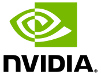 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __CSV_READER_H_
24 #define __CSV_READER_H_
44 csvData(
const char* str ) {
string = str; }
45 csvData( std::string& str ) {
string = str; }
49 template<
typename T>
void set( T value ) {
string = std::to_string(value); }
52 inline operator std::string&() {
return string; }
53 inline operator const std::string&()
const {
return string; }
54 inline operator const char*()
const {
return string.c_str(); }
57 inline operator int()
const {
return toInt(); }
58 inline operator float()
const {
return toFloat(); }
59 inline operator double()
const {
return toDouble(); }
62 inline bool toInt(
int* value )
const;
63 inline bool toFloat(
float* value )
const;
64 inline bool toDouble(
double* value )
const;
67 inline int toInt(
bool* valid=NULL )
const;
68 inline float toFloat(
bool* valid=NULL )
const;
69 inline double toDouble(
bool* valid=NULL )
const;
76 inline static std::vector<csvData>
Parse(
const char* str,
const char* delimiters=
",;\t " );
79 inline static bool Parse( std::vector<csvData>& data,
const char* str,
const char* delimiters=
",;\t " );
94 csvReader(
const char* filename,
const char* delimiters=
",;\t " );
98 inline static csvReader*
Open(
const char* filename,
const char* delimiters=
",;\t " );
104 inline bool IsOpen()
const;
108 inline std::vector<csvData>
Read();
109 inline std::vector<csvData>
Read(
const char* delimiters );
112 inline bool Read( std::vector<csvData>& data );
113 inline bool Read( std::vector<csvData>& data,
const char* delimiters );
130 std::string mFilename;
131 std::string mDelimiters;
bool toInt(int *value) const
Definition: csvReader.hpp:32
csvReader
Definition: csvReader.h:90
bool toFloat(float *value) const
Definition: csvReader.hpp:49
void SetDelimiters(const char *delimiters)
Definition: csvReader.hpp:292
bool IsOpen() const
Definition: csvReader.hpp:229
csvReader(const char *filename, const char *delimiters=",;\t ")
Definition: csvReader.hpp:176
const size_t MaxLineLength
Definition: csvReader.h:125
csvData(std::string &str)
Definition: csvReader.h:45
csvData(const char *str)
Definition: csvReader.h:44
bool toDouble(double *value) const
Definition: csvReader.hpp:66
bool IsClosed() const
Definition: csvReader.hpp:235
void set(T value)
Definition: csvReader.h:49
void Close()
Definition: csvReader.hpp:219
csvData(T value)
Definition: csvReader.h:48
const char * GetDelimiters() const
Definition: csvReader.hpp:298
~csvReader()
Definition: csvReader.hpp:195
csvData(char *str)
Definition: csvReader.h:43
const char * GetFilename() const
Definition: csvReader.hpp:304
static csvReader * Open(const char *filename, const char *delimiters=",;\t ")
Definition: csvReader.hpp:202
friend std::ostream & operator<<(std::ostream &out, const csvData &obj)
Definition: csvReader.hpp:126
static std::vector< csvData > Parse(const char *str, const char *delimiters=",;\t ")
Definition: csvReader.hpp:133
friend std::istream & operator>>(std::istream &in, csvData &obj)
Definition: csvReader.hpp:119
std::string string
Definition: csvReader.h:82
std::vector< csvData > Read()
Definition: csvReader.hpp:241
csvData
Definition: csvReader.h:39