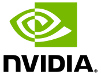 |
Jetson Inference
DNN Vision Library
|
Go to the documentation of this file.
23 #ifndef __NETWORKING_H_
24 #define __NETWORKING_H_
51 std::string
getHostByName(
const char* name, uint32_t retries=10 );
70 uint32_t
getHostByName(
const char* name,
void* ipAddress, uint32_t size, uint32_t retries=10 );
123 #define LOG_NETWORK "[network] "
struct NetworkInterface::IPv6 ipv6
std::vector< NetworkInterface > getNetworkInterfaces()
Retrieve info about the different IPv4/IPv6 network interfaces of the system.
Definition: Networking.h:83
std::string prefix
IPv6 prefix.
Definition: Networking.h:93
bool up
true if the interface is up, false if down
Definition: Networking.h:81
std::string getHostByName(const char *name, uint32_t retries=10)
Resolve the IP address of a given hostname or domain using DNS lookup, and return it as a string.
void printNetworkInterfaces(const std::vector< NetworkInterface > &interfaces)
Print out a list of network interfaces.
int findNetworkInterface(const std::vector< NetworkInterface > &interfaces, const char *name)
Find the index of a network interface by name from the list of interfaces.
std::string broadcast
IPv4 broadcast.
Definition: Networking.h:87
std::string getHostname()
Retrieve the host system's network hostname.
std::string netmask
IPv4 netmask.
Definition: Networking.h:86
std::string address
IPv4 address.
Definition: Networking.h:85
Info about a particular network interface.
Definition: Networking.h:78
Definition: Networking.h:90
struct NetworkInterface::IPv4 ipv4
std::string name
name of the network interface (e.g.
Definition: Networking.h:80
std::string address
IPv6 address.
Definition: Networking.h:92