Preview
You can add a preview of the selected area.
$('#image-1').rcrop({
minSize : [200,200],
preserveAspectRatio : true,
preview : {
display: true,
size : [100,100],
}
});
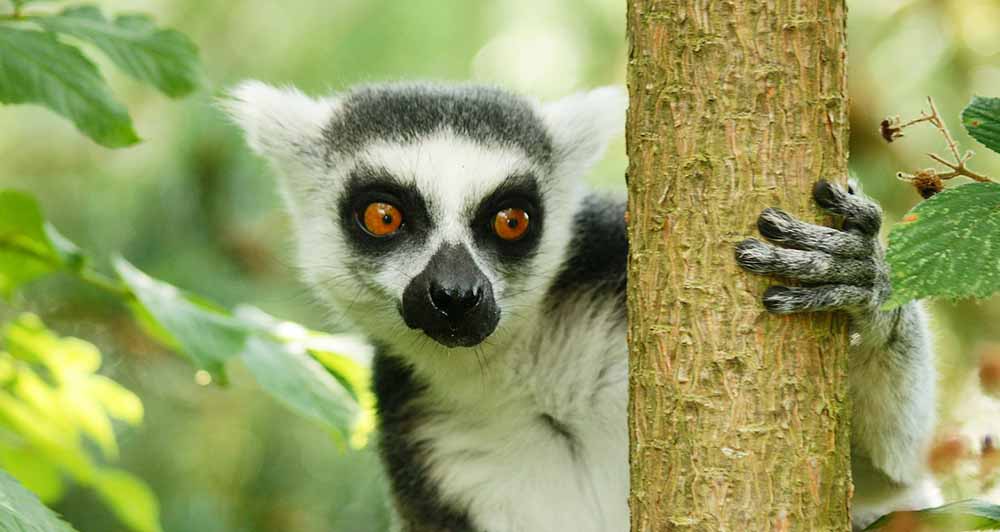
Events and methods
Here you can see an example of using 'rcrop-changed' and 'rcrop-ready' events. Also, 'getValues' and 'resize' methods are used.
Fill 'x' or 'y' fields with 'center' to center crop area, or use numbers.
var $image2 = $('#image-2'),
$update = $('#update'),
inputs = {
x : $('#x'),
y : $('#y'),
width : $('#width'),
height : $('#height')
},
fill = function(){
var values = $image2.rcrop('getValues');
for(var coord in inputs){
inputs[coord].val(values[coord]);
}
};
// Initilize
$image2.rcrop();
// Fill inputs when Responsive Cropper is ready and when crop area is being resized or dragged
$image2.on('rcrop-changed rcrop-ready', fill);
// Call resize method when button is clicked. And then fill inputs to fix invalid values.
$update.click(function(){
$image2.rcrop('resize', inputs.width.val(), inputs.height.val(), inputs.x.val(), inputs.y.val());
fill();
});
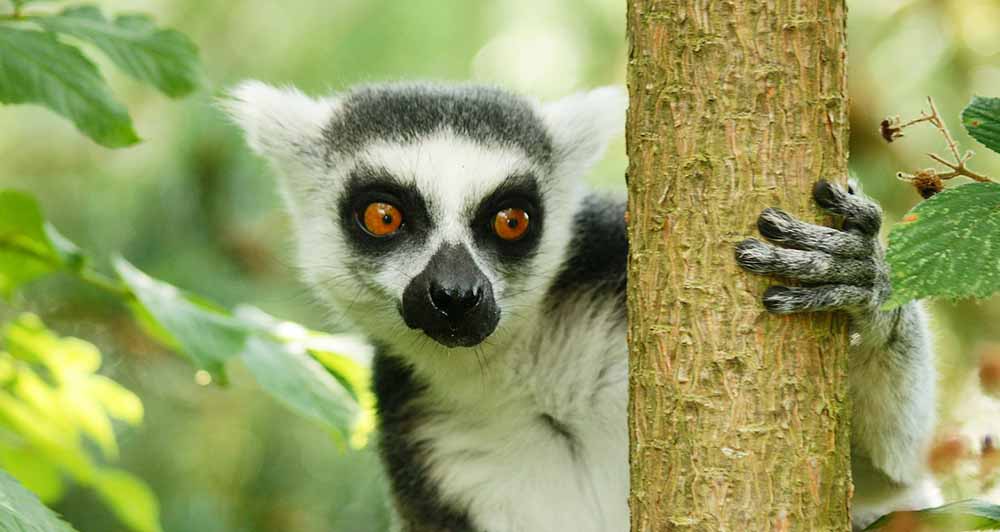
Events and methods (2)
You can add a listener to 'rcrop-changed' event. Also, you can get DataURL of the selected area
$('#image-3').rcrop({
minSize : [200,200],
preserveAspectRatio : true,
preview : {
display: true,
size : [100,100],
wrapper : '#custom-preview-wrapper'
}
});
$('#image-3').on('rcrop-changed', function(){
var srcOriginal = $(this).rcrop('getDataURL');
var srcResized = $(this).rcrop('getDataURL', 50,50);
$('#cropped-original').append('<img src="'+srcOriginal+'">');
$('#cropped-resized').append('<img src="'+srcResized+'">');
})

Images resized (50x50)
Images not resized (original size)
Styling
Style Responsive Cropper overriding CSS or editing SCSS file.
