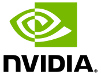 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __ACTION_NET_H__
24 #define __ACTION_NET_H__
34 #define ACTIONNET_DEFAULT_INPUT "input"
40 #define ACTIONNET_DEFAULT_OUTPUT "output"
46 #define ACTIONNET_MODEL_TYPE "action"
52 #define ACTIONNET_USAGE_STRING "actionNet arguments: \n" \
53 " --network=NETWORK pre-trained model to load, one of the following:\n" \
54 " * resnet-18 (default)\n" \
56 " --model=MODEL path to custom model to load (.onnx)\n" \
57 " --labels=LABELS path to text file containing the labels for each class\n" \
58 " --input-blob=INPUT name of the input layer (default is '" ACTIONNET_DEFAULT_INPUT "')\n" \
59 " --output-blob=OUTPUT name of the output layer (default is '" ACTIONNET_DEFAULT_OUTPUT "')\n" \
60 " --threshold=CONF minimum confidence threshold for classification (default is 0.01)\n" \
61 " --skip-frames=SKIP how many frames to skip between classifications (default is 1)\n" \
62 " --profile enable layer profiling in TensorRT\n\n"
77 bool allowGPUFallback=
true );
89 static actionNet*
Create(
const char* model_path,
const char* class_labels,
130 template<
typename T>
int Classify( T* image, uint32_t width, uint32_t height,
float* confidence=NULL ) {
return Classify((
void*)image, width, height, imageFormatFromType<T>(), confidence); }
146 int Classify(
void* image, uint32_t width, uint32_t height,
imageFormat format,
float* confidence=NULL );
200 bool init(
const char* model_path,
const char* class_path,
const char* input,
const char* output, uint32_t maxBatchSize,
precisionType precision,
deviceType device,
bool allowGPUFallback );
const char * GetClassLabel(int index) const
Retrieve the description of a particular class.
Definition: actionNet.h:156
bool preProcess(void *image, uint32_t width, uint32_t height, imageFormat format)
uint32_t GetSkipFrames() const
Return the number of frames that are skipped in between classifications.
Definition: actionNet.h:184
std::string mClassPath
Definition: actionNet.h:219
float * mInputBuffers[2]
Definition: actionNet.h:203
int mLastClassification
Definition: actionNet.h:215
float GetThreshold() const
Return the confidence threshold used for classification.
Definition: actionNet.h:171
uint32_t mCurrentInputBuffer
Definition: actionNet.h:210
#define ACTIONNET_DEFAULT_INPUT
Name of default input blob for actionNet model.
Definition: actionNet.h:34
uint32_t mCurrentFrameIndex
Definition: actionNet.h:211
float mThreshold
Definition: actionNet.h:213
Action/activity classification on a sequence of images or video, using TensorRT.
Definition: actionNet.h:69
float mLastConfidence
Definition: actionNet.h:214
void SetThreshold(float threshold)
Set the confidence threshold used for classification.
Definition: actionNet.h:178
#define ACTIONNET_USAGE_STRING
Standard command-line options able to be passed to actionNet::Create()
Definition: actionNet.h:52
#define ACTIONNET_DEFAULT_OUTPUT
Name of default output confidence values for actionNet model.
Definition: actionNet.h:40
@ DEVICE_GPU
GPU (if multiple GPUs are present, a specific GPU can be selected with cudaSetDevice()
Definition: tensorNet.h:131
virtual ~actionNet()
Destroy.
uint32_t mNumClasses
Definition: actionNet.h:205
deviceType
Enumeration for indicating the desired device that the network should run on, if available in hardwar...
Definition: tensorNet.h:129
const char * GetClassDesc(int index) const
Retrieve the description of a particular class.
Definition: actionNet.h:161
static const char * Usage()
Usage string for command line arguments to Create()
Definition: actionNet.h:109
@ TYPE_FASTEST
The fastest detected precision should be use (i.e.
Definition: tensorNet.h:105
uint32_t mNumFrames
Definition: actionNet.h:206
bool init(const char *model_path, const char *class_path, const char *input, const char *output, uint32_t maxBatchSize, precisionType precision, deviceType device, bool allowGPUFallback)
const char * GetClassPath() const
Retrieve the path to the file containing the class descriptions.
Definition: actionNet.h:166
precisionType
Enumeration for indicating the desired precision that the network should run in, if available in hard...
Definition: tensorNet.h:102
int Classify(T *image, uint32_t width, uint32_t height, float *confidence=NULL)
Append an image to the sequence and classify the action, returning the index of the top class.
Definition: actionNet.h:130
static actionNet * Create(const char *network="resnet-18", uint32_t maxBatchSize=DEFAULT_MAX_BATCH_SIZE, precisionType precision=TYPE_FASTEST, deviceType device=DEVICE_GPU, bool allowGPUFallback=true)
Load a pre-trained model, either "resnet-18" or "resnet-34".
uint32_t mFramesSkipped
Definition: actionNet.h:208
Abstract class for loading a tensor network with TensorRT.
Definition: tensorNet.h:218
std::vector< std::string > mClassDesc
Definition: actionNet.h:217
#define DEFAULT_MAX_BATCH_SIZE
Default maximum batch size.
Definition: tensorNet.h:88
uint32_t GetNumClasses() const
Retrieve the number of image recognition classes.
Definition: actionNet.h:151
Command line parser for extracting flags, values, and strings.
Definition: commandLine.h:35
void SetSkipFrames(uint32_t frames)
Set the number of frames that are skipped in between classifications.
Definition: actionNet.h:195
uint32_t mSkipFrames
Definition: actionNet.h:207