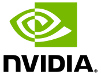 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CSV_READER_HPP_
24 #define __CSV_READER_HPP_
37 const int x = strtol(
string.c_str(), &e, 0);
39 if( *e !=
'\0' || errno != 0 )
54 const float x = strtof(
string.c_str(), &e);
56 if( *e !=
'\0' || errno != 0 )
71 const float x = strtof(
string.c_str(), &e);
73 if( *e !=
'\0' || errno != 0 )
86 const bool v=
toInt(&x);
133 inline std::vector<csvData>
csvData::Parse(
const char* str,
const char* delimiters )
135 std::vector<csvData> tokens;
136 Parse(tokens, str, delimiters);
141 inline bool csvData::Parse( std::vector<csvData>& tokens,
const char* str,
const char* delimiters )
143 if( !str || !delimiters )
148 const size_t str_length = strlen(str);
149 char* str_tokens = (
char*)malloc(str_length + 1);
154 strcpy(str_tokens, str);
156 if( str_tokens[str_length] ==
'\n' )
157 str_tokens[str_length] =
'\0';
159 if( str_tokens[str_length-1] ==
'\n' )
160 str_tokens[str_length-1] =
'\0';
162 char* token = strtok(str_tokens, delimiters);
164 while( token != NULL )
166 tokens.push_back(token);
167 token = strtok(NULL, delimiters);
171 return tokens.size() > 0;
178 if( !filename || !delimiters )
181 mFile = fopen(filename,
"r");
185 LogError(
"csvReader -- failed to open file %s\n", filename);
186 perror(
"csvReader -- error");
190 mFilename = filename;
191 mDelimiters = delimiters;
204 if( !filename || !delimiters )
231 return mFile != NULL;
243 return Read(mDelimiters.c_str());
249 std::vector<csvData> tokens;
250 Read(tokens, delimiters);
256 return Read(data, mDelimiters.c_str());
268 if( fgets(str,
sizeof(str), mFile) == NULL )
272 LogError(
"csvReader -- error reading file %s\n", mFilename.c_str());
273 perror(
"csvReader -- error");
281 if( feof(mFile) == EOF )
286 return Read(data, delimiters);
294 mDelimiters = delimiters;
300 return mDelimiters.c_str();
306 return mFilename.c_str();
bool toInt(int *value) const
Definition: csvReader.hpp:32
csvReader
Definition: csvReader.h:90
bool toFloat(float *value) const
Definition: csvReader.hpp:49
csv stream manipulators
Definition: csvWriter.h:103
void SetDelimiters(const char *delimiters)
Definition: csvReader.hpp:292
bool IsOpen() const
Definition: csvReader.hpp:229
csvReader(const char *filename, const char *delimiters=",;\t ")
Definition: csvReader.hpp:176
std::ostream & operator<<(std::ostream &out, const csvData &obj)
Definition: csvReader.hpp:126
const size_t MaxLineLength
Definition: csvReader.h:125
std::istream & operator>>(std::istream &in, csvData &obj)
Definition: csvReader.hpp:119
bool toDouble(double *value) const
Definition: csvReader.hpp:66
bool IsClosed() const
Definition: csvReader.hpp:235
void Close()
Definition: csvReader.hpp:219
const char * GetDelimiters() const
Definition: csvReader.hpp:298
~csvReader()
Definition: csvReader.hpp:195
const char * GetFilename() const
Definition: csvReader.hpp:304
static csvReader * Open(const char *filename, const char *delimiters=",;\t ")
Definition: csvReader.hpp:202
static std::vector< csvData > Parse(const char *str, const char *delimiters=",;\t ")
Definition: csvReader.hpp:133
std::string string
Definition: csvReader.h:82
std::vector< csvData > Read()
Definition: csvReader.hpp:241
#define LogError(format, args...)
Log a printf-style error message (Log::ERROR)
Definition: logging.h:150
csvData
Definition: csvReader.h:39