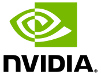 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __IMAGE_LOADER_H_
24 #define __IMAGE_LOADER_H_
104 static const uint32_t
Type = (1 << 4);
Load an image or set of images from disk into GPU memory.
Definition: imageLoader.h:52
size_t mLoopCount
Definition: imageLoader.h:137
bool IsEOS() const
Return true if End Of Stream (EOS) has been reached.
Definition: imageLoader.h:94
bool isLooping() const
Definition: imageLoader.h:134
virtual void Close()
Close the stream.
static const uint32_t Type
Unique type identifier of imageLoader class.
Definition: imageLoader.h:104
virtual uint32_t GetType() const
Return the interface type (imageLoader::Type)
Definition: imageLoader.h:99
static const char * SupportedExtensions[]
String array of supported image file extensions, terminated with a NULL sentinel value.
Definition: imageLoader.h:122
static imageLoader * Create(const char *path, const videoOptions &options=videoOptions())
Create an imageLoader instance from a path and optional videoOptions.
std::vector< std::string > mFiles
Definition: imageLoader.h:140
bool mEOS
Definition: imageLoader.h:136
size_t mNextFile
Definition: imageLoader.h:138
virtual ~imageLoader()
Destructor.
virtual bool Capture(void **image, imageFormat format, uint64_t timeout=DEFAULT_TIMEOUT, int *status=NULL)
Load the next frame.
int loop
Control the number of loops for videoSource disk-based inputs (for example, the number of times that ...
Definition: videoOptions.h:119
videoOptions mOptions
Definition: videoSource.h:378
static bool IsSupportedExtension(const char *ext)
Return true if the extension is in the list of SupportedExtensions.
static const uint64_t DEFAULT_TIMEOUT
The default Capture timeout (1000ms)
Definition: videoSource.h:371
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
imageLoader(const videoOptions &options)
virtual bool Open()
Open the stream.
std::vector< void * > mBuffers
Definition: imageLoader.h:141
The videoSource API is for capturing frames from video input devices such as MIPI CSI cameras,...
Definition: videoSource.h:118