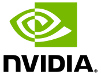 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __DEPTH_NET_H__
24 #define __DEPTH_NET_H__
34 #define DEPTHNET_DEFAULT_INPUT "input_0"
40 #define DEPTHNET_DEFAULT_OUTPUT "output_0"
46 #define DEPTHNET_MODEL_TYPE "monodepth"
52 #define DEPTHNET_USAGE_STRING "depthNet arguments: \n" \
53 " --network NETWORK pre-trained model to load, one of the following:\n" \
54 " * fcn-mobilenet\n" \
57 " --model MODEL path to custom model to load (onnx)\n" \
58 " --input_blob INPUT name of the input layer (default is '" DEPTHNET_DEFAULT_INPUT "')\n" \
59 " --output_blob OUTPUT name of the output layer (default is '" DEPTHNET_DEFAULT_OUTPUT "')\n" \
60 " --profile enable layer profiling in TensorRT\n\n"
118 static depthNet*
Create(
const char* model_path,
const char* input,
119 const Dims3& inputDims,
const char* output,
148 template<
typename T>
bool Process( T* image, uint32_t width, uint32_t height ) {
return Process((
void*)image, width, height, imageFormatFromType<T>()); }
160 template<
typename T1,
typename T2>
161 bool Process( T1* input, T2* output, uint32_t width, uint32_t height,
163 cudaFilterMode filter=
FILTER_LINEAR ) {
return Process((
void*)input, imageFormatFromType<T1>(), (
void*)output, imageFormatFromType<T2>(), width, height, colormap, filter); }
171 uint32_t width, uint32_t height,
179 template<
typename T1,
typename T2>
180 bool Process( T1* input, uint32_t input_width, uint32_t input_height,
181 T2* output, uint32_t output_width, uint32_t output_height,
183 cudaFilterMode filter=
FILTER_LINEAR ) {
return Process((
void*)input, input_width, input_height, imageFormatFromType<T1>(), (
void*)output, output_width, output_height, imageFormatFromType<T2>(), colormap, filter); }
189 bool Process(
void* input, uint32_t input_width, uint32_t input_height,
imageFormat input_format,
190 void* output, uint32_t output_width, uint32_t output_height,
imageFormat output_format,
199 bool Visualize( T* output, uint32_t width, uint32_t height,
236 bool SavePointCloud(
const char* filename,
float* rgba, uint32_t width, uint32_t height );
242 bool SavePointCloud(
const char* filename,
float* rgba, uint32_t width, uint32_t height,
243 const float2& focalLength,
const float2& principalPoint );
249 bool SavePointCloud(
const char* filename,
float* rgba, uint32_t width, uint32_t height,
250 const float intrinsicCalibration[3][3] );
256 bool SavePointCloud(
const char* filename,
float* rgba, uint32_t width, uint32_t height,
257 const char* intrinsicCalibrationPath );
274 #define DEPTH_FLOAT_TO_INT 1000000
277 #define DEPTH_HISTOGRAM_BINS 256
#define DEPTHNET_DEFAULT_OUTPUT
Name of default output blob for depthNet model.
Definition: depthNet.h:40
virtual ~depthNet()
Destroy.
bool histogramEqualization()
bool Process(T1 *input, T2 *output, uint32_t width, uint32_t height, cudaColormapType colormap=COLORMAP_VIRIDIS_INVERTED, cudaFilterMode filter=FILTER_LINEAR)
Process an RGB/RGBA image and map the depth image with the specified colormap.
Definition: depthNet.h:161
#define DEPTHNET_USAGE_STRING
Command-line options able to be passed to depthNet::Create()
Definition: depthNet.h:52
float * mDepthEqualized
Definition: depthNet.h:267
@ FILTER_LINEAR
Bilinear filtering.
Definition: cudaFilterMode.h:38
bool SavePointCloud(const char *filename)
Extract and save the point cloud to a PCD file (depth only).
float * mHistogramPDF
Definition: depthNet.h:269
@ COLORMAP_VIRIDIS_INVERTED
Viridis colormap (inverted), see http://bids.github.io/colormap/.
Definition: cudaColormap.h:52
uint32_t GetDepthFieldHeight() const
Return the height of the depth field.
Definition: depthNet.h:224
static uint32_t VisualizationFlagsFromStr(const char *str, uint32_t default_value=VISUALIZE_INPUT|VISUALIZE_DEPTH)
Parse a string of one of more VisualizationMode values.
uint32_t * mHistogramEDU
Definition: depthNet.h:271
float * mHistogramCDF
Definition: depthNet.h:270
bool Process(T *image, uint32_t width, uint32_t height)
Compute the depth field from a monocular RGB/RGBA image.
Definition: depthNet.h:148
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
nvinfer1::Dims3 Dims3
Definition: tensorNet.h:58
bool allocHistogramBuffers()
@ COLORMAP_DEFAULT
Definition: cudaColormap.h:60
bool Visualize(T *output, uint32_t width, uint32_t height, cudaColormapType colormap=COLORMAP_DEFAULT, cudaFilterMode filter=FILTER_LINEAR)
Visualize the raw depth field into a colorized RGB/RGBA depth map.
Definition: depthNet.h:199
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
float * GetDepthField() const
Return the raw depth field.
Definition: depthNet.h:214
@ VISUALIZE_DEPTH
Display the colorized depth field.
Definition: depthNet.h:76
#define DEPTHNET_DEFAULT_INPUT
Name of default input blob for depthNet model.
Definition: depthNet.h:34
#define DIMS_H(x)
Definition: tensorNet.h:61
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
bool Process(T1 *input, uint32_t input_width, uint32_t input_height, T2 *output, uint32_t output_width, uint32_t output_height, cudaColormapType colormap=COLORMAP_DEFAULT, cudaFilterMode filter=FILTER_LINEAR)
Process an RGB/RGBA image and map the depth image with the specified colormap.
Definition: depthNet.h:180
cudaFilterMode
Enumeration of interpolation filtering modes.
Definition: cudaFilterMode.h:35
@ VISUALIZE_INPUT
Display the original input image.
Definition: depthNet.h:75
static depthNet * Create(const char *network="fcn-mobilenet", uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load a pre-trained model.
static const char * Usage()
Usage string for command line arguments to Create()
Definition: depthNet.h:137
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
Mono depth estimation from monocular images, using TensorRT.
Definition: depthNet.h:67
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
#define DIMS_W(x)
Definition: tensorNet.h:62
int2 * mDepthRange
Definition: depthNet.h:266
cudaColormapType
Enumeration of built-in colormaps.
Definition: cudaColormap.h:36
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
uint32_t * mHistogram
Definition: depthNet.h:268
bool histogramEqualizationCUDA()
VisualizationFlags
Visualization flags.
Definition: depthNet.h:73
std::vector< layerInfo > mOutputs
Definition: tensorNet.h:819
uint32_t GetDepthFieldWidth() const
Return the width of the depth field.
Definition: depthNet.h:219