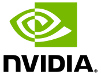 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __BACKGROUND_NET_H__
24 #define __BACKGROUND_NET_H__
35 #define BACKGROUNDNET_DEFAULT_INPUT "input_0"
41 #define BACKGROUNDNET_DEFAULT_OUTPUT "output_0"
47 #define BACKGROUNDNET_MODEL_TYPE "background"
53 #define BACKGROUNDNET_USAGE_STRING "backgroundNet arguments: \n" \
54 " --network=NETWORK pre-trained model to load, one of the following:\n" \
55 " * u2net (default)\n" \
56 " --model=MODEL path to custom model to load (caffemodel, uff, or onnx)\n" \
57 " --input-blob=INPUT name of the input layer (default is '" BACKGROUNDNET_DEFAULT_INPUT "')\n" \
58 " --output-blob=OUTPUT name of the output layer (default is '" BACKGROUNDNET_DEFAULT_OUTPUT "')\n" \
59 " --profile enable layer profiling in TensorRT\n\n"
118 template<
typename T>
int Process( T* image, uint32_t width, uint32_t height,
131 template<
typename T>
int Process( T* input, T* output, uint32_t width, uint32_t height,
132 cudaFilterMode filter=
FILTER_LINEAR,
bool maskAlpha=
true ) {
return Process((
void*)input, (
void*)output, width, height, imageFormatFromType<T>(), filter, maskAlpha); }
156 bool Process(
void* input,
void* output, uint32_t width, uint32_t height,
imageFormat format,
162 bool init(
const char* model_path,
const char* input,
const char* output, uint32_t maxBatchSize,
precisionType precision,
deviceType device,
bool allowGPUFallback );
static backgroundNet * Create(const char *network="u2net", uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load a pre-trained model.
bool Process(void *image, uint32_t width, uint32_t height, imageFormat format, cudaFilterMode filter=FILTER_LINEAR, bool maskAlpha=true)
Perform background subtraction/removal on the image (in-place).
Definition: backgroundNet.h:143
Background subtraction/removal with DNNs, using TensorRT.
Definition: backgroundNet.h:66
@ FILTER_LINEAR
Bilinear filtering.
Definition: cudaFilterMode.h:38
virtual ~backgroundNet()
Destroy.
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
int Process(T *input, T *output, uint32_t width, uint32_t height, cudaFilterMode filter=FILTER_LINEAR, bool maskAlpha=true)
Perform background subtraction/removal on the image.
Definition: backgroundNet.h:131
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
#define BACKGROUNDNET_USAGE_STRING
Standard command-line options able to be passed to backgroundNet::Create()
Definition: backgroundNet.h:53
#define BACKGROUNDNET_DEFAULT_OUTPUT
Name of default output layer for backgroundNet model.
Definition: backgroundNet.h:41
cudaFilterMode
Enumeration of interpolation filtering modes.
Definition: cudaFilterMode.h:35
#define BACKGROUNDNET_DEFAULT_INPUT
Name of default input layer for backgroundNet model.
Definition: backgroundNet.h:35
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
static const char * Usage()
Usage string for command line arguments to Create()
Definition: backgroundNet.h:102
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
int Process(T *image, uint32_t width, uint32_t height, cudaFilterMode filter=FILTER_LINEAR, bool maskAlpha=true)
Perform background subtraction/removal on the image (in-place).
Definition: backgroundNet.h:118
bool init(const char *model_path, const char *input, const char *output, uint32_t maxBatchSize, precisionType precision, deviceType device, bool allowGPUFallback)
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35