoOutput.h:104
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
bool Render(T *image, uint32_t width, uint32_t height)
Save the next frame.
Definition: imageWriter.h:72