Supported Controllers
In this article, we will examine what types of controllers are supported by MonoGame.
We will also create a function to inspect a connected controller's properties.
Let's get started.
What is a Gamepad?
A Gamepad is a controller. In MonoGame controllers are referred to as gamepads.
Because MonoGame was built upon XNA, MonoGame supports Xbox360 controllers by default.
MonoGame also supports other types of controllers, but there is no official list of supported controllers.
However, you can check to see if MonoGame supports your controller through code.
Below we create a function called InspectGamePad().
Add this function to InputHelper.cs.
The above function InspectGamePad() uses MonoGame's GamePad.GetCapabilities() method.
This collects all the available information about an attached controller.
Then we report on what kind of capabilities the controller has.
You could use this in your game to make sure an attached controller has the buttons your game requires.
Perhaps your game only requires the A button and a joystick?
Using the above method you can check for an A button and joystick, or alert the user their controller is unsupported.
If MonoGame doesn't recognize your controller, there's not much you can do.
This is likely due to the computer missing the controller's drivers.
The best thing you can do as a developer is to alert the player that their controller is unsupported.
Using InspectGamePad()
Now that we've added a function to inspect an attached gamepad, let's update Game1.cs to check our controller.
Add the code below to Game1.Update(), near the end of the method.
Note that we are passing a value of 1 to the InspectGamePad() method.
This means InspectGamePad() is checking Player1's controller, but you may have multiple controllers attached.
Simply change the passed value to 1, 2, 3, or 4 to check different Player's controllers.
Run your game by pressing F5, then press the Enter key.
You should see output similar to the image below.
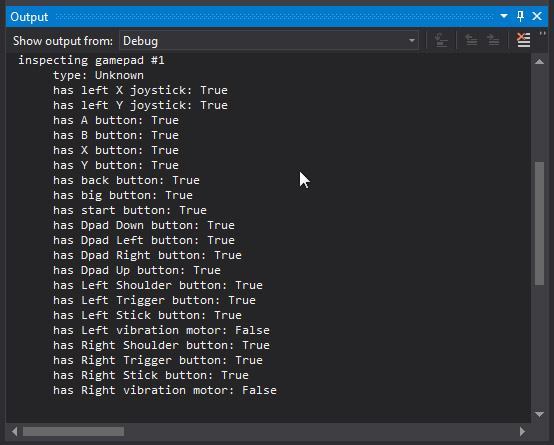
If your controller is unsupported your output will probably look like this.

If the controller is unsupported, the best thing you can do is alert the player.
Summary
You created a method in InputHelper to inspect a controller's capabilities.
You then added code to Game1.cs to inspect an attached controller.
Finally, you learned about how to inspect different attached controllers.