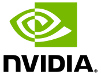 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __GL_BUFFER_H__
24 #define __GL_BUFFER_H__
28 #include "cuda_gl_interop.h"
35 #define GL_VERTEX_BUFFER GL_ARRAY_BUFFER
41 #define GL_INDEX_BUFFER GL_ELEMENT_ARRAY_BUFFER
47 #define GL_MAP_CPU 0x1
53 #define GL_MAP_CUDA 0x2
59 #define GL_FROM_CPU 0x3
65 #define GL_FROM_CUDA 0x4
77 #define GL_TO_CUDA 0x6
83 #define GL_WRITE_DISCARD (GL_READ_WRITE + 0xff)
90 #define GL_WRITE_ONLY GL_WRITE_ONLY_ARB
98 #define GL_READ_ONLY GL_READ_ONLY_ARB
105 #ifndef GL_READ_WRITE
106 #define GL_READ_WRITE GL_READ_WRITE_ARB
124 static glBuffer*
Create( uint32_t type, uint32_t size,
void* data=NULL, uint32_t usage=GL_STATIC_DRAW );
134 static glBuffer*
Create( uint32_t type, uint32_t numElements, uint32_t elementSize,
void* data=NULL, uint32_t usage=GL_STATIC_DRAW );
154 inline uint32_t
GetID()
const {
return mID; }
159 inline uint32_t
GetType()
const {
return mType; }
164 inline uint32_t
GetSize()
const {
return mSize; }
197 void*
Map( uint32_t device, uint32_t flags );
222 bool Copy(
void* ptr, uint32_t flags );
240 bool Copy(
void* ptr, uint32_t size, uint32_t flags );
262 bool Copy(
void* ptr, uint32_t offset, uint32_t size, uint32_t flags );
267 bool init( uint32_t type, uint32_t size,
void* data, uint32_t usage);
274 uint32_t mNumElements;
275 uint32_t mElementSize;
280 cudaGraphicsResource* mInteropCUDA;
static glBuffer * Create(uint32_t type, uint32_t size, void *data=NULL, uint32_t usage=GL_STATIC_DRAW)
Allocate an OpenGL buffer.
bool Copy(void *ptr, uint32_t flags)
Copy entire contents of the buffer to/from CPU or CUDA memory.
void * Map(uint32_t device, uint32_t flags)
Map the buffer for accessing from the CPU or CUDA.
uint32_t GetSize() const
Retrieve the total size in bytes of the buffer.
Definition: glBuffer.h:164
~glBuffer()
Free the buffer.
uint32_t GetType() const
Retrieve the buffer type (GL_VERTEX_BUFFER or GL_INDEX_BUFFER)
Definition: glBuffer.h:159
bool Bind()
Activate using the buffer.
uint32_t GetElementSize() const
Retrieve the size in bytes of each element.
Definition: glBuffer.h:180
void Unbind()
Deactivate using the buffer.
void Unmap()
Unmap the buffer from CPU/CUDA access.
OpenGL buffer with CUDA interoperability.
Definition: glBuffer.h:114
uint32_t GetID() const
Retrieve the OpenGL resource handle of the buffer.
Definition: glBuffer.h:154
uint32_t GetNumElements() const
Retrieve the number of elements (i.e.
Definition: glBuffer.h:172