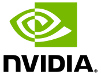 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CUDA_FONT_H__
24 #define __CUDA_FONT_H__
72 static cudaFont*
Create(
const std::vector<std::string>& fonts,
float size );
83 uint32_t width, uint32_t height,
84 const char* str,
int x,
int y,
87 int backgroundPadding=5 );
93 uint32_t width, uint32_t height,
94 const std::vector< std::pair< std::string, int2 > >& text,
97 int backgroundPadding=5 );
102 template<
typename T>
bool OverlayText( T* image, uint32_t width, uint32_t height,
103 const char* str,
int x,
int y,
106 int backgroundPadding=5 )
108 return OverlayText(image, imageFormatFromType<T>(), width, height, str, x, y,
color, background, backgroundPadding);
114 template<
typename T>
bool OverlayText( T* image, uint32_t width, uint32_t height,
115 const std::vector< std::pair< std::string, int2 > >& text,
118 int backgroundPadding=5 )
120 return OverlayText(image, imageFormatFromType<T>(), width, height, text,
color, background, backgroundPadding);
131 int4
TextExtents(
const char* str,
int x=0,
int y=0 );
136 bool init(
const char* font,
float size );
bool OverlayText(T *image, uint32_t width, uint32_t height, const char *str, int x, int y, const float4 &color=make_float4(0, 0, 0, 255), const float4 &background=make_float4(0, 0, 0, 0), int backgroundPadding=5)
Render text overlay onto image.
Definition: cudaFont.h:102
static cudaFont * Create(float size=32.0f)
Create new CUDA font overlay object using baked fonts.
bool OverlayText(T *image, uint32_t width, uint32_t height, const std::vector< std::pair< std::string, int2 > > &text, const float4 &color=make_float4(0, 0, 0, 255), const float4 &background=make_float4(0, 0, 0, 0), int backgroundPadding=5)
Render text overlay onto image.
Definition: cudaFont.h:114
static const uint32_t FirstGlyph
Definition: cudaFont.h:155
uchar3 color
The RGB color of the point.
Definition: cudaPointCloud.h:11
int mRectIndex
Definition: cudaFont.h:152
float GetSize() const
Return the size of the font (height in pixels)
Definition: cudaFont.h:126
void * mCommandCPU
Definition: cudaFont.h:146
bool init(const char *font, float size)
uint16_t width
Definition: cudaFont.h:163
static const uint32_t NumGlyphs
Definition: cudaFont.h:157
Definition: cudaFont.h:159
int mFontMapHeight
Definition: cudaFont.h:144
static const uint32_t LastGlyph
Definition: cudaFont.h:156
int4 TextExtents(const char *str, int x=0, int y=0)
Return the bounding rectangle of the given text string.
float adaptFontSize(uint32_t dimension)
Determine an appropriate font size given a particular dimension to use (typically an image's width).
float4 * mRectsCPU
Definition: cudaFont.h:150
uint8_t * mFontMapCPU
Definition: cudaFont.h:140
struct cudaFont::GlyphInfo mGlyphInfo[NumGlyphs]
uint8_t * mFontMapGPU
Definition: cudaFont.h:141
uint16_t y
Definition: cudaFont.h:162
uint16_t x
Definition: cudaFont.h:161
float xAdvance
Definition: cudaFont.h:166
float4 * mRectsGPU
Definition: cudaFont.h:151
__host__ __device__ float4 make_float4(float s)
Definition: cudaMath.h:248
TTF font rasterization and image overlay rendering using CUDA.
Definition: cudaFont.h:49
float mSize
Definition: cudaFont.h:138
void * mCommandGPU
Definition: cudaFont.h:147
bool OverlayText(void *image, imageFormat format, uint32_t width, uint32_t height, const char *str, int x, int y, const float4 &color=make_float4(0, 0, 0, 255), const float4 &background=make_float4(0, 0, 0, 0), int backgroundPadding=5)
Render text overlay onto image.
float xOffset
Definition: cudaFont.h:167
uint16_t height
Definition: cudaFont.h:164
float yOffset
Definition: cudaFont.h:168
static const uint32_t MaxCommands
Definition: cudaFont.h:154
int mCmdIndex
Definition: cudaFont.h:148
int mFontMapWidth
Definition: cudaFont.h:143