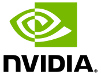 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __FILESYSTEM_UTIL_H__
24 #define __FILESYSTEM_UTIL_H__
43 std::string
absolutePath(
const std::string& relative_path );
56 std::string
locateFile(
const std::string& path );
67 std::string
locateFile(
const std::string& path, std::vector<std::string>& locations );
77 std::string
readFile(
const std::string& path );
84 std::string
pathJoin(
const std::string& a,
const std::string& b );
91 std::string
pathDir(
const std::string& path );
104 std::pair<std::string, std::string>
splitPath(
const std::string& path );
136 bool listDir(
const std::string& path, std::vector<std::string>& list, uint32_t mask=0 );
146 bool fileExists(
const std::string& path, uint32_t mask=0 );
154 bool fileIsType(
const std::string& path, uint32_t mask );
161 uint32_t
fileType(
const std::string& path );
172 size_t fileSize(
const std::string& path );
187 bool fileHasExtension(
const std::string& path,
const std::string& extension );
193 bool fileHasExtension(
const std::string& path,
const std::vector<std::string>& extensions );
217 std::string
fileChangeExtension(
const std::string& filename,
const std::string& newExtension );
bool fileExists(const std::string &path, uint32_t mask=0)
Return the directory /** Verify path and return true if the file exists.
@ FILE_REGULAR
Definition: filesystem.h:113
std::string pathFilename(const std::string &path)
Return the filename from the path, including the file extension.
@ FILE_BLOCK
Definition: filesystem.h:117
bool fileIsType(const std::string &path, uint32_t mask)
Return true if the file is one of the types in the fileTypes mask.
std::pair< std::string, std::string > splitPath(const std::string &path)
Split a path into directory and filename components.
std::string fileChangeExtension(const std::string &filename, const std::string &newExtension)
Return the input string with a changed file extension For example, fileChangeExtension("~/workspace/s...
@ FILE_FIFO
Definition: filesystem.h:118
std::string pathJoin(const std::string &a, const std::string &b)
Join two paths, and properly include a path separator (/) as needed.
@ FILE_MISSING
Definition: filesystem.h:112
@ FILE_SOCKET
Definition: filesystem.h:119
size_t fileSize(const std::string &path)
Return the size (in bytes) of the specified file.
@ FILE_CHAR
Definition: filesystem.h:116
@ FILE_LINK
Definition: filesystem.h:115
std::string pathDir(const std::string &path)
Return the parent directory of the specified path, removing the filename and extension.
std::string absolutePath(const std::string &relative_path)
Given a relative path, resolve the absolute path using the working directory.
uint32_t fileType(const std::string &path)
Return the file type, or FILE_MISSING if it doesn't exist.
std::string fileRemoveExtension(const std::string &filename)
Return the input string with the file extension removed For example, fileRemoveExtension("~/workspace...
@ FILE_DIR
Definition: filesystem.h:114
std::string locateFile(const std::string &path)
Locate a file from common system locations.
bool fileHasExtension(const std::string &path, const std::string &extension)
Return true if the file has the given extension, otherwise false.
std::string readFile(const std::string &path)
Read a text file into a string.
std::string fileExtension(const std::string &path)
Extract the file extension from the path.
bool listDir(const std::string &path, std::vector< std::string > &list, uint32_t mask=0)
Return a sorted list of the files in the specified directory.
fileTypes
File types.
Definition: filesystem.h:110