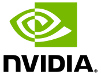 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GSTREAMER_CAMERA_H__
24 #define __GSTREAMER_CAMERA_H__
106 static gstCamera*
Create( uint32_t width, uint32_t height,
const char* camera=NULL );
132 virtual void Close();
189 static const uint32_t
Type = (1 << 0);
202 static void onEOS(_GstAppSink* sink,
void* user_data);
203 static GstFlowReturn onPreroll(_GstAppSink* sink,
void* user_data);
204 static GstFlowReturn onBuffer(_GstAppSink* sink,
void* user_data);
210 bool buildLaunchStr();
215 bool matchCaps( GstCaps* caps );
216 bool printCaps( GstCaps* caps );
220 _GstAppSink* mAppSink;
221 _GstElement* mPipeline;
223 std::string mLaunchStr;
bool CaptureRGBA(float **image, uint64_t timeout=DEFAULT_TIMEOUT, bool zeroCopy=false)
Capture the next image frame from the camera and convert it to float4 RGBA format,...
virtual void Close()
Stop streaming the camera.
static gstCamera * Create(const videoOptions &options)
Create a MIPI CSI or V4L2 camera device.
gstBufferManager recieves GStreamer buffers from appsink elements and unpacks/maps them into CUDA add...
Definition: gstBufferManager.h:61
MIPI CSI and V4L2 camera capture using GStreamer and nvarguscamerasrc or v4l2src elements.
Definition: gstCamera.h:57
void SetZeroCopy(bool zeroCopy)
Set whether converted RGB(A) images should use ZeroCopy buffer allocation.
Definition: gstCamera.h:179
static const uint32_t DefaultWidth
Default camera width, unless otherwise specified during Create()
Definition: gstCamera.h:194
Codec
Video codecs.
Definition: videoOptions.h:198
~gstCamera()
Release the camera interface and resources.
virtual bool Capture(void **image, imageFormat format, uint64_t timeout=DEFAULT_TIMEOUT, int *status=NULL)
Capture the next image frame from the camera.
videoOptions mOptions
Definition: videoSource.h:378
bool zeroCopy
If true, indicates the buffers are allocated in zeroCopy memory that is mapped to both the CPU and GP...
Definition: videoOptions.h:104
virtual uint32_t GetType() const
Return the interface type (gstCamera::Type)
Definition: gstCamera.h:184
static const uint64_t DEFAULT_TIMEOUT
The default Capture timeout (1000ms)
Definition: videoSource.h:371
static const uint32_t DefaultHeight
Default camera height, unless otherwise specified during Create()
Definition: gstCamera.h:199
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
virtual bool Open()
Begin streaming the camera.
static const uint32_t Type
Unique type identifier of gstCamera class.
Definition: gstCamera.h:189
The videoSource API is for capturing frames from video input devices such as MIPI CSI cameras,...
Definition: videoSource.h:118