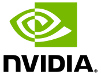 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CUDA_COLORMAP_H__
24 #define __CUDA_COLORMAP_H__
87 size_t width,
size_t height,
91 cudaStream_t stream=NULL) {
return cudaColormap(input, (
void*)output, width, height, input_range, input_format, imageFormatFromType<T>(), colormap, stream); }
103 size_t width,
size_t height,
108 cudaStream_t stream=NULL);
121 cudaError_t
cudaColormap(
float* input,
size_t input_width,
size_t input_height,
122 T* output,
size_t output_width,
size_t output_height,
127 cudaStream_t stream=NULL ) {
return cudaColormap(input, input_width, input_height, output, output_width, output_height, input_range, input_format, imageFormatFromType<T>(), colormap, filter, stream); }
139 cudaError_t
cudaColormap(
float* input,
size_t input_width,
size_t input_height,
140 void* output,
size_t output_width,
size_t output_height,
146 cudaStream_t stream=NULL );
@ COLORMAP_PLASMA
Plasma colormap, see http://bids.github.io/colormap/.
Definition: cudaColormap.h:42
@ COLORMAP_PLASMA_INVERTED
Plasma colormap (inverted), see http://bids.github.io/colormap/.
Definition: cudaColormap.h:50
@ COLORMAP_INFERNO_INVERTED
Inferno colormap (inverted), see http://bids.github.io/colormap/.
Definition: cudaColormap.h:47
@ COLORMAP_PARULA_INVERTED
Parula colormap (inverted), see https://www.mathworks.com/help/matlab/ref/parula.html.
Definition: cudaColormap.h:49
cudaDataFormat
Enumeration of image layout formats.
Definition: cudaFilterMode.h:59
@ FILTER_LINEAR
Bilinear filtering.
Definition: cudaFilterMode.h:38
@ COLORMAP_NONE
Pass-through (no remapping applied)
Definition: cudaColormap.h:56
@ COLORMAP_VIRIDIS_INVERTED
Viridis colormap (inverted), see http://bids.github.io/colormap/.
Definition: cudaColormap.h:52
@ COLORMAP_VIRIDIS
Viridis colormap, see http://bids.github.io/colormap/.
Definition: cudaColormap.h:44
@ COLORMAP_INFERNO
Inferno colormap, see http://bids.github.io/colormap/.
Definition: cudaColormap.h:39
@ COLORMAP_DEFAULT
Definition: cudaColormap.h:60
const char * cudaColormapToStr(cudaColormapType colormap)
Convert a cudaColormapType enum to a string.
cudaError_t cudaColormapFree()
Free the colormap palettes after they are done being used.
cudaFilterMode
Enumeration of interpolation filtering modes.
Definition: cudaFilterMode.h:35
@ COLORMAP_LINEAR
Linearly remap the values to [0,255].
Definition: cudaColormap.h:57
@ COLORMAP_TURBO
Turbo colormap, see https://ai.googleblog.com/2019/08/turbo-improved-rainbow-colormap-for....
Definition: cudaColormap.h:43
float4 * cudaColormapPalette(cudaColormapType colormap)
Retrieve a CUDA device pointer to one of the colormaps palettes.
cudaError_t cudaColormapInit()
Initialize the colormap palettes by allocating them in CUDA memory.
@ COLORMAP_PARULA
Parula colormap, see https://www.mathworks.com/help/matlab/ref/parula.html.
Definition: cudaColormap.h:41
__host__ __device__ float2 make_float2(float s)
Definition: cudaMath.h:81
cudaColormapType
Enumeration of built-in colormaps.
Definition: cudaColormap.h:36
cudaColormapType cudaColormapFromStr(const char *colormap)
Parse a cudaColormapType enum from a string.
@ COLORMAP_MAGMA_INVERTED
Magma colormap (inverted), see http://bids.github.io/colormap/.
Definition: cudaColormap.h:48
@ COLORMAP_TURBO_INVERTED
Turbo colormap (inverted), see https://ai.googleblog.com/2019/08/turbo-improved-rainbow-colormap-for....
Definition: cudaColormap.h:51
cudaError_t cudaColormap(float *input, T *output, size_t width, size_t height, const float2 &input_range=make_float2(0, 255), cudaDataFormat input_format=FORMAT_DEFAULT, cudaColormapType colormap=COLORMAP_DEFAULT, cudaStream_t stream=NULL)
Apply a colormap from an input image or vector field to RGB/RGBA.
Definition: cudaColormap.h:86
@ FORMAT_DEFAULT
Definition: cudaFilterMode.h:65
@ COLORMAP_FLOW
Optical flow x/y velocity (2D)
Definition: cudaColormap.h:55
@ COLORMAP_MAGMA
Magma colormap, see http://bids.github.io/colormap/.
Definition: cudaColormap.h:40