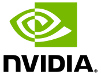 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __MULTITHREAD_RINGBUFFER_H_
24 #define __MULTITHREAD_RINGBUFFER_H_
70 inline bool Alloc( uint32_t numBuffers,
size_t size, uint32_t flags=0 );
80 inline void*
Peek( uint32_t flags );
85 inline void*
Next( uint32_t flags );
95 inline void SetFlags( uint32_t flags );
116 #include "RingBuffer.inl"
bool mReadOnce
Definition: RingBuffer.h:111
@ Threaded
Buffers should be thread-safe (enabled by default).
Definition: RingBuffer.h:46
uint32_t mNumBuffers
Definition: RingBuffer.h:104
RingBuffer(uint32_t flags=Threaded)
Construct a new ring buffer.
@ ReadOnce
Read the next buffer, but only if it hasn't been read before.
Definition: RingBuffer.h:42
size_t mBufferSize
Definition: RingBuffer.h:110
void * Next(uint32_t flags)
Get the next read/write buffer and advance the position in the queue.
A lightweight mutual exclusion lock.
Definition: Mutex.h:35
void ** mBuffers
Definition: RingBuffer.h:109
uint32_t GetFlags() const
Get the flags of the ring buffer.
@ ReadLatest
Read the latest buffer in the queue, skipping other buffers that may not have been read.
Definition: RingBuffer.h:43
void SetFlags(uint32_t flags)
Set the ring buffer's flags.
@ Write
Write the next buffer.
Definition: RingBuffer.h:45
bool Alloc(uint32_t numBuffers, size_t size, uint32_t flags=0)
Allocate memory for a set of buffers, where each buffer has the specified size.
Mutex mMutex
Definition: RingBuffer.h:112
Flags
Ring buffer flags.
Definition: RingBuffer.h:39
void SetThreaded(bool threaded)
Enable or disable multi-threading.
void Free()
Free the buffer allocations.
@ Read
Read the next buffer.
Definition: RingBuffer.h:41
Thread-safe circular ring buffer queue.
Definition: RingBuffer.h:33
@ ZeroCopy
Buffers should be allocated in mapped CPU/GPU zeroCopy memory (otherwise GPU only)
Definition: RingBuffer.h:47
uint32_t mLatestWrite
Definition: RingBuffer.h:106
@ ReadLatestOnce
Combination of ReadOnce and ReadLatest flags.
Definition: RingBuffer.h:44
uint32_t mLatestRead
Definition: RingBuffer.h:105
uint32_t mFlags
Definition: RingBuffer.h:107
void * Peek(uint32_t flags)
Get the next read/write buffer without advancing the position in the queue.