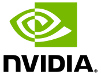 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CUDA_UTILITY_H_
24 #define __CUDA_UTILITY_H_
27 #include <cuda_runtime.h>
41 #define CUDA(x) cudaCheckError((x), #x, __FILE__, __LINE__)
47 #define CUDA_SUCCESS(x) (CUDA(x) == cudaSuccess)
53 #define CUDA_FAILED(x) (CUDA(x) != cudaSuccess)
59 #define CUDA_VERIFY(x) if(CUDA_FAILED(x)) return false;
65 #define LOG_CUDA "[cuda] "
79 inline cudaError_t
cudaCheckError(cudaError_t retval,
const char* txt,
const char* file,
int line )
81 #if !defined(CUDA_TRACE)
82 if( retval == cudaSuccess)
91 if( retval == cudaSuccess )
100 if( retval != cudaSuccess )
102 LogError(
LOG_CUDA " %s (error %u) (hex 0x%02X)\n", cudaGetErrorString(retval), retval, retval);
114 #define CUDA_FREE(x) if(x != NULL) { cudaFree(x); x = NULL; }
120 #define CUDA_FREE_HOST(x) if(x != NULL) { cudaFreeHost(x); x = NULL; }
126 #define SAFE_DELETE(x) if(x != NULL) { delete x; x = NULL; }
132 #define SAFE_FREE(x) if(x != NULL) { free(x); x = NULL; }
152 inline __device__ __host__
int iDivUp(
int a,
int b ) {
return (a % b != 0) ? (a / b + 1) : (a / b); }
cudaError_t cudaCheckError(cudaError_t retval, const char *txt, const char *file, int line)
cudaCheckError
Definition: cudaUtility.h:79
__device__ __host__ int iDivUp(int a, int b)
If a / b has a remainder, round up.
Definition: cudaUtility.h:152
#define LOG_CUDA
LOG_CUDA string.
Definition: cudaUtility.h:65
#define LogError(format, args...)
Log a printf-style error message (Log::ERROR)
Definition: logging.h:150
#define LogDebug(format, args...)
Log a printf-style debug message (Log::DEBUG)
Definition: logging.h:180