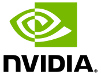 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
60 template<
typename T>
bool loadImage(
const char* filename, T** ptr,
int* width,
int* height ) {
return loadImage(filename, (
void**)ptr, width, height, imageFormatFromType<T>()); }
91 bool loadImage(
const char* filename,
void** output,
int* width,
int* height,
imageFormat format );
100 bool loadImageRGBA(
const char* filename, float4** ptr,
int* width,
int* height );
110 bool loadImageRGBA(
const char* filename, float4** cpu, float4** gpu,
int* width,
int* height );
139 template<
typename T>
bool saveImage(
const char* filename, T* ptr,
int width,
int height,
int quality=95,
140 const float2& pixel_range=
make_float2(0,255),
bool sync=
true ) {
return saveImage(filename, (
void*)ptr, width, height, imageFormatFromType<T>(), quality, pixel_range, sync); }
169 int quality=95,
const float2& pixel_range=
make_float2(0,255),
bool sync=
true );
178 bool saveImageRGBA(
const char* filename, float4* ptr,
int width,
int height,
float max_pixel=255.0f,
int quality=100 );
185 #define LOG_IMAGE "[image] "
bool loadImage(const char *filename, T **ptr, int *width, int *height)
Load a color image from disk into CUDA memory, in uchar3/uchar4/float3/float4 formats with pixel valu...
Definition: imageIO.h:60
bool loadImageRGBA(const char *filename, float4 **ptr, int *width, int *height)
Load a color image from disk into CUDA memory with alpha, in float4 RGBA format with pixel values 0-2...
bool saveImage(const char *filename, T *ptr, int width, int height, int quality=95, const float2 &pixel_range=make_float2(0, 255), bool sync=true)
Save an image in CPU/GPU shared memory to disk.
Definition: imageIO.h:139
__host__ __device__ float2 make_float2(float s)
Definition: cudaMath.h:81
bool saveImageRGBA(const char *filename, float4 *ptr, int width, int height, float max_pixel=255.0f, int quality=100)
Save a float4 image in CPU/GPU shared memory to disk.