#image-4-wrapper .rcrop-outer-wrapper{
opacity: .75;
}
#image-4-wrapper .rcrop-outer{
background: #000
}
#image-4-wrapper .rcrop-croparea-inner{
border: 1px dashed #fff;
}
#image-4-wrapper .rcrop-handler-corner{
width:12px;
height: 12px;
background: none;
border : 0 solid #51aeff;
}
#image-4-wrapper .rcrop-handler-top-left{
border-top-width: 4px;
border-left-width: 4px;
top:-2px;
left:-2px
}
#image-4-wrapper .rcrop-handler-top-right{
border-top-width: 4px;
border-right-width: 4px;
top:-2px;
right:-2px
}
#image-4-wrapper .rcrop-handler-bottom-right{
border-bottom-width: 4px;
border-right-width: 4px;
bottom:-2px;
right:-2px
}
#image-4-wrapper .rcrop-handler-bottom-left{
border-bottom-width: 4px;
border-left-width: 4px;
bottom:-2px;
left:-2px
}
#image-4-wrapper .rcrop-handler-border{
display: none;
}
// For touch devices we must use clayfy handler
#image-4-wrapper .clayfy-touch-device.clayfy-handler{
background: none;
border : 0 solid #51aeff;
border-bottom-width: 6px;
border-right-width: 6px;
}
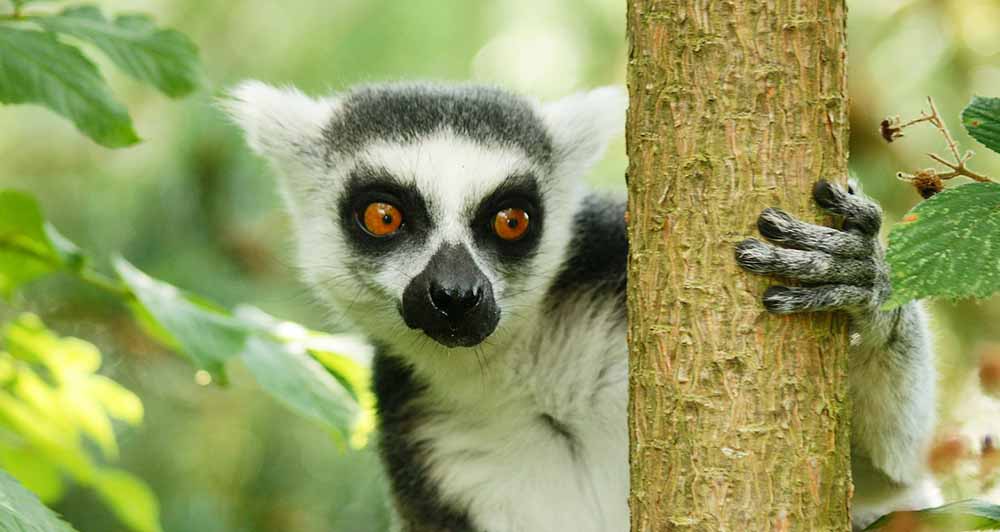