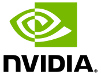 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GSTREAMER_ENCODER_H__
24 #define __GSTREAMER_ENCODER_H__
73 template<
typename T>
bool Render( T* image, uint32_t width, uint32_t height ) {
return Render((
void**)image, width, height, imageFormatFromType<T>()); }
79 virtual bool Render(
void* image, uint32_t width, uint32_t height,
imageFormat format );
111 static const uint32_t
Type = (1 << 2);
143 bool encodeYUV(
void* buffer,
size_t size );
146 static void onNeedData( GstElement* pipeline, uint32_t size,
void* user_data );
147 static void onEnoughData( GstElement* pipeline,
void* user_data );
static void onEnoughData(GstElement *pipeline, void *user_data)
std::string mLaunchStr
Definition: gstEncoder.h:159
RTSP server for transmitting encoded GStreamer pipelines to client devices.
Definition: RTSPServer.h:55
static const char * SupportedExtensions[]
String array of supported video file extensions, terminated with a NULL sentinel value.
Definition: gstEncoder.h:124
RingBuffer mBufferYUV
Definition: gstEncoder.h:161
static const uint32_t Type
Unique type identifier of gstEncoder class.
Definition: gstEncoder.h:111
virtual bool Open()
Open the stream.
GstBus * mBus
Definition: gstEncoder.h:152
WebRTC signalling server for establishing and negotiating connections with peers for bi-directional m...
Definition: WebRTCServer.h:116
GstElement * mPipeline
Definition: gstEncoder.h:155
WebRTCServer * GetWebRTCServer() const
Return the WebRTC server (only used when the protocol is "webrtc://")
Definition: gstEncoder.h:101
static gstEncoder * Create(const videoOptions &options)
Create an encoder from the provided video options.
GstCaps * mBufferCaps
Definition: gstEncoder.h:153
virtual uint32_t GetType() const
Return the interface type (gstEncoder::Type)
Definition: gstEncoder.h:106
GstElement * mAppSrc
Definition: gstEncoder.h:154
Codec
Video codecs.
Definition: videoOptions.h:198
virtual void Close()
Close the stream.
bool encodeYUV(void *buffer, size_t size)
Resource URI of a video device, IP stream, or file/directory.
Definition: URI.h:101
static void onWebsocketMessage(WebRTCPeer *peer, const char *message, size_t message_size, void *user_data)
std::string mCapsStr
Definition: gstEncoder.h:158
static bool IsSupportedExtension(const char *ext)
Return true if the extension is in the list of SupportedExtensions.
bool Render(T *image, uint32_t width, uint32_t height)
Encode the next frame.
Definition: gstEncoder.h:73
Thread-safe circular ring buffer queue.
Definition: RingBuffer.h:33
GstPipeline * GetPipeline() const
Return the GStreamer pipeline object.
Definition: gstEncoder.h:96
gstEncoder(const videoOptions &options)
The videoOutput API is for rendering and transmitting frames to video input devices such as display w...
Definition: videoOutput.h:104
RTSPServer * mRTSPServer
Definition: gstEncoder.h:163
bool mNeedData
Definition: gstEncoder.h:156
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
Remote peer that has connected.
Definition: WebRTCServer.h:85
WebRTCServer * mWebRTCServer
Definition: gstEncoder.h:164
static void onNeedData(GstElement *pipeline, uint32_t size, void *user_data)
Hardware-accelerated video encoder for Jetson using GStreamer.
Definition: gstEncoder.h:51