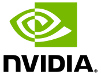 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CSV_WRITER_H_
24 #define __CSV_WRITER_H_
46 csvWriter(
const char* filename,
const char* delimiter=
", " );
50 inline static csvWriter*
Open(
const char* filename,
const char* delimiter=
", " );
57 inline bool IsOpen()
const;
68 template<
typename T,
typename... Args>
72 template<
typename T,
typename... Args>
93 std::string mFilename;
94 std::string mDelimiter;
void EndLine()
Definition: csvWriter.hpp:104
bool IsClosed() const
Definition: csvWriter.hpp:98
csv stream manipulators
Definition: csvWriter.h:103
csvWriter & Write(const T &value)
Definition: csvWriter.hpp:112
void Flush()
Definition: csvWriter.hpp:86
const char * GetDelimiter() const
Definition: csvWriter.hpp:162
static csvWriter * Open(const char *filename, const char *delimiter=", ")
Definition: csvWriter.hpp:59
csvWriter & WriteLine(const T &value, const Args &... args)
Definition: csvWriter.hpp:134
const char * GetFilename() const
Definition: csvWriter.hpp:168
void Close()
Definition: csvWriter.hpp:76
void SetDelimiter(const char *delimiters)
Definition: csvWriter.hpp:156
csvWriter(const char *filename, const char *delimiter=", ")
Definition: csvWriter.hpp:32
csvWriter
Definition: csvWriter.h:42
csvWriter & operator<<(const T &value)
Definition: csvWriter.hpp:144
~csvWriter()
Definition: csvWriter.hpp:52
bool IsOpen() const
Definition: csvWriter.hpp:92