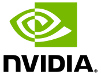 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GSTREAMER_BUFFER_MANAGER_H__
24 #define __GSTREAMER_BUFFER_MANAGER_H__
35 #if !GST_CHECK_VERSION(1,0,0)
36 #undef ENABLE_NVMM // NVMM is only enabled for GStreamer 1.0 and newer
40 #if NV_TENSORRT_MAJOR > 8 || (NV_TENSORRT_MAJOR == 8 && NV_TENSORRT_MINOR >= 4)
41 #undef ENABLE_NVMM // debug NVMM under JetPack 5
45 #define GST_CAPS_FEATURE_MEMORY_NVMM "memory:NVMM"
77 bool Enqueue( GstBuffer* buffer, GstCaps* caps );
Event mWaitEvent
Event that gets triggered when a new frame is recieved.
Definition: gstBufferManager.h:106
bool Enqueue(GstBuffer *buffer, GstCaps *caps)
Enqueue a GstBuffer from GStreamer.
imageFormat mFormatYUV
The YUV colorspace format coming from appsink (typically NV12 or YUY2)
Definition: gstBufferManager.h:101
RingBuffer mBufferYUV
Ringbuffer of CPU-based YUV frames (non-NVMM) that come from appsink.
Definition: gstBufferManager.h:102
Event object for signalling other threads.
Definition: Event.h:33
videoOptions * mOptions
Options of the gstDecoder / gstCamera object.
Definition: gstBufferManager.h:108
gstBufferManager(videoOptions *options)
Constructor.
A lightweight mutual exclusion lock.
Definition: Mutex.h:35
gstBufferManager recieves GStreamer buffers from appsink elements and unpacks/maps them into CUDA add...
Definition: gstBufferManager.h:61
uint64_t GetLastTimestamp() const
Get timestamp of the latest dequeued frame.
Definition: gstBufferManager.h:87
RingBuffer mBufferRGB
Ringbuffer of frames that have been converted to RGB colorspace.
Definition: gstBufferManager.h:104
uint64_t mFrameCount
Total number of frames that have been recieved.
Definition: gstBufferManager.h:109
~gstBufferManager()
Destructor.
RingBuffer mTimestamps
Ringbuffer of timestamps that come from appsink.
Definition: gstBufferManager.h:103
uint64_t GetFrameCount() const
Get the total number of frames that have been recieved.
Definition: gstBufferManager.h:97
int Dequeue(void **output, imageFormat format, uint64_t timeout=UINT64_MAX)
Dequeue the next frame.
Thread-safe circular ring buffer queue.
Definition: RingBuffer.h:33
imageFormat GetRawFormat() const
Get raw image format.
Definition: gstBufferManager.h:92
The videoOptions struct contains common settings that are used to configure and query videoSource and...
Definition: videoOptions.h:37
uint64_t mLastTimestamp
Timestamp of the latest dequeued frame.
Definition: gstBufferManager.h:105
bool mNvmmUsed
Is NVMM memory actually used by the stream?
Definition: gstBufferManager.h:110