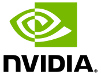 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __IMAGE_NET_H__
24 #define __IMAGE_NET_H__
34 #define IMAGENET_DEFAULT_INPUT "data"
40 #define IMAGENET_DEFAULT_OUTPUT "prob"
46 #define IMAGENET_DEFAULT_THRESHOLD 0.01f
52 #define IMAGENET_MODEL_TYPE "classification"
58 #define IMAGENET_USAGE_STRING "imageNet arguments: \n" \
59 " --network=NETWORK pre-trained model to load, one of the following:\n" \
61 " * googlenet (default)\n" \
70 " --model=MODEL path to custom model to load (caffemodel, uff, or onnx)\n" \
71 " --prototxt=PROTOTXT path to custom prototxt to load (for .caffemodel only)\n" \
72 " --labels=LABELS path to text file containing the labels for each class\n" \
73 " --input-blob=INPUT name of the input layer (default is '" IMAGENET_DEFAULT_INPUT "')\n" \
74 " --output-blob=OUTPUT name of the output layer (default is '" IMAGENET_DEFAULT_OUTPUT "')\n" \
75 " --threshold=CONF minimum confidence threshold for classification (default is 0.01)\n" \
76 " --smoothing=WEIGHT weight between [0,1] or number of frames (disabled by default)\n" \
77 " --profile enable layer profiling in TensorRT\n\n"
117 static imageNet*
Create(
const char* prototxt_path,
const char* model_path,
118 const char* mean_binary,
const char* class_labels,
158 template<
typename T>
int Classify( T* image, uint32_t width, uint32_t height,
float* confidence=NULL ) {
return Classify((
void*)image, width, height, imageFormatFromType<T>(), confidence); }
174 int Classify(
void* image, uint32_t width, uint32_t height,
imageFormat format,
float* confidence=NULL );
208 template<
typename T>
int Classify( T* image, uint32_t width, uint32_t height,
Classifications& classifications,
int topK=1 ) {
return Classify((
void*)image, width, height, imageFormatFromType<T>(), classifications, topK); }
295 bool init(
const char* prototxt_path,
const char* model_path,
const char* mean_binary,
const char* class_path,
const char* input,
const char* output, uint32_t maxBatchSize,
precisionType precision,
deviceType device,
bool allowGPUFallback );
296 bool loadClassInfo(
const char* filename,
int expectedClasses=-1 );
int Classify(T *image, uint32_t width, uint32_t height, float *confidence=NULL)
Predict the maximum-likelihood image class whose confidence meets the minimum threshold.
Definition: imageNet.h:158
static const char * Usage()
Usage string for command line arguments to Create()
Definition: imageNet.h:138
#define IMAGENET_DEFAULT_INPUT
Name of default input blob for imageNet model.
Definition: imageNet.h:34
int Classify(T *image, uint32_t width, uint32_t height, Classifications &classifications, int topK=1)
Classify the image and return the topK image classification results that meet the minimum confidence ...
Definition: imageNet.h:208
float GetSmoothing() const
Return the temporal smoothing weight or number of frames in the smoothing window.
Definition: imageNet.h:269
const char * GetClassSynset(int index) const
Retrieve the class synset category of a particular class.
Definition: imageNet.h:246
#define IMAGENET_DEFAULT_OUTPUT
Name of default output confidence values for imageNet model.
Definition: imageNet.h:40
float * mSmoothingBuffer
Definition: imageNet.h:310
void SetSmoothing(float factor)
Enable temporal smoothing of the results using EWMA (exponentially-weighted moving average).
Definition: imageNet.h:289
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
const char * GetClassLabel(int index) const
Retrieve the description of a particular class.
Definition: imageNet.h:236
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
uint32_t GetNumClasses() const
Retrieve the number of image recognition classes (typically 1000)
Definition: imageNet.h:231
std::vector< std::string > mClassSynset
Definition: imageNet.h:304
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
std::vector< std::pair< uint32_t, float > > Classifications
List of classification results where each entry represents a (classID, confidence) pair.
Definition: imageNet.h:90
float GetThreshold() const
Return the confidence threshold used for classification.
Definition: imageNet.h:256
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
bool preProcess(void *image, uint32_t width, uint32_t height, imageFormat format)
virtual ~imageNet()
Destroy.
std::vector< std::string > mClassDesc
Definition: imageNet.h:305
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
const char * GetClassPath() const
Retrieve the path to the file containing the class descriptions.
Definition: imageNet.h:251
Image recognition with classification networks, using TensorRT.
Definition: imageNet.h:84
float mThreshold
Definition: imageNet.h:313
std::string mClassPath
Definition: imageNet.h:307
uint32_t mNumClasses
Definition: imageNet.h:302
#define IMAGENET_USAGE_STRING
Standard command-line options able to be passed to imageNet::Create()
Definition: imageNet.h:58
const char * GetClassDesc(int index) const
Retrieve the description of a particular class.
Definition: imageNet.h:241
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
float mSmoothingFactor
Definition: imageNet.h:311
bool init(const char *prototxt_path, const char *model_path, const char *mean_binary, const char *class_path, const char *input, const char *output, uint32_t maxBatchSize, precisionType precision, deviceType device, bool allowGPUFallback)
static imageNet * Create(const char *network="googlenet", uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load one of the following pre-trained models:
bool loadClassInfo(const char *filename, int expectedClasses=-1)
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
void SetThreshold(float threshold)
Set the confidence threshold used for classification.
Definition: imageNet.h:263