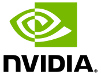 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __NETWORK_SOCKET_H_
24 #define __NETWORK_SOCKET_H_
48 #define IP_ANY 0x00000000
49 #define IP_BROADCAST 0xFFFFFFFF
50 #define IP_LOOPBACK 0x7F000001
80 bool Accept( uint64_t timeout=0 );
88 bool Bind(
const char* localIP, uint16_t port );
97 bool Bind( uint32_t localIP, uint16_t port );
104 bool Bind( uint16_t port=0 );
110 bool Connect(
const char* remoteIP, uint16_t port );
116 bool Connect( uint32_t remoteIP, uint16_t port );
127 size_t Recieve( uint8_t* buffer,
size_t size, uint32_t* remoteIP=NULL, uint16_t* remotePort=NULL );
135 size_t Recieve( uint8_t* buffer,
size_t size, uint32_t* remoteIP, uint16_t* remotePort, uint32_t* localIP );
140 bool Send(
void* buffer,
size_t size, uint32_t remoteIP, uint16_t remotePort );
160 inline int GetFD()
const {
return mSock; }
186 bool mPktInfoEnabled;
187 bool mBroadcastEnabled;
193 uint16_t mRemotePort;
size_t Recieve(uint8_t *buffer, size_t size, uint32_t *remoteIP=NULL, uint16_t *remotePort=NULL)
Wait for a packet to be recieved and dump it into the user-supplied buffer.
SocketType GetType() const
GetType.
Definition: Socket.h:165
bool Bind(const char *localIP, uint16_t port)
Bind the socket to a local host IP address and port.
bool Connect(const char *remoteIP, uint16_t port)
Connect to a listening server (TCP only).
static Socket * Create(SocketType type)
Create.
void PrintIP() const
PrintIP.
bool SetBufferSize(size_t size)
Set the rx/tx buffer sizes.
int GetFD() const
Retrieve the socket's file descriptor.
Definition: Socket.h:160
size_t GetMTU()
Retrieve the MTU (in bytes).
bool Send(void *buffer, size_t size, uint32_t remoteIP, uint16_t remotePort)
Send message to remote host.
The Socket class provides TCP or UDP ethernet networking.
Definition: Socket.h:63
@ SOCKET_TCP
flag indicating TCP virtual circuit service (SOCK_STREAM)
Definition: Socket.h:44
SocketType
TCP/UDP enumeration.
Definition: Socket.h:34
bool EnableJumboBuffer()
Enable jumbo buffer.
@ SOCKET_UDP
flag indicating UDP datagram service (SOCK_DGRAM)
Definition: Socket.h:39
bool SetRecieveTimeout(uint64_t timeout)
Set Receive() timeout (in microseconds).
bool Accept(uint64_t timeout=0)
Accept incoming connections (TCP only).