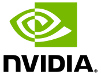 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GL_WIDGET_H__
24 #define __GL_WIDGET_H__
71 bool Contains(
float x,
float y )
const;
76 inline void Move(
float x,
float y ) {
mX += x;
mY += y; }
81 inline float X()
const {
return mX; }
86 inline float Y()
const {
return mY; }
91 inline void SetX(
float x ) {
mX = x; }
96 inline void SetY(
float y ) {
mY = y; }
141 inline void GetSize(
float* width,
float* height )
const {
if(width) *width=
mWidth;
if(height) *height=
mHeight; }
261 void GlobalToLocal(
float x,
float y,
float* x_out,
float* y_out )
const;
266 void LocalToGlobal(
float x,
float y,
float* x_out,
float* y_out )
const;
302 bool OnEvent( uint16_t event,
int a,
int b,
void* user );
int GetIndex() const
Get the index of the widget in the window (or -1 if none)
void SetFillAlpha(float a)
Set fill alpha.
Definition: glWidget.h:161
std::vector< eventHandler > mEventHandlers
Definition: glWidget.h:358
void SetVisible(bool visible)
Show/hide the widget.
Definition: glWidget.h:231
void SetSelected(bool selected)
Select/de-select the widget.
Definition: glWidget.h:221
void setCursor(DragState cursor)
bool OnEvent(uint16_t event, int a, int b, void *user)
bool mResizeable
Definition: glWidget.h:348
void SetFillColor(float r, float g, float b, float a=1.0f)
Set fill color.
Definition: glWidget.h:176
void SetHeight(float height)
Set the widget's height.
Definition: glWidget.h:116
@ DragResizeS
Definition: glWidget.h:315
void GetSize(float *width, float *height) const
Get the widget's size.
Definition: glWidget.h:141
void SetMoveable(bool moveable)
Toggle if the user can move/drag the widget.
Definition: glWidget.h:201
float Width() const
Get the widget's width.
Definition: glWidget.h:101
@ DragResizeE
Definition: glWidget.h:319
DragState mDragState
Definition: glWidget.h:356
OpenGL display window and image/video renderer with CUDA interoperability.
Definition: glDisplay.h:47
void GetPosition(float *x, float *y) const
Get position of widget in global window coordinates.
Definition: glWidget.h:121
void SetShape(Shape shape)
Set the shape.
Definition: glWidget.h:156
bool IsVisible() const
Is the widget visible.
Definition: glWidget.h:226
float mY
Definition: glWidget.h:336
void * GetUserData() const
Retrieve user data.
Definition: glWidget.h:236
float mLineWidth
Definition: glWidget.h:345
@ DragResizeNW
Definition: glWidget.h:313
void SetLineWidth(float width)
Set outline width.
Definition: glWidget.h:171
float mX
Definition: glWidget.h:335
glDisplay * mDisplay
Definition: glWidget.h:355
void RemoveEventHandler(glWidgetEventHandler callback, void *user=NULL)
Remove an event message handler from being called by the widget.
void dispatchEvent(uint16_t msg, int a, int b)
@ DragResizeNE
Definition: glWidget.h:314
void SetResizeable(bool resizeable)
Toggle if the user can resize the widget.
Definition: glWidget.h:211
void SetCoords(float x1, float y1, float x2, float y2)
Set the bounding coordinates of the widget.
Definition: glWidget.h:136
bool mSelected
Definition: glWidget.h:349
void SetSize(float width, float height)
Set the widget's size.
Definition: glWidget.h:146
bool IsSelected() const
Is the widget selected?
Definition: glWidget.h:216
@ DragNone
Definition: glWidget.h:310
void * mUserData
Definition: glWidget.h:353
void SetX(float x)
Set the widget's X coordinate.
Definition: glWidget.h:91
glWidget(Shape shape=Rect)
Constructor.
float mSelectedFillColor[4]
Definition: glWidget.h:340
@ DragMove
Definition: glWidget.h:311
float mSelectedLineColor[4]
Definition: glWidget.h:341
@ DragResizeW
Definition: glWidget.h:318
Shape
Shape enum.
Definition: glWidget.h:46
void GetCoords(float *x1, float *y1, float *x2, float *y2) const
Get the bounding coordinates of the widget.
Definition: glWidget.h:131
void SetSelectedFillColor(float r, float g, float b, float a=1.0f)
Set selected fill color.
Definition: glWidget.h:186
DragState coordToBorder(float x, float y, float max_distance=10.0f)
void SetSelectedLineColor(float r, float g, float b, float a=1.0f)
Set selected outline color.
Definition: glWidget.h:191
float Y() const
Get the widget's Y coordinate.
Definition: glWidget.h:86
float mLineColor[4]
Definition: glWidget.h:344
bool mMoveable
Definition: glWidget.h:347
void Move(float x, float y)
Move the widget's position by the specified offset.
Definition: glWidget.h:76
void LocalToGlobal(float x, float y, float *x_out, float *y_out) const
Convert from local widget offset to global window coordinates.
bool(* glWidgetEventHandler)(glWidget *widget, uint16_t event, int a, int b, void *user)
Event message handler callback for recieving UI messages from widgets.
Definition: glWidget.h:281
bool IsResizeable() const
Is the widget resizeable by the user?
Definition: glWidget.h:206
@ DragResizeSE
Definition: glWidget.h:317
void GlobalToLocal(float x, float y, float *x_out, float *y_out) const
Convert from global window coordinates to local widget offset.
@ Line
Definition: glWidget.h:49
Shape mShape
Definition: glWidget.h:352
@ Rect
Definition: glWidget.h:48
@ Ellipse
Definition: glWidget.h:50
bool mVisible
Definition: glWidget.h:350
DragState
Definition: glWidget.h:308
Shape GetShape() const
Get the shape.
Definition: glWidget.h:151
float mHeight
Definition: glWidget.h:338
bool Contains(float x, float y) const
Test if the point is inside the widget.
void SetLineAlpha(float a)
Set outline alpha.
Definition: glWidget.h:166
void SetLineColor(float r, float g, float b, float a=1.0f)
Set outline color.
Definition: glWidget.h:181
float X() const
Get the widget's X coordinate.
Definition: glWidget.h:81
float mWidth
Definition: glWidget.h:337
@ DragResizeN
Definition: glWidget.h:312
void setDisplay(glDisplay *display)
void AddEventHandler(glWidgetEventHandler callback, void *user=NULL)
Register an event message handler the widget will send events to.
void SetWidth(float width)
Set the widget's width.
Definition: glWidget.h:111
virtual ~glWidget()
Destructor.
void SetPosition(float x, float y)
Set position of widget in global window coordinates.
Definition: glWidget.h:126
@ DragResizeSW
Definition: glWidget.h:316
void SetY(float y)
Set the widget's Y coordinate.
Definition: glWidget.h:96
glDisplay * GetDisplay() const
Get the root window of the widget.
Definition: glWidget.h:246
float Height() const
Get the widget's height.
Definition: glWidget.h:106
bool IsMoveable() const
Is the widget moveable/draggable by the user?
Definition: glWidget.h:196
float mFillColor[4]
Definition: glWidget.h:343
void SetUserData(void *user)
Set user-defined data.
Definition: glWidget.h:241
void Render()
Render (automatically called by parent)